React Native SDK Setup
Instructions for adding the OneSignal React Native & Expo SDK to your app for iOS, Android, and derivatives like Amazon
Step 1. Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs
iOS Requirements
- iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- mac with Xcode 12+
- p8 Authentication Token or p12 Push Notification Certificate
Android Requirements
- Android 4.1+ device or emulator with "Google Play Store (Services)" installed.
- Set up your Google/Firebase keys in OneSignal.
- Project using AndroidX.
- compileSDKVersion set to 33 or higher.
Amazon & Huawei Requirements
Follow these instructions if your app is distributed on the Amazon AppStore and/or the Huawei AppGallery.
Running Example Project
For your convenience, we created an example project, based on React Native 0.63.
You can run this project to test configurations, debug, and build upon it.
git clone https://github.com/OneSignal/react-native-onesignal
cd react-native-onesignal && cd examples && cd RNOneSignal
yarn
- Running the Android example app:
react-native run-android
- Running the iOS example app:
- Open the
RNOneSignal
project in Xcode- Change the Signing Team and Bundle Identifier for both the RNOneSignal target as well as the OneSignalNotificationServiceExtension
- The Service Extension bundle id should be .OneSignalNotificationServiceExtension
- Build
Step 2. Add the OneSignal package to your app
Expo Setup
OneSignal is a native library and leverages the Google FCM and Apple APNS protocols. There are 2 options for adding OneSignal to your Expo Project:
- Try the OneSignal Expo Plugin. Recommended if you are using a Managed Expo Workflow. Follow the plugin's README for details.
- Use an Expo Bare Workflow. Follow Expo’s guide on Ejecting from the Managed Workflow.
2.1 Install the SDK using Yarn or NPM
- Yarn:
yarn add react-native-onesignal
- NPM
npm install --save react-native-onesignal
2.2 Link OneSignal (for RN versions < 0.60)
Skip if using React Native version of 0.60 or greater. Autolinking is now done automatically so skip to step 3.
React Native: react-native link react-native-onesignal
Step 3. Install for Android using Gradle (For Android apps)
Within your Android project's app/build.gradle
, validate that your compile and target SDK versions are set to version 33 or higher:
android {
compileSdkVersion 33
...
defaultConfig {
...
targetSdkVersion 33
}
}
Step 4. Install for iOS using Cocoapods (For iOS Apps)
You must use be using a Cocoapods version >= 1.12.1
4.1 Run cd ios && pod install
4.2 Add Required Capabilities
In your project's ios directory, open the <your-project>.xcworkspace
file in Xcode.
Select the root project and main app target. In Signing & Capabilities, select All and + Capability. Add "Push Notifications".
Click + Capability to add Background Modes and check Remote notifications.
4.3 Add a Notification Service Extension
The OneSignalNotificationServiceExtension
allows your application to receive rich notifications with images and/or buttons, and to report analytics about which notifications users receive.
4.3.1 In Xcode Select File
> New
> Target...
4.3.2 Select Notification Service Extension
then press Next
.
4.3.3 Enter the product name as OneSignalNotificationServiceExtension
and press Finish
. Do not press "Activate" on the dialog shown after this.
4.3.4 Press Cancel on the Activate scheme prompt.
By canceling, you are keeping Xcode debugging your app, instead of just the extension. If you activate by accident, you can always switch back to debug your app within Xcode (next to the play button).
4.3.5 In the Project Navigator, select the top-level project directory and select the OneSignalNotificationServiceExtension
target.
Ensure the Deployment Target
is set to the same value as your Main Application Target. Unless you have a specific reason not to, you should set the Deployment Target
to be iOS 10 which is the version of iOS that Apple released Rich Media for push. iOS versions under 10 will not be able to get Rich Media.
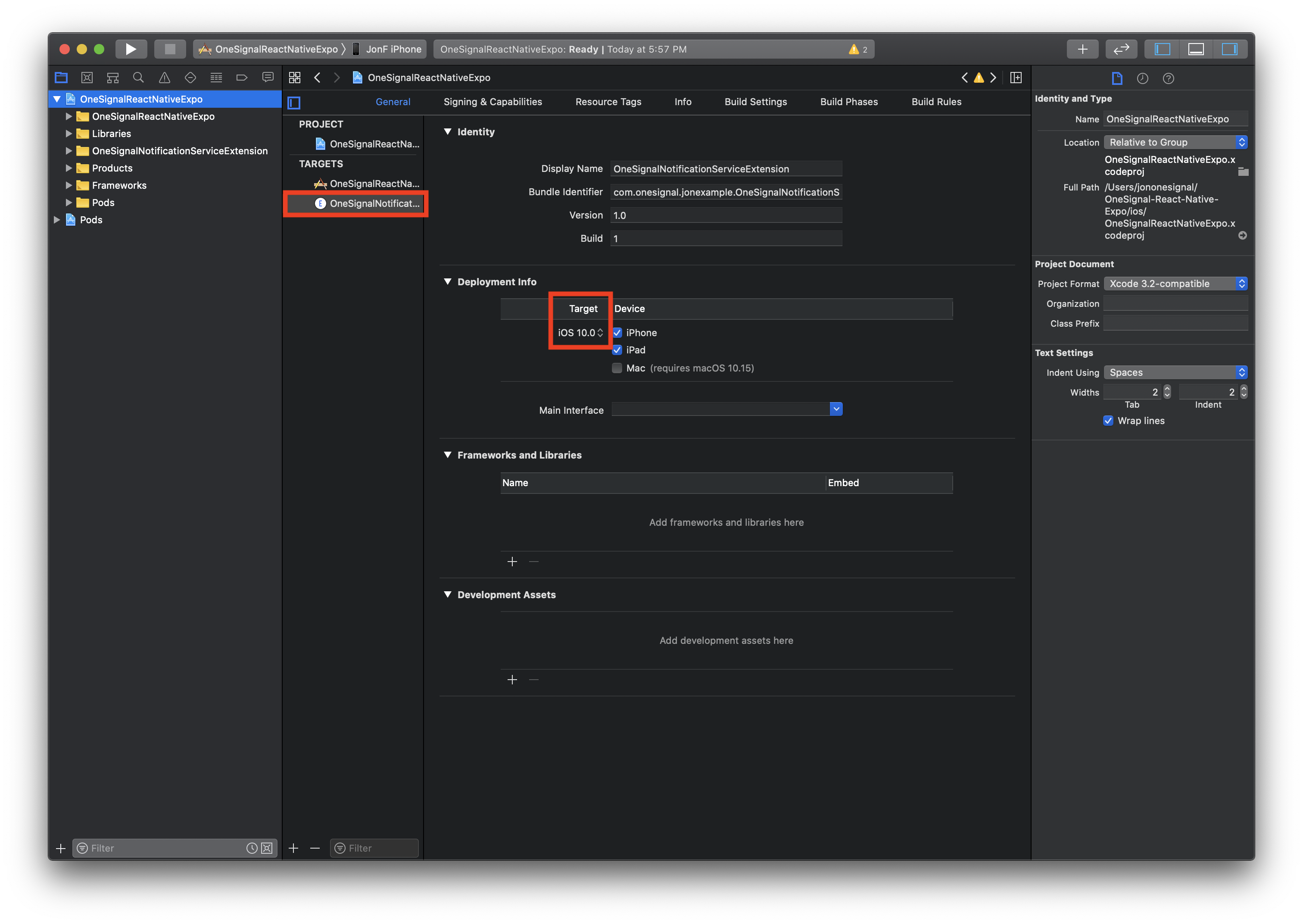
This should be the same value as your Main Application Target.
4.3.6 In your Project Root > ios > Podfile
, add the notification service extension outside the main target (should be at the same level as your main target):
target 'OneSignalNotificationServiceExtension' do
pod 'OneSignalXCFramework', '>= 3.0', '< 4.0'
end
require_relative '../node_modules/react-native/scripts/react_native_pods'
require_relative '../node_modules/@react-native-community/cli-platform-ios/native_modules'
platform :ios, '10.0'
target 'ReactNativeDemo' do
config = use_native_modules!
use_react_native!(
:path => config[:reactNativePath],
# to enable hermes on iOS, change `false` to `true` and then install pods
:hermes_enabled => false
)
target 'ReactNativeDemoTests' do
inherit! :complete
# Pods for testing
end
# Enables Flipper.
#
# Note that if you have use_frameworks! enabled, Flipper will not work and
# you should disable the next line.
use_flipper!()
post_install do |installer|
react_native_post_install(installer)
end
end
target 'OneSignalNotificationServiceExtension' do
pod 'OneSignalXCFramework', '>= 3.0', '< 4.0'
end
Close Xcode. While still in the ios
directory, run pod install
again.
Re-Open the . xcworkspace
file in Xcode. In the OneSignalNotificationServiceExtension directory > NotificationService.m
or NotificationService.swift
file, replace the whole file contents with the code below:
#import <OneSignal/OneSignal.h>
#import "NotificationService.h"
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNNotificationRequest *receivedRequest;
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@end
@implementation NotificationService
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
self.receivedRequest = request;
self.contentHandler = contentHandler;
self.bestAttemptContent = [request.content mutableCopy];
//If your SDK version is < 3.5.0 uncomment and use this code:
/*
[OneSignal didReceiveNotificationExtensionRequest:self.receivedRequest
withMutableNotificationContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
*/
/* DEBUGGING: Uncomment the 2 lines below and comment out the one above to ensure this extension is excuting
Note, this extension only runs when mutable-content is set
Setting an attachment or action buttons automatically adds this */
// NSLog(@"Running NotificationServiceExtension");
// self.bestAttemptContent.body = [@"[Modified] " stringByAppendingString:self.bestAttemptContent.body];
// Uncomment this line to set the default log level of NSE to VERBOSE so we get all logs from NSE logic
//[OneSignal setLogLevel:ONE_S_LL_VERBOSE visualLevel:ONE_S_LL_NONE];
[OneSignal didReceiveNotificationExtensionRequest:self.receivedRequest
withMutableNotificationContent:self.bestAttemptContent
withContentHandler:self.contentHandler];
}
- (void)serviceExtensionTimeWillExpire {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
[OneSignal serviceExtensionTimeWillExpireRequest:self.receivedRequest withMutableNotificationContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
}
@end
import UserNotifications
import OneSignal
class NotificationService: UNNotificationServiceExtension {
var contentHandler: ((UNNotificationContent) -> Void)?
var receivedRequest: UNNotificationRequest!
var bestAttemptContent: UNMutableNotificationContent?
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.receivedRequest = request
self.contentHandler = contentHandler
self.bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
if let bestAttemptContent = bestAttemptContent {
//If your SDK version is < 3.5.0 uncomment and use this code:
/*
OneSignal.didReceiveNotificationExtensionRequest(self.receivedRequest, with: self.bestAttemptContent)
contentHandler(bestAttemptContent)
*/
/* DEBUGGING: Uncomment the 2 lines below to check this extension is excuting
Note, this extension only runs when mutable-content is set
Setting an attachment or action buttons automatically adds this */
//OneSignal.setLogLevel(.LL_VERBOSE, visualLevel: .LL_NONE)
//bestAttemptContent.body = "[Modified] " + bestAttemptContent.body
OneSignal.didReceiveNotificationExtensionRequest(self.receivedRequest, with: bestAttemptContent, withContentHandler: self.contentHandler)
}
}
override func serviceExtensionTimeWillExpire() {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
if let contentHandler = contentHandler, let bestAttemptContent = bestAttemptContent {
OneSignal.serviceExtensionTimeWillExpireRequest(self.receivedRequest, with: self.bestAttemptContent)
contentHandler(bestAttemptContent)
}
}
}
Ignore any build errors at this point, we will resolve these later by importing the OneSignal library.
4.4 Add App Group
In order for your application to use Confirmed Deliveries and increment/decrement Badges through push notifications, you need to set up an App Group for your application.
4.4.1 In your main app target go back to Signing & Capabilities > All > + Capability and add App Groups
4.4.2 Under the newly added “App Groups” capability click the + button.
Set the “App Groups” container to be group.YOUR_BUNDLE_IDENTIFIER.onesignal
where YOUR_BUNDLE_IDENTIFIER
is the same as shown in "Bundle Identifier" then press OK.
4.4.3 Repeat this process for the OneSignalNotificationServiceExtension
Make sure the "App Groups" container is the same for both targets! Do not include OneSignalNotificationServiceExtension
. Then press OK.
If you require more details or troubleshooting help, see the iOS SDK App Groups setup guide.
Step 5. Initialize the OneSignal SDK
In your App.js
or index.js
initialize OneSignal and try the example methods below:
import OneSignal from 'react-native-onesignal';
// OneSignal Initialization
OneSignal.setAppId(ONESIGNAL_APP_ID);
// promptForPushNotificationsWithUserResponse will show the native iOS or Android notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission (See step 8)
OneSignal.promptForPushNotificationsWithUserResponse();
//Method for handling notifications received while app in foreground
OneSignal.setNotificationWillShowInForegroundHandler(notificationReceivedEvent => {
console.log("OneSignal: notification will show in foreground:", notificationReceivedEvent);
let notification = notificationReceivedEvent.getNotification();
console.log("notification: ", notification);
const data = notification.additionalData
console.log("additionalData: ", data);
// Complete with null means don't show a notification.
notificationReceivedEvent.complete(notification);
});
//Method for handling notifications opened
OneSignal.setNotificationOpenedHandler(notification => {
console.log("OneSignal: notification opened:", notification);
});
Event Listeners & Components
We suggest using a base/root component to add as an event listener. If you choose a sub-component that is only shown in some situations (such as using a homepage as an event listener), the component may unmount later on as the user navigates elsewhere in your app.
If you encounter problems with one or more of the events listeners, please see our troubleshooting documentation here.
Manually updating iOS OneSignalNativeSDK
When you install react-native-onesignal
it will automatically include a specific version of the OneSignal iOS native SDK that is known to work with it. Only follow the instructions below if there is a native OneSignal SDK fix you need that isn't included already in the latest react-native-onesignal
update.
- Download the latest OneSignal iOS native release.
- Delete
libOneSignal.a
andOneSignal.h
fromnode_modules/react-native-onesignal/ios/
- From
/iOS_SDK/OneSignalSDK/Framework/OneSignal.framework/Versions/A/
, copyOneSignal
to/node_modules/react-native-onesignal/ios/
and rename itlibOneSignal.a
- Copy
OneSignal.h
from/iOS_SDK/OneSignalSDK/Framework/OneSignal.framework/Versions/A/Headers
to/node_modules/react-native-onesignal/ios/
Step 6. Run Your App and Send Yourself a Notification
Run your app on a physical device to make sure it builds correctly. Note that the iOS Simulator does not support receiving remote push notifications.
- iOS and Android devices should be prompted to subscribe to push notifications if you used the example setup code provided.
Check your OneSignal Dashboard Audience > All Users to see your Device Record.
Then head over to Messages > New Push to Send your first Push Notification from OneSignal.
Troubleshooting
If you run into any issues please see our React Native troubleshooting guide.
Try the example project on our Github repository.
If stuck, contact support directly or email [email protected] for help.
For faster assistance, please provide:
- Your OneSignal App Id
- Details, logs, and/or screenshots of the issue.
- Steps to reproduce
Step 7. Set Custom User Properties
Recommended
After initialization, OneSignal will automatically collect common user data by default. Use the following methods to set your own custom userIds, emails, phone numbers, and other user-level properties.
Set External User Id
Required if using integrations.
Recommended for messaging across multiple channels (push, email, sms).
OneSignal creates channel-level device records under a unique Id called the player_id
. A single user can have multiple player_id
records based on how many devices, email addresses, and phone numbers they use to interact with your app.
If your app has its own login system to track users, call setExternalUserId
at any time to link all channels to a single user. For more details, see External User Ids.
let externalUserId = '123456789'; // You will supply the external user id to the OneSignal SDK
OneSignal.setExternalUserId(externalUserId);
Set Email and Phone Number
Recommended if using Email and SMS messaging.
Use the provided SDK methods to capture email and phone number when provided. Follow the channel quickstart guides for setup:
// Pass in email provided by customer
OneSignal.setEmail("[email protected]");
// Pass in phone number provided by customer
OneSignal.setSMSNumber("+11234567890");
Data Tags
Optional
All other event and user properties can be set using Data Tags. Setting this data is required for more complex segmentation and message personalization.
OneSignal.sendTag("key", "value");
Step 8. Implement a Soft-Prompt In-App Message
Optional
It is recommended that apps create an alert, modal view, or other interface that describes the types of information they want to send and gives people a clear way to opt in or out.
OneSignal provides an easy option for a "soft-prompt" using In-App Messages to meet this recommendation and have a better user experience. This also permits you to ask for permission again in the future, since the native permission prompt can no longer be shown in your app if the user clicks deny.
See our How to Prompt for Push Permissions with an In-App Message Guide for details on implementing this.
Done!
Visit Mobile Push Tutorials for next steps.
Updated 5 months ago