Web SDK Methods
OneSignal Web Push SDK Reference. Works with Chrome, Firefox, and Safari.
Legacy Player Model Docs
The methods below require the OneSignal SDK version 15.
It is recommended to upgrade to our latest version 16 SDK for User Model APIs.
See Update to User Model for migration steps.
Initialization
Loading SDK Asynchronously
Javascript flag Recommended
OneSignal recommends loading OneSignalSDK.js
with the async
flag so your page load times don't increase. To use it, place the following code before calling any other OneSignal functions.
<script src="https://cdn.onesignal.com/sdks/OneSignalSDK.js" async></script>
<script>window.OneSignal = window.OneSignal || [];</script>
Our API functions can be called asynchronously using either:
OneSignal.push(["functionName", param1, param2]);
OneSignal.push(function() { OneSignal.functionName(param1, param2); });
Option 2 must be used for functions that return a value like isPushNotificationsSupported
. Option 2 also lets you call as many OneSignal functions as you need inside the passed-in function block.
init
init
Call this from each page of your site to initialize OneSignal.
Initialization Notes
- Do not call this method twice. Doing so results in an error.
- This call is required before any other function can be used.
Init JSON options
are as follows:
Parameter | Type | Description |
---|---|---|
appId | String | Required: Your OneSignal app id found on the settings page at onesignal.com. |
subdomainName | String | Required on HTTP ONLY: This must match the label you entered in Site Settings. |
requiresUserPrivacyConsent | Boolean | Allows you to delay the initialization of the SDK until the user provides privacy consent. The SDK will not be fully initialized until the provideUserConsent function is called. |
promptOptions | JSON | Localize the HTTP popup prompt. See below details. |
welcomeNotification | JSON | Customize or disable the welcome notification sent to new site visitors. See below details. |
notifyButton | JSON | Enable and customize the notifyButton. See below details. |
persistNotification | Boolean | Chrome (non-mobile) - true : persists notification, false : disappears after some time. See our Notification Persistence Section for more info.Firefox and Safari - notifications automatically dismiss after some time and this parameter does not control that. |
webhooks | JSON | See our Webhooks page for the nested options. |
autoResubscribe | Boolean | Recommended, HTTPS ONLY - Automatically resubscribes users when they clear browser cache or migrating to OneSignal. This is set in the OneSignal dashboard during setup but if set again during initialization, will override dashboard config. |
autoRegister | Boolean | Not Recommended: Shows Native Browser Prompt immediately. We do not recommend using because it creates a poor user experience. Should be removed from code. |
notificationClickHandlerMatch | String | Default: If the launch URL of the notification matches exactly to a tab already open it will be focused."origin" - If the launch URL of the notification matches any tab already open to your domain it will be focused. See addListenerForNotificationOpened documentation for more details. |
notificationClickHandlerAction | String | "navigate" Default: Automatically navigate to the launchURL on opening the notification."focus" - Only apply if notificationClickHandlerMatch is set to "origin" . Only focuses an existing tab if the launch URL matches the domain instead of navigating. See addListenerForNotificationOpened documentation for more details. |
path | String | Special Case on HTTPS ONLY: Absolute path to OneSignal SDK web worker files. You only need to set this parameter if you do not want the files at the root of your site. |
Init promptOptions
parameters
promptOptions
parametersPass in these optional parameters within promptOptions
when initializing to localize the prompts to your custom text and language. All entries are limited in length. Foreign characters accepted. Each parameter is optional, and its default is used when it is not included.
Parameter | Type | Description |
---|---|---|
actionMessage | String | Text that says 'wants to show notifications' by default. Limited to 90 characters. |
exampleNotificationTitleDesktop | String | Text that says 'This is an example notification'. Displays on non-mobile devices. Only one line is allowed. |
exampleNotificationMessageDesktop | String | Text that says 'Notifications will appear on your desktop'. Displays on non-mobile devices. Only one line is allowed. |
exampleNotificationTitleMobile | String | Text that says 'This is an example notification'. Displays on mobile devices with limited screen width. Only one line is allowed. |
exampleNotificationMessageMobile | String | Text that says 'Notifications will appear on your device'. Displays on mobile devices with limited screen width. Only one line is allowed. |
exampleNotificationCaption | String | Text that says '(you can unsubscribe anytime)'. |
acceptButton - Slide PromptacceptButtonText - HTTP PopUp Prompt | String | Customize the "Continue" text. Limited to 15 characters. |
cancelButton - Slide PromptcancelButtonText - HTTP PopUp Prompt | String | Customize the "Cancel" text. Limited to 15 characters. |
showCredit | Boolean | Set to false to hide the OneSignal logo. |
Init welcomeNotification
parameters
welcomeNotification
parametersPass in these optional parameters within welcomeNotification
when initializing to customize or disable the welcome notification sent to new site visitors. Any person visiting your site for their first time, or an existing user who has completely cleared their cache is considered a new site visitor.
Parameter | Type | Description |
---|---|---|
disable | Boolean | Disables sending a welcome notification to new site visitors. If you want to disable welcome notifications, this is the only option you need. |
title | String | The welcome notification's title. You can localize this to your own language. If not set, or left blank, the site's title will be used. Set to one space ' ' to clear the title, although this is not recommended. |
message | String | Required: The welcome notification's message. You can localize this to your own language. A message is required. If left blank or set to blank, the default of 'Thanks for subscribing!' will be used. |
url | URL | An optional URL to open after the user clicks the welcome notification. By default, clicking the welcome notification does not open any link. This is recommended because the user has just visited your site and subscribed. |
Init notifyButton
parameters
notifyButton
parametersPass in these optional parameters within notifyButton
when initializing to enable and customize the Subscription Bell (formerly known as the notify button). All parameters below are optional. If not set, they will be replaced with their defaults.
Parameter | Type | Description |
---|---|---|
enable | Boolean | Enable the Subscription Bell. The Subscription Bell is otherwise disabled by default. |
displayPredicate | Function | A function you define that returns true to show the Subscription Bell, or false to hide it. You can also return a Promise that resolves to true or false for awaiting asynchronous operations.Typically used the hide the Subscription Bell after the user is subscribed. This function is not re-evaluated on every state change; this function is only evaluated once when the Subscription Bell begins to show. See Hiding the Subscription Bell for an example. |
size | String | One of 'small', 'medium', or 'large'. The Subscription Bell will initially appear at one of these sizes, and then shrink down to size 'small' after the user subscribes. |
position | String | Either 'bottom-left' or 'bottom-right'. The Subscription Bell will be fixed at this location on your page. |
offset | JSON | Specify CSS-valid pixel offsets using bottom, left, and right. |
prenotify | Boolean | If true, the Subscription Bell will display an icon that there is 1 unread message. When hovering over the Subscription Bell, the user will see custom text set by message.prenotify . |
showCredit | Boolean | Set false to hide the 'Powered by OneSignal' text in the Subscription Bell dialog popup. |
text | JSON | Customize the Subscription Bell text. See the example code below to know what to change. |
The following is a basic template of how you would call init()
.
// OneSignal is defined as an array here and uses push calls to support OneSignalSDK.js being loaded async.
var OneSignal = OneSignal || [];
OneSignal.push(["init", {
appId: "YOUR_APP_ID",
// Your other init settings
}]);
//Another way to initialize OneSignal
window.OneSignal = window.OneSignal || [];
OneSignal.push(function() {
OneSignal.init({
appId: "YOUR_APP_ID",
// Your other init settings
});
});
Non-HTTPS subdomainName
Parameter
subdomainName
ParameterNon-HTTPS Pages (Custom Setup)
Non-HTTPS pages require the subdomainName parameter within the label set within the OneSignal Web configuration.
The following is a basic template of a custom setup using the subDomainName parameter.
OneSignal.push(["init", {
appId: "YOUR_APP_ID",
// Your other init settings
subdomainName: "YOUR_SUBDOMAIN_NAME_HERE"
}]);
setDefaultNotificationUrl
Method
setDefaultNotificationUrl
MethodPass in the full URL of the default page you want to open when a notification is clicked. When creating a notification, any URL you specify will take precedence and override the default URL. However if no URL is specified, this default URL specified by this call will open instead. If no default URL is specified at all, the notification opens to the root of your site by default.
Safari - This function is not available. Instead, the default notification icon URL is the Site URL you set in your Safari settings.
Parameter | Type | Description |
---|---|---|
url | String | page url to open from notifications |
OneSignal.push(function() {
/* These examples are all valid */
OneSignal.setDefaultNotificationUrl("https://example.com");
OneSignal.setDefaultNotificationUrl("https://example.com/subresource/resource");
OneSignal.setDefaultNotificationUrl("http://subdomain.example.com:8080/a/b");
});
setDefaultTitle
Method
setDefaultTitle
MethodSets the default title to display on notifications. If a notification is created with a title, the specified title always overrides this default title.
A notification's title defaults to the title of the page the user last visited. If your page titles vary between pages, this inconsistency can be undesirable. Call this to standardize page titles across notifications, as long as a notification title isn't specified.
Parameter | Type | Description |
---|---|---|
title | String | String to set as a default title on notifications |
OneSignal.push(function() {
OneSignal.setDefaultTitle("My Title");
});
provideUserConsent
Method
provideUserConsent
MethodIf your website is set to require the user's privacy consent or some action before they can subscribe to push, add requiresUserPrivacyConsent: true
property in the OneSignal init call. This will stop our SDK from initializing until you call provideUserConsent(true)
.
You can also revoke consent by setting provideUserConsent(false)
. This will prevent further collection of user data. To delete the user's current data see Delete User Data.
function userTappedProvideConsentButton() {
// Will initialize the SDK and register for push notifications
OneSignal.push(function() {
OneSignal.provideUserConsent(true);
});
}
Registering Push
showNativePrompt
Method
showNativePrompt
MethodHTTP Setup
Opens a popup window to mylabel.os.tc/subscribe
to prompt the user to subscribe to push notifications. Call this in response to a user action like a button or link that was just clicked, otherwise the browser's popup blocker will prevent the popup from opening.
HTTPS Setup
You may call this at any time to show the prompt for push notifications. If notification permissions have already been granted, nothing will happen.
OneSignal.push(function() {
OneSignal.showNativePrompt();
});
OneSignal.push(function() {
OneSignal.registerForPushNotifications();
});
registerForPushNotifications
Method
registerForPushNotifications
MethodSee showNativePrompt.
permissionPromptDisplay
Event
permissionPromptDisplay
EventEvent occurs when the browser's native permission request has just been shown.
OneSignal.push(function() {
OneSignal.on('permissionPromptDisplay', function () {
console.log("The prompt displayed");
});
});
showSlidedownPrompt
Method
showSlidedownPrompt
MethodShows the OneSignal Slide Prompt for HTTP and HTTPS sites. This slides down from the top (or up from the bottom on mobile). Please see Slide Prompt for more details.
Note: This does not replace the Native Browser Prompt required for subscription.
OneSignal.push(function() {
OneSignal.showSlidedownPrompt();
});
OneSignal.push(function() {
OneSignal.showHttpPrompt();
});
Prompting behavior if declined
If declined, future calls will be ignored for 3 days with additional backoff on another decline.
Note: If you need to override this back off behavior to prompt the user again you can do so by passing {force: true}
. To provide a good user experience however ONLY do this from an action taken on your site to avoid unexpected prompts.
showCategorySlidedown
Method
showCategorySlidedown
MethodShows the OneSignal Category Slidedown for HTTP and HTTPS sites. This slides down from the top (or up from the bottom on mobile). Please see Category Slidedown for more details.
Note: This does not replace the Native Browser Prompt required for subscription.
OneSignal.push(function() {
OneSignal.showCategorySlidedown();
});
Prompting behavior if declined
(see showSlidedownPrompt)
getNotificationPermission
Event
getNotificationPermission
EventReturns a Promise that resolves to the browser's current notification permission as 'default', 'granted', or 'denied'.
You can use this to detect whether the user has allowed notifications, blocked notifications, or has not chosen either setting.
Parameter | Type | Description |
---|---|---|
callback | function | A callback function that will be called when the browser's current notification permission has been obtained, with one of 'default', 'granted', or 'denied'. |
OneSignal.push(["getNotificationPermission", function(permission) {
console.log("Site Notification Permission:", permission);
// (Output) Site Notification Permission: default
}]);
isPushNotificationsSupported
Method
isPushNotificationsSupported
MethodReturns true if the current browser environment viewing the page supports push notifications.
Almost all of the API calls on this page internally call this method first before continuing; this check is therefore optional but you may call it if you wish to display a custom message to the user.
This method is synchronous and returns immediately.
OneSignal.push(function() {
/* These examples are all valid */
var isPushSupported = OneSignal.isPushNotificationsSupported();
if (isPushSupported) {
// Push notifications are supported
} else {
// Push notifications are not supported
}
});
isPushNotificationsEnabled
Method
isPushNotificationsEnabled
MethodHTTPS Only Returns a Promise
that resolves to true
if the user has already accepted push notifications and successfully registered with Google's FCM server and OneSignal's server (i.e. the user is able to receive notifications).
If you used OneSignal.setSubscription()
or unsubscribed using the Bell Prompt or Custom Link prompt after the user successfully subscribes through the browser, isPushNotificationsEnabled
will show whatever value you set for setSubscription
.
If you're deleting your user entry on our online dashboard for testing, the SDK will not sync with our dashboard and so this method will still return true
(because you are still subscribed to the site's notifications). Follow Clearing your cache and resetting push permissions to reset the browser data.
Parameter | Type | Description |
---|---|---|
isPushNotificationsEnabledCallBack | Function | Callback to be fired when the check completes. The first parameter of the callback is a boolean value indicating whether the user can receive notifications. |
OneSignal.push(function() {
OneSignal.isPushNotificationsEnabled(function(isEnabled) {
if (isEnabled)
console.log("Push notifications are enabled!");
else
console.log("Push notifications are not enabled yet.");
});
});
// Another option
OneSignal.push(function() {
OneSignal.isPushNotificationsEnabled().then(function(isEnabled) {
if (isEnabled)
console.log("Push notifications are enabled!");
else
console.log("Push notifications are not enabled yet.");
});
});
subscriptionChange
Event
subscriptionChange
EventEvent occurs when the user's subscription state changes between unsubscribed and subscribed.
Chrome and Firefox - the user's subscription is true
when:
- Your site is granted notification permissions
- Your site visitor's web storage database containing OneSignal-related data is intact
- You have not manually opted out the user from receiving notifications
- The Subscription Bell 'Subscribe' and 'Unsubscribe' button opts users in and out without affecting their other subscription parameters
OneSignal.setSubscription()
is used to opt the user in and out
- Your site visitor has an installed background web worker used to display notifications
Safari - only the first three are required for a valid subscription.
Use this event to find out when the user has successfully subscribed. The parameter will be set to true to represent a new subscribed state.
A user is no longer subscribed if:
- The user changes notification permissions
- The icon next to the URL in the address bar can be clicked to modify site permissions
- Chrome and Firefox notifications come with a button on the notification to block site notifications
- The user clears their browser data (clearing cookies will not effect subscription)
- Another background web worker overwrites our web worker
This event is not related to OneSignal.setSubscription()
, which is used to temporarily opt-out the user from receiving notifications by setting a flag on our system so that notifications are not delivered to the user.
To detect changes when using the OneSignal.setSubscription()
flag, you would use the OneSignal.isPushNotificationsEnabled()
method but this will not get called until the page is refreshed.
Callback Event Parameters
Parameter | Type | Description |
---|---|---|
subscribed | Boolean | Set to true if the user is currently subscribed; otherwise set to false . |
OneSignal.push(function() {
// Occurs when the user's subscription changes to a new value.
OneSignal.on('subscriptionChange', function (isSubscribed) {
console.log("The user's subscription state is now:", isSubscribed);
});
// This event can be listened to via the `on()` or `once()` listener.
});
Analytics
notificationPermissionChange
Event
notificationPermissionChange
Event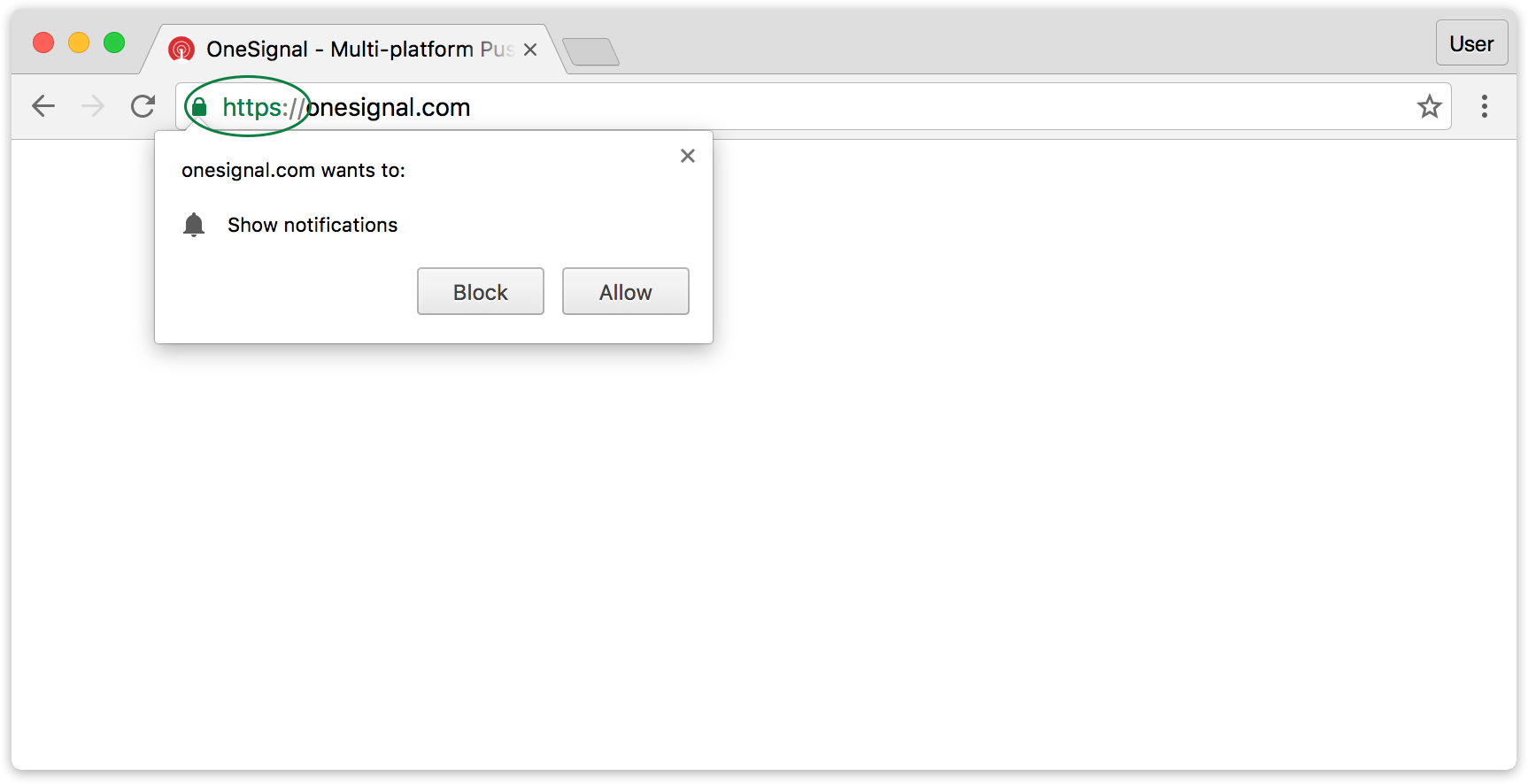
Event occurs when the user clicks Allow or Block or dismisses the browser's native permission request.
Callback Event Parameters
Parameter | Type | Description |
---|---|---|
event | Hash | A JavaScript object with a to property.If to is "granted" , the user has allowed notifications. If to is "denied" , the user has blocked notifications. If to is "default" , the user has clicked the 'X' button to dismiss the prompt. |
OneSignal.push(function() {
OneSignal.on('notificationPermissionChange', function(permissionChange) {
var currentPermission = permissionChange.to;
console.log('New permission state:', currentPermission);
});
// This event can be listened to via the on() or once() listener
});
Slide Prompt Events
popoverShown
- Slide Prompt has just animated into view and is being shown to the user.
popoverAllowClick
- The "Continue" button on the Slide Prompt was clicked.
popoverCancelClick
- The "No Thanks" button on the Slide Prompt was clicked.
popoverClosed
- The Slide Prompt was just closed.
OneSignal.push(function() {
OneSignal.on('popoverShown', function() {
console.log('Slide Prompt Shown');
});
});
customPromptClick
Event
customPromptClick
EventEvent occurs when the user clicks "No Thanks" or "Continue" on our HTTP Pop-Up Prompt (not the browser's permission request).
Our web SDK shows different permission messages and requests, and this event is best used only with the HTTP Pop-Up Prompt.
Callback Event Parameters
Parameter | Type | Description |
---|---|---|
event | Hash | A JavaScript object with a result property.- If result is denied , the user clicked No Thanks on the HTTP Pop-Up Prompt. Popup window closes after this.- If result is granted , the user clicked Continue on the HTTP Pop-Up Prompt. |
Note: If you are using the Slide Prompt, you will always see this event fire with granted
, since our SDK will automatically click Continue on the popup window for the user. You should therefore ignore this event if you are using the Slide Prompt.
OneSignal.push(function() {
OneSignal.on('customPromptClick', function(promptClickResult) {
var promptClickResult = permissionChange.result;
console.log('HTTP Pop-Up Prompt click result:', promptClickResult);
});
// This event can be listened to via the on() or once() listener
});
User IDs
getUserId
Method
getUserId
MethodReturns a Promise
that resolves to the stored OneSignal subscription ID of the Push Record if one is set, otherwise the Promise resolves to null
. If the user isn't already subscribed, this function will resolve to null
immediately.
If you're getting the subscription ID after the user subscribes, call this within the subscriptionChange
event, and check that subscriptionChange
is true
.
If you're getting the subscription ID on page load, check that the user is subscribed to notifications (e.g. isPushNotificationsEnabled()
).
For custom implementations involving our REST API, associate this OneSignal subscription ID with your data.
Once created, the subscription ID will not change. If the user unsubscribes from web push, for example by clearing their browser data, and resubscribes, a new subscription ID will be created and a new entry will be stored on your app's users list. The old entry will be unsubscribed and not be automatically deleted.
Parameter | Type | Description |
---|---|---|
callback | function | A callback function which sets the first parameter to the stored Push Record's OneSignal subscription ID if one is set, otherwise the first parameter is set to null. |
OneSignal.push(function() {
/* These examples are all valid */
OneSignal.getUserId(function(userId) {
console.log("OneSignal User ID:", userId);
// (Output) OneSignal User ID: 270a35cd-4dda-4b3f-b04e-41d7463a2316
});
});
//Another example:
OneSignal.push(function() {
OneSignal.getUserId().then(function(userId) {
console.log("OneSignal User ID:", userId);
// (Output) OneSignal User ID: 270a35cd-4dda-4b3f-b04e-41d7463a2316
});
});
External User Ids
OneSignal creates and stores device & channel level data under a unique OneSignal Id called the subscription_id
. A single user can have multiple subscription_id
records based on how many devices, email addresses, and phone numbers they use to interact with your app/site.
You can combine subscription_id
records in OneSignal under a unique User Id called the external_id
.
See the External User Ids guide for more details.
If you have a backend server, we strongly recommend using Identity Verification with your users. Your backend can generate an identifier authentication token and send it to your site.
Tags
Data Tags are custom key : value
pairs of string or number data you set on users based on events or user data of your choosing. See Data Tags Overview for more details on what tags are used for.
See Data Tag Implementation for SDK Method details.
Push Notifications
Create Notification
To send notifications to users, we recommend using the Create notification REST API docs, or Messages Dashboard because the Web Push API is run client-side, it does not have the ability to programmatically send to multiple users at once, or users that are not currently on your website.
sendSelfNotification
Method
sendSelfNotification
MethodSends to individual users only
This does not send to all users. Please see our Create notification REST API for sending notifications. This function is primarily for your own internal testing.
Sends a push notification to the current user on the webpage. This is a simplified utility function to send a test message to yourself or a quick message to the user. It does not support any targeting options.
Parameter | Type | Description |
---|---|---|
title | String | The notification's title. Currently defaults to "OneSignal Test Message" if not set. Use a " " single space to get around this. Multiple languages are not supported; write the text in the language the current user should receive it in. |
message | String | The notification's body content. Required. Multiple languages are not supported; write the text in the language the current user should receive it in. |
url | String | The URL to launch when the notification is clicked. Use a special URL to prevent any URL from launching. Defaults to this special URL if none is set. |
icon | String | The URL to use for the notification icon. |
data | Hash | Additional data to pass for the notification. |
buttons | Array of Hash | See Action Buttons. |
OneSignal.sendSelfNotification(
/* Title (defaults if unset) */
"OneSignal Web Push Notification",
/* Message (defaults if unset) */
"Action buttons increase the ways your users can interact with your notification.",
/* URL (defaults if unset) */
'https://example.com/?_osp=do_not_open',
/* Icon */
'https://onesignal.com/images/notification_logo.png',
{
/* Additional data hash */
notificationType: 'news-feature'
},
[{ /* Buttons */
/* Choose any unique identifier for your button. The ID of the clicked button is passed to you so you can identify which button is clicked */
id: 'like-button',
/* The text the button should display. Supports emojis. */
text: 'Like',
/* A valid publicly reachable URL to an icon. Keep this small because it's downloaded on each notification display. */
icon: 'http://i.imgur.com/N8SN8ZS.png',
/* The URL to open when this action button is clicked. See the sections below for special URLs that prevent opening any window. */
url: 'https://example.com/?_osp=do_not_open'
},
{
id: 'read-more-button',
text: 'Read more',
icon: 'http://i.imgur.com/MIxJp1L.png',
url: 'https://example.com/?_osp=do_not_open'
}]
);
setSubscription
Method
setSubscription
MethodThis will not subscribe users that have not opted in to notifications already
Only available if user opted-in to notifications through native prompt. Meant for a profile settings option and not to subscribe users automatically.
Do not use with Subscription Button
This function is not for use with sites that implement the Subscription Bell, as this function may override user preferences.
This function is for sites that wish to have more granular control of which users receive notifications, such as when implementing notification preference pages.
This function lets a site mute or unmute notifications for the current user. This event is not related to actually prompting the user to subscribe. The user must already be subscribed for this function to have any effect.
Set to false
to temporarily "mute" notifications from going to the user. If you previously set this to false, you can set it to true
to "un-mute" notifications so that the user receives them again.
Parameter | Type | Description |
---|---|---|
unmute | Boolean | true un-mutes any subscribed userfalse mutes any subscribed user |
Returns a promise that resolves after temporarily opting the user out of receiving notifications by setting a flag on our system so that notifications are not delivered to the user.
// Either option works:
OneSignal.setSubscription(false);
//or
OneSignal.push(["setSubscription", false]);
Receiving Notifications
notificationDisplay
Event
notificationDisplay
EventNote: Not supported on Safari and requires HTTPS website.
Event occurs after a notification is visibly displayed on the user's screen.
This event is fired on your page. If multiple browser tabs are open to your site, this event will be fired on all pages on which OneSignal is active.
Callback Event Parameters
event
is a JavaScript object hash containing:
Parameter | Type | Description |
---|---|---|
id | UUID | The OneSignal notification ID of the notification that was just displayed. |
heading | String | The notification's title. |
content | String | The notification's body message, not including the title. |
data | Hash | Custom additional data you send along with the notification. If you did not send any data, this field will not exist and be undefined . |
url | String | The URL that will be opened if the user clicks on the notification. |
icon | String | The URL to the icon used for the notification. This is fetched every time the notification is displayed. |
Notification Display Event Limitations
The
notificationDisplay
event is only emitted on sites using HTTPS and not available for Safari. Depending on the notification, more fields (e.g. action buttons) can be included in the callback parameter.
OneSignal.push(function() {
OneSignal.on('notificationDisplay', function(event) {
console.warn('OneSignal notification displayed:', event);
});
//This event can be listened to via the `on()` or `once()` listener
});
notificationDismiss
Event
notificationDismiss
EventNote: Not supported on Safari and requires HTTPS website.
This event occurs when:
- A user purposely dismisses the notification without clicking the notification body or action buttons
- On Chrome on Android, a user dismisses all web push notifications (this event will be fired for each web push notification we show)
- A notification expires on its own and disappears
Note: This event does not occur if a user clicks on the notification body or one of the action buttons. That is considered a notification click (please see the addListenerForNotificationOpened
method).
This event is fired on your page. If multiple browser tabs are open to your site, this event will be fired on all pages on which OneSignal is active.
Callback Event Parameters
event
is a JavaScript object hash containing:
Parameter | Type | Description |
---|---|---|
id | UUID | The OneSignal notification ID of the notification that was just displayed. |
heading | String | The notification's title. |
content | String | The notification's body message, not including the title. |
data | Hash | Custom additional data you send along with the notification. If you did not send any data, this field will not exist and be undefined . |
url | String | The URL that will be opened if the user clicks on the notification. |
icon | String | The URL to the icon used for the notification. This is fetched every time the notification is displayed. |
Note: Depending on the notification, more fields (e.g. action buttons) can be included in the callback parameter.
OneSignal.push(function() {
OneSignal.on('notificationDismiss', function(event) {
console.warn('OneSignal notification dismissed:', event);
});
//This event can be listened to via the `on()` or `once()` listener
});
addListenerForNotificationOpened
Event
addListenerForNotificationOpened
EventNote: Not supported on Safari.
Note: This event occurs once only. If you would this to occur continuously every time a notification is clicked, please call this method again after your callback fires.
Use this function to:
- Listen for future clicked notifications
- Check for notifications clicked in the last 5 minutes
Your callback will execute when the notification's body/title or action buttons are clicked.
The spec below describes:
- The default behavior
Events only fire if your URL matches the notification's opening URL exactly; the event will be available on the newly opened tab (and only that opened tab) to the notification's opening URL. See the special flagsnotificationClickHandlerMatch: origin
andnotificationClickHandlerAction: focus
for different use cases. - A special init flag
notificationClickHandlerMatch: 'origin'
If you have an existing tab of your site open, and the clicked notification is also to your site, the existing tab will be used. - A special init flag
notificationClickHandlerAction: 'focus'
Instead of navigating the target tab to the notification's URL, the tab will be focused. You can perform navigation manually or choose not to navigate.
These two optional flags must be placed in the root of your OneSignal initialization options. They will be stored (or removed if missing) on each page load as OneSignal parses the available init options.
HTTPS Sites
-
Default Behavior (no special flags)
- If no existing site tabs are open, a new tab is opened. You may call
addListenerForNotificationOpened()
to get the details of the notification you just clicked. - If one or more tabs to your site is open:
- And the clicked notification shares the same URL as one of your site's tab, the existing tab is focused. Call
addListenerForNotificationOpened()
to get the clicked notification's details, or if you installed a callback listener your callback will be invoked. - And the clicked notification's URL is different, a new tab is opened. You may call
addListenerForNotificationOpened()
to get the details of the notification you just clicked.
- And the clicked notification shares the same URL as one of your site's tab, the existing tab is focused. Call
- If no existing site tabs are open, a new tab is opened. You may call
-
Using init option
notificationClickHandlerMatch: 'origin'
- If no existing site tabs are open, the behavior is the same as the default (see above).
- If one or more tabs to your site is open:
- And the clicked notification shares the same URL as one of your site's tab, the behavior is the same as the default (see above).
- And the clicked notification's URL is different, the most recently opened tab of your site is focused and navigated to the notification's URL. Call
addListenerForNotificationOpened()
on the newly navigated tab to get the clicked notification's details.
-
Using init option
notificationClickHandlerAction: 'focus'
- If no existing site tabs are open, the behavior is the same as the default (see above).
- Any other case: The most recently opened tab of your site is focused only (not navigated away). Call
addListenerForNotificationOpened()
to get the clicked notification's details, or if you installed a callback listener your callback will be invoked.
Does not work when using sendSelfNotification()
testing method.
HTTP Integrations
Mostly identical to HTTPS sites above, with the following exceptions:
Due to limitations on HTTP sites:
- If multiple stale tabs are open to your site, a clicked notification sharing an identical URL to an existing tab may not focus the tab and may instead open a new tab.
- When using
notificationClickHandlerMatch: 'origin'
, if multiple stale tabs are open to your site, a different tab than the matching tab may be focused.
Parameter | Type | Description |
---|---|---|
id | String | The OneSignal notification ID of the notification that was just clicked. |
heading | String | Title set on the notification. |
content | String | The message text the user seen in the notification. |
icon | String | Icon set on the notification. |
url | String | The URL that this notification opened. |
data | Hash | Custom additional data you send along with the notification. If you did not send any data, this field will not exist and be undefined . |
action | String | Describes whether the notification body was clicked or one of the action buttons (if present) was clicked. An empty string means the notification body was clicked, otherwise the string is set to the action button ID. |
OneSignal.push(["addListenerForNotificationOpened", function(data) {
console.log("Received NotificationOpened:");
console.log(data);
}]);
/* Do NOT call init twice */
OneSignal.push(["init", {
/* Your other init settings ... */
notificationClickHandlerMatch: 'exact', /* See above documentation: 'origin' relaxes tab matching requirements, 'exact' is default */
notificationClickHandlerAction: 'navigate', /* See above documentation: 'focus' does not navigate the targeted tab, 'navigate' is default */
/* ... */
}]);
Email
OneSignal's Mobile and Web SDKs provide methods for collecting emails, logging out emails, and tracking email subscription changes.
See the Email SDK Methods guide for more details.
If you have a backend server, we strongly recommend using Identity Verification with your users. Your backend can generate an Email authentication token and send it to your app.
SMS
OneSignal's Mobile and Web SDKs provide methods for collecting phone numbers, logging out phone numbers, and tracking sms subscription changes.
See the SMS SDK Methods guide for more details.
If you have a backend server, we strongly recommend using Identity Verification with your users. Your backend can generate an SMS authentication token and send it to your app.
Updated 13 days ago