iOS: Image Carousel Push Notifications
How to implement an image carousel in OneSignal iOS push notifications using Swift.
iOS provides a UNNotificationContentExtension protocol as the entry point for a notification content app extension. This can be used to display a custom interface for your app’s notifications. This example guide explains how to use this for creating a carousel within an iOS notification.
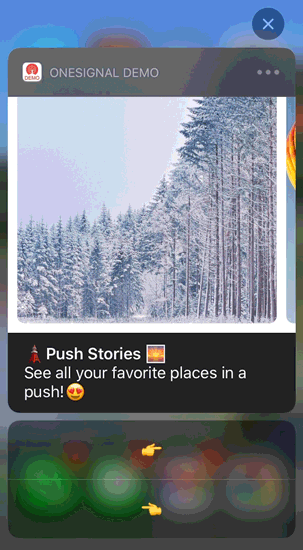
Image showing a carousel in a push notification
1. Add a Notification Content Extension
In Xcode, select File > New > Target...
Select the Notification Content Extension
Name it OSNotificationContentExtension
Select Activate to debug the new scheme.
2. Add Code to your App
Download the OSNotificationContentExtension from Github and replace the OSNotificationContentExtension
in your Xcode Project with the same file from Github.
You should see the following files added:
3. Set Your Notification Category
This example Declares The Actionable Notification Type within the AppDelegate.swift didFinishLaunchingWithOptions
.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
//START Authorize OS Notification Carousel Category
if #available(iOS 10.0, *) {
let options: UNAuthorizationOptions = [.alert]
UNUserNotificationCenter.current().requestAuthorization(options: options) { (authorized, error) in
if authorized {
let categoryIdentifier = "OSNotificationCarousel"
let carouselNext = UNNotificationAction(identifier: "OSNotificationCarousel.next", title: "👉", options: [])
let carouselPrevious = UNNotificationAction(identifier: "OSNotificationCarousel.previous", title: "👈", options: [])
let carouselCategory = UNNotificationCategory(identifier: categoryIdentifier, actions: [carouselNext, carouselPrevious], intentIdentifiers: [], options: [])
UNUserNotificationCenter.current().setNotificationCategories([carouselCategory])
}
}
}
//END Authorize OS Notification Carousel Category
return true
}
4. Send Your Push Notification
When Sending Push Messages you can set the iOS Category and custom Data.
iOS Category
Use OSNotificationCarousel
as the iOS Category:
-
API: Set with the
ios_category
API Parameter. -
Dashboard: Set under "Platform Settings" > Send to Apple iOS > "Category"
Custom Data
OneSignal doesn't have an option to upload multiple images per notification.
Instead you must list the Image URLs separated by a comma ,
- API: Use the
data
API Parameter like this:
data: {
"images" : "https://cdn.pixabay.com/photo/2015/12/01/20/28/road-1072823_960_720.jpg,https://cdn.pixabay.com/photo/2013/11/28/10/36/road-220058_960_720.jpg,https://cdn.pixabay.com/photo/2012/08/27/14/19/mountains-55067_960_720.png,https://cdn.pixabay.com/photo/2015/01/28/23/35/landscape-615429_960_720.jpg,https://cdn.pixabay.com/photo/2016/05/05/02/37/sunset-1373171_960_720.jpg"
}
- Dashboard: Set under "Advanced Settings" > "Additional Data"
For the "Key" set images
and the "Value" set the list of comma separated URLs without quotes.
Example, copy paste:
https://cdn.pixabay.com/photo/2015/12/01/20/28/road-1072823_960_720.jpg,https://cdn.pixabay.com/photo/2013/11/28/10/36/road-220058_960_720.jpg,https://cdn.pixabay.com/photo/2012/08/27/14/19/mountains-55067_960_720.png,https://cdn.pixabay.com/photo/2015/01/28/23/35/landscape-615429_960_720.jpg,https://cdn.pixabay.com/photo/2016/05/05/02/37/sunset-1373171_960_720.jpg
Send the Push
Once you receive the push, you will need to long press or swipe left and click "View" to expand the notification depending on the iOS version.
Further Reading
Updated 6 months ago