Android SDK Setup
Instructions for adding the OneSignal Android Mobile App SDK to your Android and/or Amazon Kindle Fire Apps using Android Studio.
Step 1. Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs
- Android Studio
- An Android 4.1+ device or emulator with "Google Play Store (Services)" installed.
- Set up your Google/Firebase keys in OneSignal.
Step 2. Add OneSignal SDK
Open your App build.gradle (Module: app)
file, add the following to your dependencies
section.
A Gradle build defines the project and its tasks and dependancies. Here we are declaring the OneSignal SDK as an external dependancy for the project. For more details, please view Gradle's documentation on Build Scripts.
implementation 'com.onesignal:OneSignal:[4.0.0, 4.99.99]'
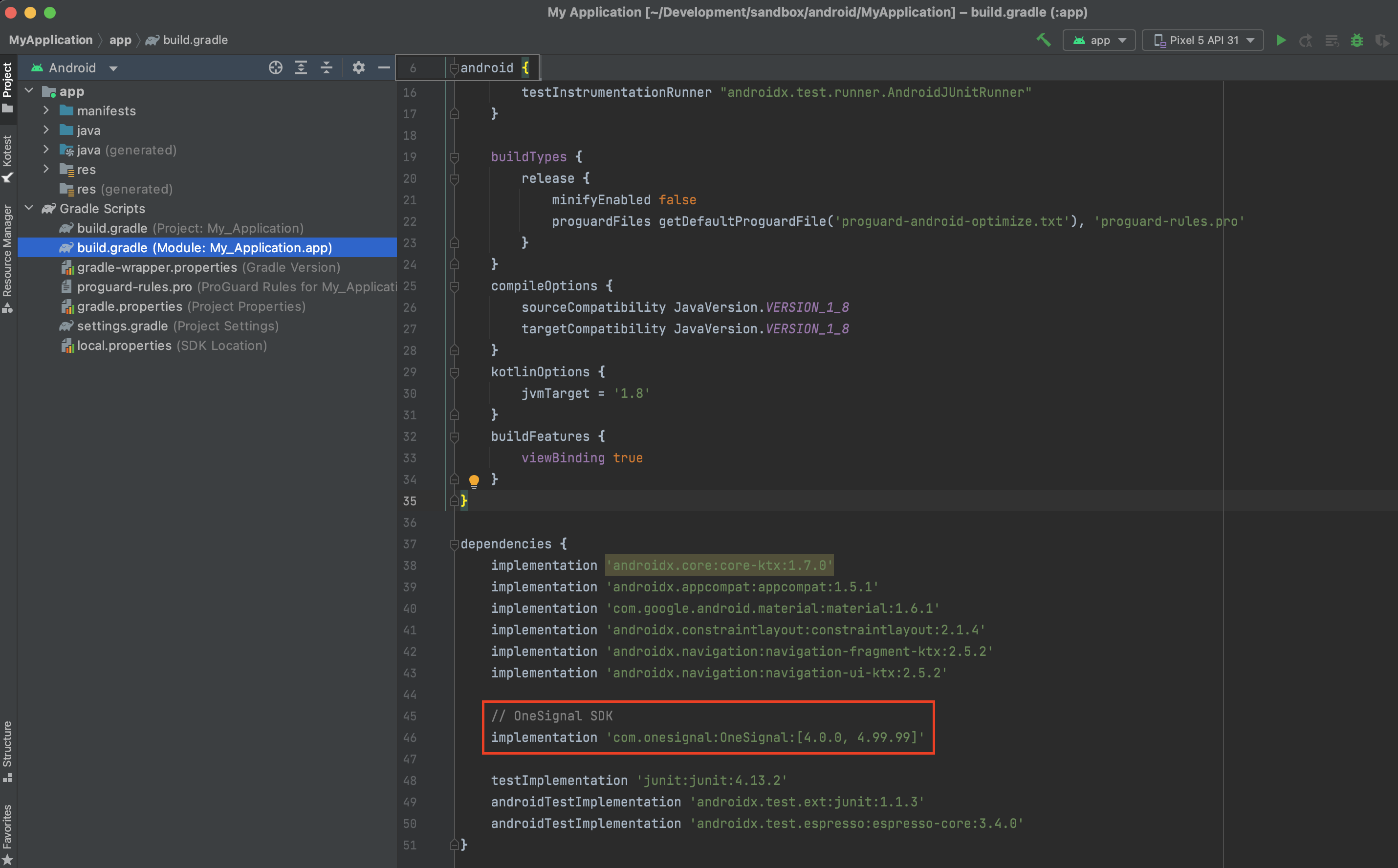
Sync Gradle
Make sure to press "Sync Now" on the top banner that pops up after saving!
Step 3. Add Required Code
Important
Add the following code to the onCreate
method in your Application
class. If you don't have an Application class follow our Create Application Class Guide.
The SDK needs an Android Context to listen to foreground changes to the app. An Activity is also a Context so the SDK can utilize it, however, if you only initialize OneSignal in MainActivity
it may not cover all the entry points to your app. Also, there are edge cases such as the app being cold started but resuming to a specific Activity where it's not going to trigger the onCreate
on your MainActivity
. This is why we recommend following the Create Application Class Guide.
Your ONESIGNAL_APP_ID
can be found in the dashboard Settings > Keys & IDs.
import com.onesignal.OneSignal
// Replace the below with your own ONESIGNAL_APP_ID
const val ONESIGNAL_APP_ID = "########-####-####-####-############"
class ApplicationClass : Application() {
override fun onCreate() {
super.onCreate()
// Logging set to help debug issues, remove before releasing your app.
OneSignal.setLogLevel(OneSignal.LOG_LEVEL.VERBOSE, OneSignal.LOG_LEVEL.NONE)
// OneSignal Initialization
OneSignal.initWithContext(this)
OneSignal.setAppId(ONESIGNAL_APP_ID)
// promptForPushNotifications will show the native Android notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission (See step 7)
OneSignal.promptForPushNotifications();
}
}
import com.onesignal.OneSignal;
public class ApplicationClass extends Application {
// Replace the below with your own ONESIGNAL_APP_ID
private static final String ONESIGNAL_APP_ID = "########-####-####-####-############";
@Override
public void onCreate() {
super.onCreate();
// Enable verbose OneSignal logging to debug issues if needed.
OneSignal.setLogLevel(OneSignal.LOG_LEVEL.VERBOSE, OneSignal.LOG_LEVEL.NONE);
// OneSignal Initialization
OneSignal.initWithContext(this);
OneSignal.setAppId(ONESIGNAL_APP_ID);
// promptForPushNotifications will show the native Android notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission (See step 7)
OneSignal.promptForPushNotifications();
}
}
In Kotlin, the code should be added in the following way:
In Java, the code should be added like so:
Step 4. Customize the default notification icons
Recommended
By default, notifications will be shown with a small bell icon in the notification shade. Follow the Customize Notification Icons guide to create your own small and large notification icons for your app.
Step 5. Run Your App and Send Yourself a Notification
Run your app on a physical device to make sure it builds correctly.
Your Android device should be prompted to subscribe to push notifications if using the promptForPushNotifications
method as shown in the example.
Check your OneSignal Dashboard Audience > Users to see your Device Record.
Then head over to Messages > New Push to Send your first Push Notification from OneSignal.
Troubleshooting
If running into issues, see the Android troubleshooting guide. You can also try the example project on Github.
Try Capturing a Debug Log and if stuck, share the full log with our Support team for assistance:
Step 6. Set Custom User Properties
After initialization, OneSignal will automatically collect common user data by default. Use the following methods to set your own custom user IDs, emails, phone numbers, and other user-level properties.
Set External User ID
Required if using Integrations or Journeys.
OneSignal creates channel-level device records under a unique ID called the player_id
. A single user can have multiple player_id
records based on how many devices, email addresses, and phone numbers they use to interact with your app.
If your app has its own login system to track users, call setExternalUserId
at any time to link all channels to a single user. For more details, see External User IDs.
Set Email and Phone Number
Recommended for messaging across multiple channels (push, email, sms).
Use the provided SDK methods to capture the email address and phone number when provided. Follow the channel quickstart guides for setup:
// Pass in email provided by customer
OneSignal.setEmail("[email protected]");
// Pass in phone number provided by customer
OneSignal.setSMSNumber("+11234567890");
// Pass in email provided by customer
OneSignal.setEmail("[email protected]");
// Pass in phone number provided by customer
OneSignal.setSMSNumber("+11234567890");
Data Tags
All other event and user properties can be set using Data Tags. Setting this data is required for more complex segmentation and message personalization.
OneSignal.sendTag("key", "value");
OneSignal.sendTag("key", "value");
Step 7. Implement a Soft-Prompt In-App Message
Recommended
It is recommended that apps create an alert, modal view, or other interface that describes the types of information they want to send and gives people a clear way to opt in or out.
OneSignal provides an easy option for a "soft-prompt" using In-App Messages to meet this recommendation and have a better user experience. This also permits you to ask for permission again in the future, since the native permission prompt can no longer be shown in your app if the user clicks deny.
See our Push Opt-In Prompt Guide for details on implementing this.
Updated 3 months ago