Solar2D SDK Setup
Instructions for adding the OneSignal SDK to your Solar2D app for iOS, Android, and derivatives like Amazon
Step 1. Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs.
iOS Requirements
- An iOS 9+ device (iPhone, iPad, iPod Touch) to test on. The Xcode simulator doesn't support push notifications so you must test on a real device.
- A Mac with Xcode 12+.
- An iOS Push Certificate.
Android Requirements
- An Android 4.0.3+ device or emulator with "Google Play Store (Services)" installed.
- A Google/Firebase Server API Key.
Amazon Requirements
Follow these instructions if your app is distributed on the Amazon AppStore.
Step 2. Add OneSignal to your Account
2.1 Login into your account on Solar2D's site and search for the OneSignal plugin on the Solar2D plugin directory.
2.2 Click the "FREE" button.
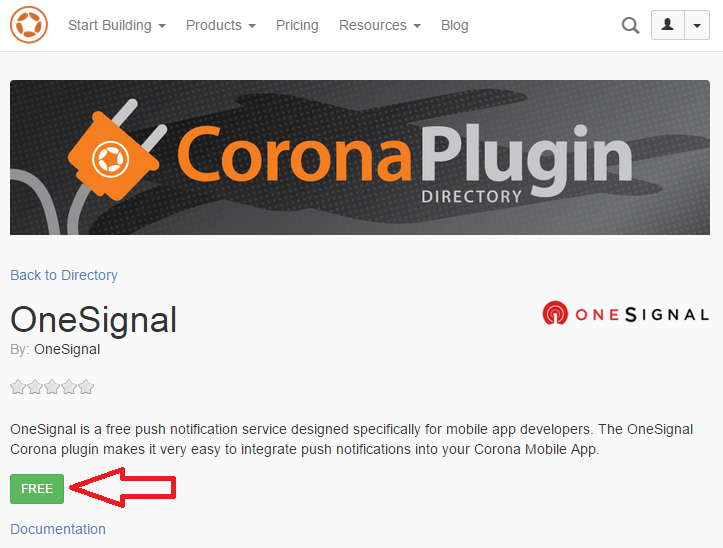
2.3 Click "Finish Checkout".
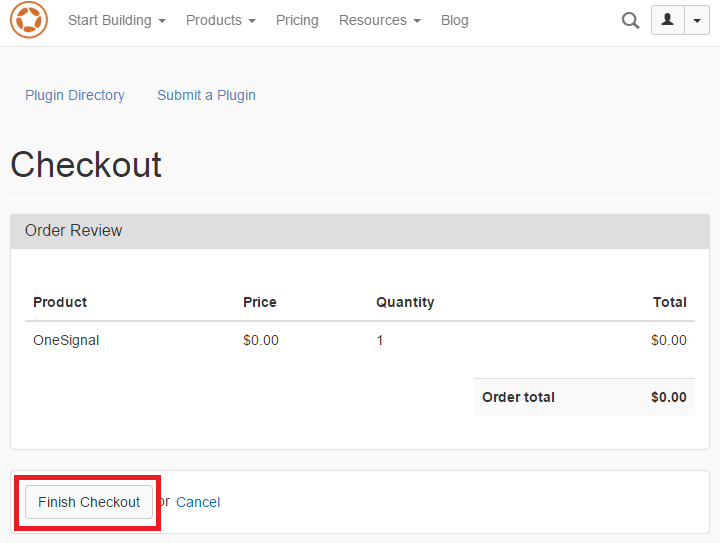
Step 3. Add OneSignal to Your Project
3.1 Update the build.settings
file in the Solar2D project folder to contain the following inside of settings = {
.
plugins =
{
["plugin.OneSignal"] =
{
publisherId = "com.onesignal",
}
}
3.2 iOS - Add the following line inside of 'plist = {' in build.settings.
UIBackgroundModes = {"remote-notification"},
Step 4. Add Required Code
4.1 At the top of main.lua
, place the following code.
-- This function gets called when the user opens a notification or one is received when the app is open and active.
-- Change the code below to fit your app's needs.
function DidReceiveRemoteNotification(message, additionalData, isActive)
if (additionalData) then
if (additionalData.discount) then
native.showAlert( "Discount!", message, { "OK" } )
-- Take user to your app store
elseif (additionalData.actionSelected) then -- Interactive notification button pressed
native.showAlert("Button Pressed!", "ButtonID:" .. additionalData.actionSelected, { "OK"} )
end
else
native.showAlert("OneSignal Message", message, { "OK" } )
end
end
local OneSignal = require("plugin.OneSignal")
-- Uncomment SetLogLevel to debug issues.
-- OneSignal.SetLogLevel(4, 4)
OneSignal.Init("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX", "############", DidReceiveRemoteNotification)
4.2 Replace XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
with your Application Key, available in the OneSignal dashboard under Settings > Keys & IDs.
4.3 Android - Replace ############
with your Google Project number.
4.4 Amazon - Put your app_key.txt
(from Generate an Amazon API Key instructions above) into the root of your Solar2D project folder.
When you build your app, change the Target App Store
setting to Amazon
.
Step 5. Run Your App and Send Yourself a Notification
Run your app on a physical device to make sure it builds correctly. Note that the iOS Simulator does not support receiving remote push notifications.
- Android devices should be subscribed to push notifications immediately upon opening the app.
- iOS devices should be prompted to subscribe to push notifications if you used the example setup code provided.
Check your OneSignal Dashboard Audience > All Users to see your Device Record.
Then head over to Messages > New Push to Send your first Push Notification from OneSignal.
Troubleshooting
If you get an error saying
"Plugin_OneSignal not found"
, make sure the plugin shows as active on your account on the Solar2D's plugin directory page.Also, make sure the same account you used on the Solar2D plugin directory is the same account you signed into in the Solar2D Simulator.
If that is correct, you may need to wait 30 minutes to 1 hour for OneSignal to become active on your account. Feel free to contact us or Solar2D if the error persists.
Try the example project on our Github repository.
If stuck, feel free to contact [email protected] for assistance.
Setup SDK for Solar2D Native
1. Add OneSignal to Your Project
1.1 Update the build.settings
file in the Solar2D project folder to contain the following inside of settings = {
.
plugins =
{
["plugin.OneSignal"] =
{
publisherId = "com.onesignal",
}
}
2. Add Required Code
2A Android - Run android/download_plugins.sh
located in your app folder. You can find more details about this step in Solar2D's blog post.
2B iOS - 1. Open your project in Xcode. 2. In the Xcode toolbar, click on the active scheme button, and select Download Plugins. You can find more details about this step in Solar2D's Blog post.
2.1 At the top of main.lua
, place the following code.
-- This function gets called when the user opens a notification or one is received when the app is open and active.
-- Change the code below to fit your app's needs.
function DidReceiveRemoteNotification(message, additionalData, isActive)
if (additionalData) then
if (additionalData.discount) then
native.showAlert( "Discount!", message, { "OK" } )
-- Take user to your app store
elseif (additionalData.actionSelected) then -- Interactive notification button pressed
native.showAlert("Button Pressed!", "ButtonID:" .. additionalData.actionSelected, { "OK"} )
end
else
native.showAlert("OneSignal Message", message, { "OK" } )
end
end
local OneSignal = require("plugin.OneSignal")
OneSignal.Init("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX", "############", DidReceiveRemoteNotification)
2.2 Replace XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
with your Application Key, which you can find in the OneSignal dashboard under Settings > Keys & IDs.
2.3 Replace ############
with your Google Project number if your app is for Android.
3. Add Plugin to iOS
3.1 Add Required Capabilities
3.1A Select the root project, and Under Capabilities, enable "Push Notifications"
3.1B Next, enable "Background Modes" and check "Remote Notifications"
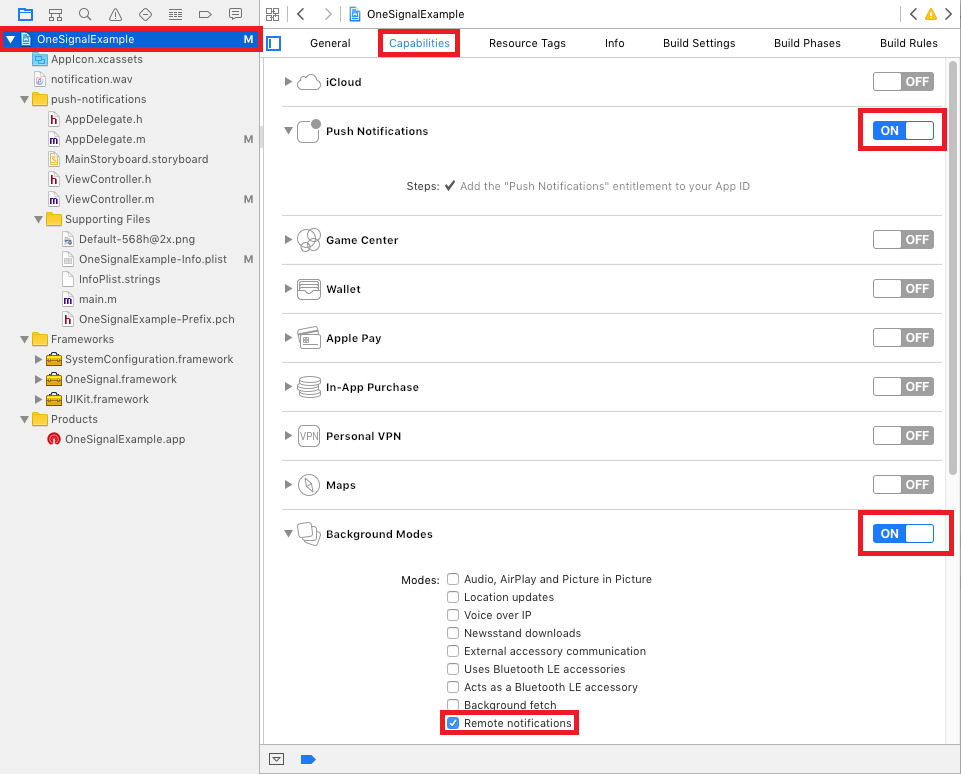
3.2 Open your -Info.p
file add the key CoronaDelegates
as an Array type. Add an entry as OneSignalCoronaDelegate
.

4. Add Plugin to Android or Amazon
4.1 Add the following permissions to your AndroidManifest.xml
file.
<permission android:name="COM.YOUR.PACKAGE_NAME.permission.C2D_MESSAGE"
android:protectionLevel="signature"/>
<uses-permission android:name="COM.YOUR.PACKAGE_NAME.permission.C2D_MESSAGE"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.VIBRATE"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<!-- Keeps the processor from sleeping when a message is received. -->
<uses-permission android:name="android.permission.WAKE_LOCK"/>
<!-- This app has permission to register and receive data message. -->
<uses-permission android:name="com.google.android.c2dm.permission.RECEIVE"/>
<!-- Use to restore notifications the user hasn't interacted with.
They could be missed notifications if the user reboots their device if this isn't in place.
-->
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
4.2 In the application tag, add the following.
<application ...>
<receiver android:name="com.onesignal.NotificationOpenedReceiver" />
<service android:name="com.onesignal.GcmIntentService" />
<service android:name="com.onesignal.GcmIntentJobService"
android:permission="android.permission.BIND_JOB_SERVICE" />
<service android:name="com.onesignal.RestoreJobService"
android:permission="android.permission.BIND_JOB_SERVICE" />
<service android:name="com.onesignal.RestoreKickoffJobService"
android:permission="android.permission.BIND_JOB_SERVICE" />
<service android:name="com.onesignal.SyncService" android:stopWithTask="true" />
<service android:name="com.onesignal.SyncJobService"
android:permission="android.permission.BIND_JOB_SERVICE" />
<activity android:name="com.onesignal.PermissionsActivity"
android:theme="@android:style/Theme.Translucent.NoTitleBar" />
<service android:name="com.onesignal.NotificationRestoreService" />
<receiver android:name="com.onesignal.BootUpReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
<action android:name="android.intent.action.QUICKBOOT_POWERON" />
</intent-filter>
</receiver>
<receiver android:name="com.onesignal.UpgradeReceiver" >
<intent-filter>
<action android:name="android.intent.action.MY_PACKAGE_REPLACED" />
</intent-filter>
</receiver>
</application>
4.3 Optional If you need receive other GCM messages through Solar2D (like Helpshift), then add the following to your AndroidManifest.xml
.
<receiver
android:name="com.onesignal.CoronaGCMFilterProxyReceiver"
android:permission="com.google.android.c2dm.permission.SEND" >
<intent-filter>
<action android:name="com.google.android.c2dm.intent.RECEIVE" />
<category android:name="COM.YOUR.PACKAGE_NAME" />
</intent-filter>
</receiver>
4.4 Replace COM.YOUR.PACKAGE_NAME
with your full package name from the last 2 steps.
4.5 In Eclipse, open your android/project.properties
and add the following.
android.library.reference.2=relative_path/google-play-services_lib
The path you add MUST be relative and not absolute, and should look something like this:
../../../android-sdks/extras/google/google_play_services/libproject/google-play-services_lib
4.6 In Eclipse, build your app. If you get an error about build.xml
missing, then cd
to the google-play-services_lib
folder in the Android SDK and run:
android update lib-project --path . --target android-10
Proguard
If you have Proguard enabled on your project, you must add the following lines to your Proguard config file. The default filename is proguard-project.txt
.
-dontwarn com.onesignal.**
-dontwarn com.gamethrive.**
5. Add Plugin to Amazon
5.1 Follow all the steps from Add Plugin to Android
5.2 Add the following permission to your AndroidManifest.xml
<uses-permission android:name="com.amazon.device.messaging.permission.RECEIVE" />
<permission android:name="COM.YOUR.PACKAGE_NAME.permission.RECEIVE_ADM_MESSAGE" android:protectionLevel="signature" />
<uses-permission android:name="COM.YOUR.PACKAGE_NAME.permission.RECEIVE_ADM_MESSAGE" />
5.3 In the application
tag, add the following:
<application ...>
<amazon:enable-feature android:name="com.amazon.device.messaging" android:required="false" xmlns:amazon="http://schemas.amazon.com/apk/res/android" />
<service android:name="com.onesignal.ADMMessageHandler" android:exported="false" />
<receiver
android:name="com.onesignal.ADMMessageHandler$Receiver"
android:permission="com.amazon.device.messaging.permission.SEND" >
<intent-filter>
<action android:name="com.amazon.device.messaging.intent.REGISTRATION" />
<action android:name="com.amazon.device.messaging.intent.RECEIVE" />
<category android:name="COM.YOUR.PACKAGE_NAME" />
</intent-filter>
</receiver>
</application>
5.4 Replace COM.YOUR.PACKAGE_NAME
with your full package name from the last 2 steps.
5.5. Put your app_key.txt
into the root of your Solar2D project folder. To get this file, follow our Generate an Amazon API Key instructions.
Updated over 1 year ago