Huawei SDK setup
OneSignal Huawei SDK Setup Guide for Android Studio.
These instructions are for native apps written in Java or Kotlin. For other supported SDKs see:
- OneSignal Ionic/Cordova/Capacitor setup guide
- OneSignal Flutter SDK setup guide
- OneSignal React Native SDK setup guide
- OneSignal Unity SDK setup guide
Requirements
- Android Studio
- A Huawei device with "Huawei App Gallery" installed
- Configured OneSignal App and Platform
Configure your OneSignal app and platform
If your team already created an account with OneSignal, ask to be invited as an admin role so you can setup the app. Otherwise, sign up for a free account at onesignal.com to get started!
Details on configuring your OneSignal app (click to expand)
You can setup multiple platforms (iOS, Android, Web, Email, SMS) within the same OneSignal App under Settings > Platforms. If you want to create a new app select New App/Website. If this is your first OneSignal app, you will see the next page.
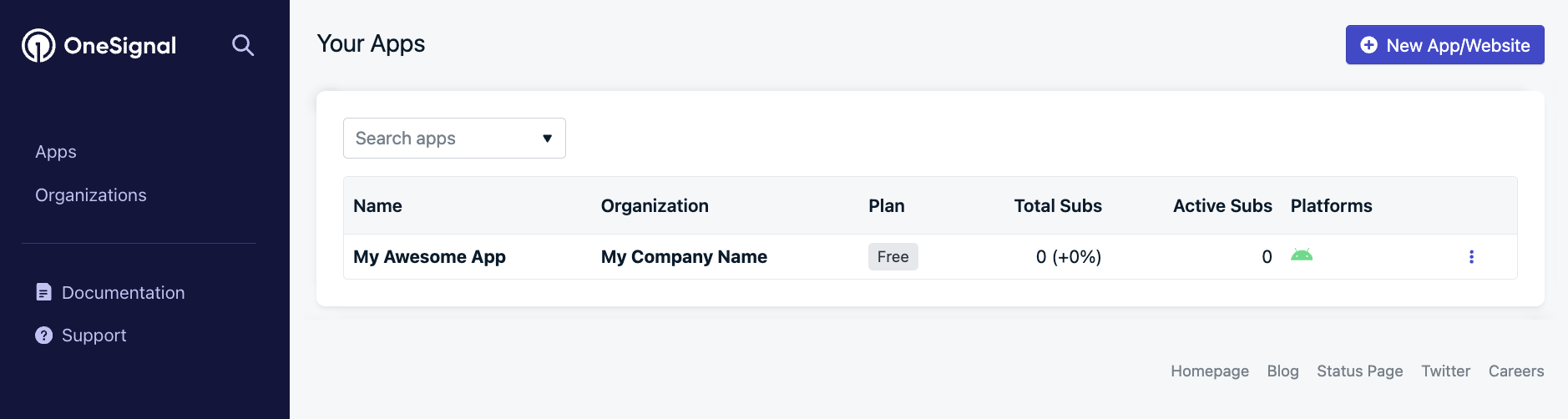
Name your app and organization something recognizable, then select the platform to setup. You can always set up more platforms in this OneSignal App later within Settings > Platforms.
Click Next: Configure Your Platform.
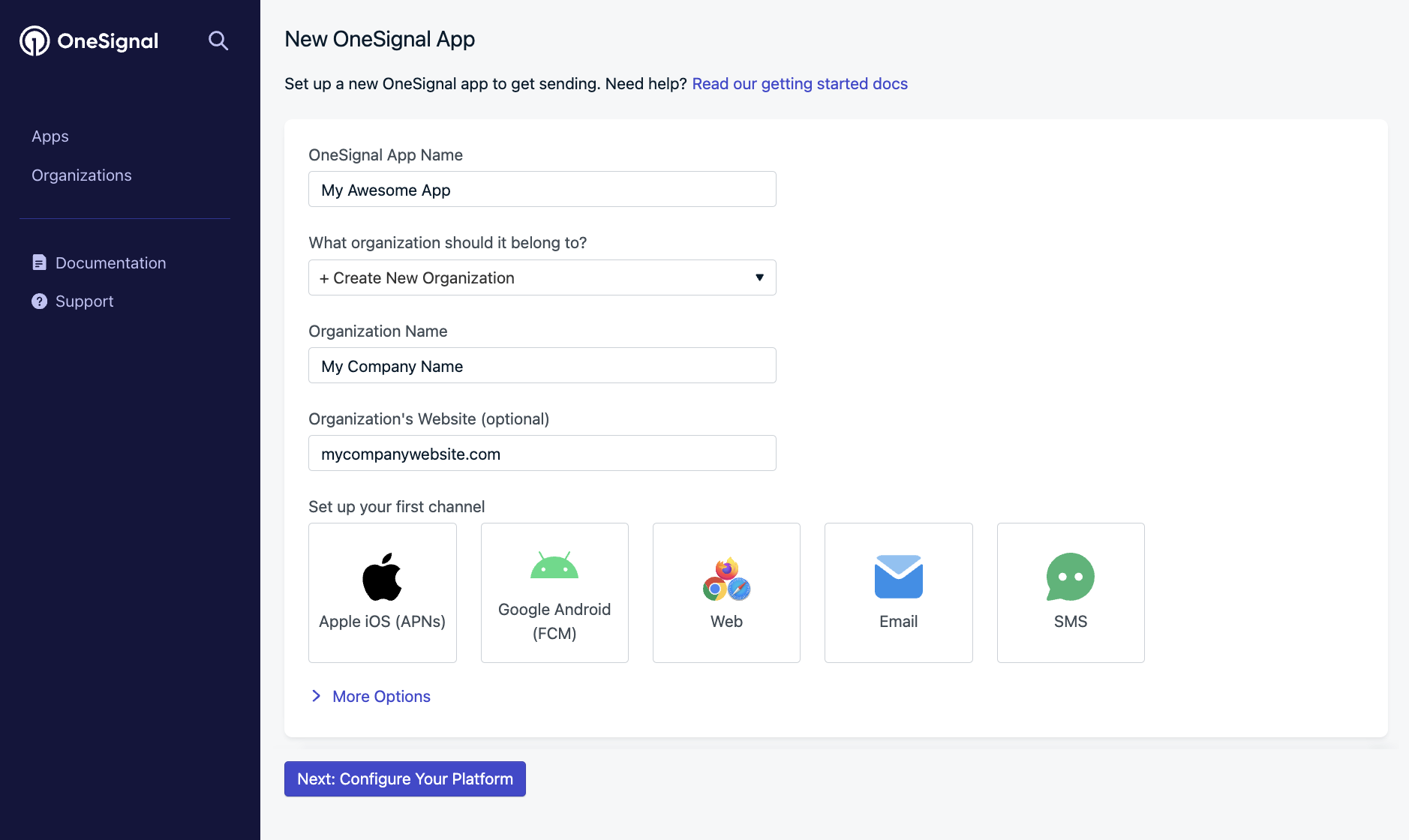
To configure your app, follow the prompts based on the platforms you support.
- Android: Set up your Android Firebase Credentials
- iOS: p8 Authentication Token or p12 Push Notification Certificate
- Amazon: Generate an Amazon API Key
- Huawei: Authorize OneSignal to send Huawei Push
After you setup your credentials, click Save & Continue.
Choose your Apps Target SDK, the click Save & Continue.
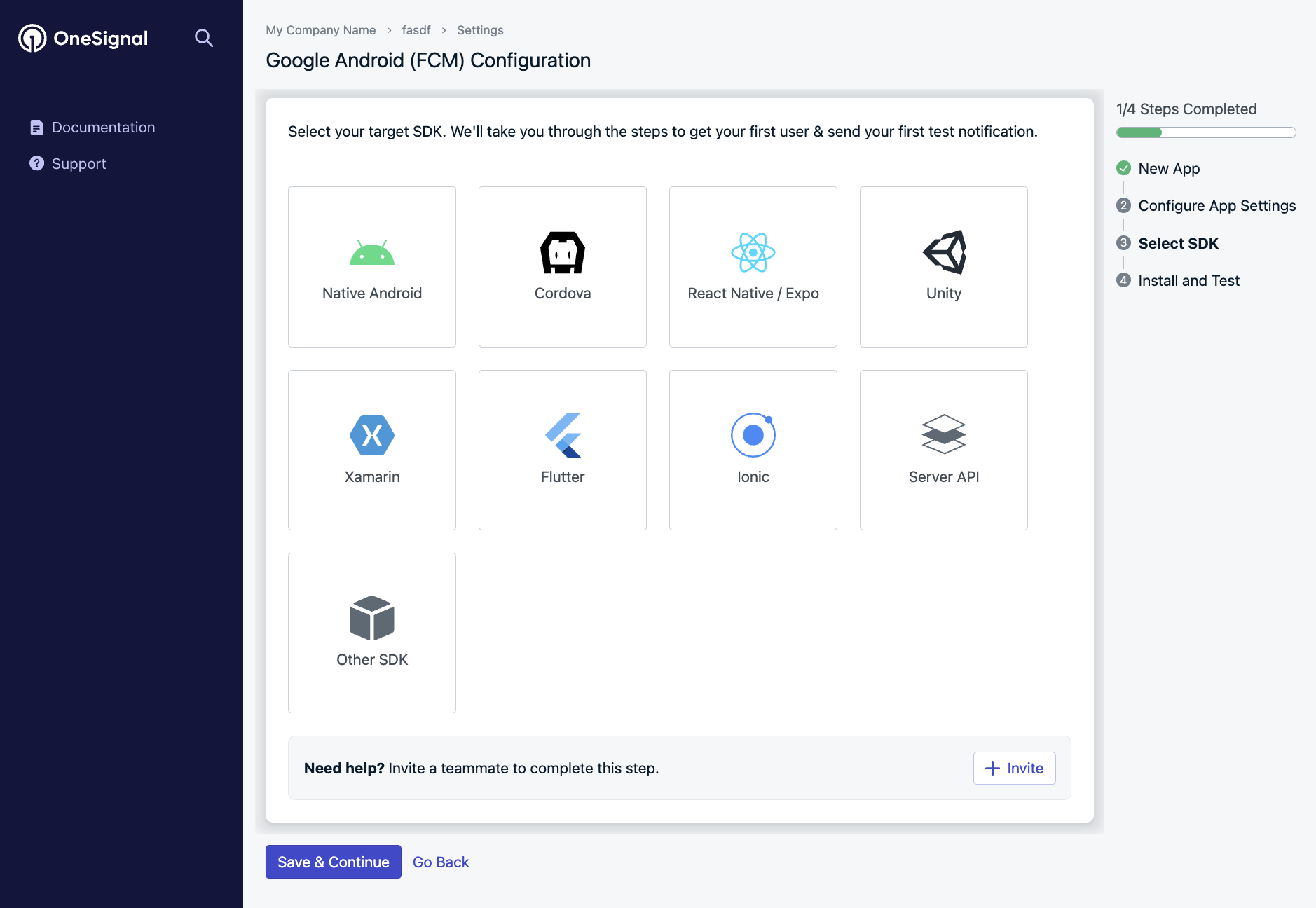
Finally, you will be directed to install the SDK and provided your OneSignal App ID. Make sure to save your App ID as you will need it later.
If you need a teammate or your developer to assist, you can click Invite them to the app and select Done when finished.
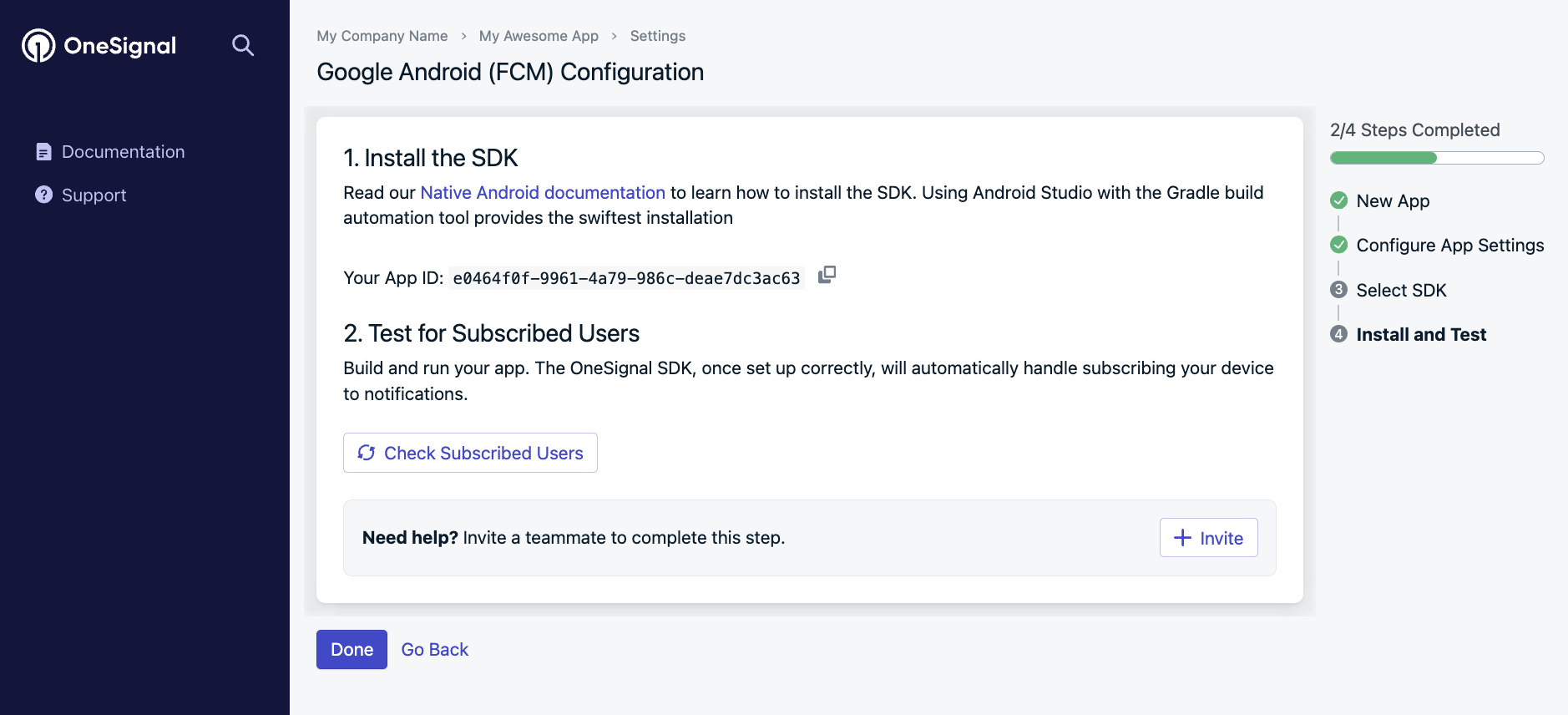
Continue through the documentation to finish adding OneSignal to your app.
Setup
1. Setup the OneSignal SDK
Follow the OneSignal Android SDK setup guide.
Firebase / Google setup not required for app builds released to the Huawei AppGallery.
2. Huawei Configuration File (agconnect-services.json)
You can skip this step if you already have a Huawei agconnect-services.json
in your Android Studio Project from setting up a different Huawei service.
From the AppGallery Connect Project List select your app.
Click on the "agconnect-services.json" button to download this file.
Place this file in your app directory in Android Studio.
3. Generating a Signing Certificate Fingerprint
You can skip this step if you already have added your SHA-256 certificate fingerprint to Huawei's dashboard for a different Huawei service.
From your Android Studio go to View > Tool Windows > Gradle
From here select app > Tasks > android > signingReport
Copy your SHA-256
for your release
variant.
- Optional but recommended for quicker testing is the
SHA-256
for yourdebug
variant too. - You may have other custom variants in your project, if you need push support for them copy these as well.
From the AppGallery Connect Project List select your app.
Scroll to the bottom to find the "SHA-256 certificate fingerprint" field were you should enter your keys.
4. Add Huawei Gradle Plugin and Dependencies
Open your root build.gradle
(Project: ) in Android Studio and add maven {url 'https://developer.huawei.com/repo/'}
under buildscript { repositories }
and allprojects { repositories }
Under buildscript { dependencies }
add classpath 'com.huawei.agconnect:agcp:1.6.5.300'
You should have in total 3 new lines in your root build.gradle
, highlighted below.
Open your app/build.gradle
file and add implementation 'com.huawei.hms:push:6.3.0.304' under the
dependencies` section.
Also to the app/build.gradle
file add apply plugin: 'com.huawei.agconnect'
to the very bottom of the file.
You should have in total 2 new lines in your app/build.gradle
, highlighted below.
Sync Gradle
Make sure to press "Sync Now" on the banner that pops up after saving!
5. Compatibility with other HMS push libraries or your own HmsMessageService
class"
HmsMessageService
class"This is only required if you must keep another 3rd-party HMS push SDK / Library in your app in-addition to OneSignal or you have your own HmsMessageService
.
Create a class that extends from HmsMessageService
, if you don't have one already and add the following methods.
extends HmsMessageService
If you already had a class that extends
HmsMessageService
please add the two newOneSignalHmsEventBridge
lines instead of creating another class.
public class YourHmsMessageService extends HmsMessageService {
public void onNewToken(String token) {
// ...
// Forward event on to OneSignal SDK
OneSignalHmsEventBridge.onNewToken(this, token);
}
@Override
public void onMessageReceived(RemoteMessage message) {
// ...
// Forward event on to OneSignal SDK
OneSignalHmsEventBridge.onMessageReceived(this, message);
}
}
This is to forward onNewToken
and onMessageReceived
to OneSignal via the OneSignalHmsEventBridge
.
If you didn't have a class that extended HmsMessageService
before make sure to add it to your AndroidManifest.xml
under the <application>
tag.
<application>
...
<!--
Ensure you only have one intent-filter for "com.huawei.push.action.MESSAGING_EVENT".
HMS only supports one per app.
-->
<service
android:name=".YourHmsMessageService"
android:exported="false">
<intent-filter>
<action android:name="com.huawei.push.action.MESSAGING_EVENT" />
</intent-filter>
</service>
...
</application>
6. Run and test your app
Run your app on real Huawei device to make sure your device is subscribed to notifications as a Huawei device and can receive notifications sent from the OneSignal dashboard.
Troubleshooting
If you run into any issues please see our Android troubleshooting guide
You can see a fully implemented example project on Github.
7. Omit Google Libraries for Huawei AppGallery builds (Optional)
Step 7 - Option 1
If your app will only be available on the Huawei AppGallery and you want to omit any Google related dependencies that OneSignal includes you can use exclude
with implementation
in your app/build.gradle
.
implementation('com.onesignal:OneSignal:[5.0.0-beta, 5.99.99]') {
exclude group: 'com.google.android.gms'
exclude group: 'com.google.firebase'
}
Step 7 - Option 2
If your app will be for both the Google Play Store and Huawei AppGallery and you want to be selective on which libraries you will include to make your APK smaller you can add dependencies based on buildTypes or variants.
Example:
android {
// ...
buildTypes {
debug {
}
release {
}
// **** Add your custom hauwei buildType here
huawei {
}
}
}
dependencies {
// **** Use the full OneSignal dependencies for Google Play Store builds
debugImplementation 'com.onesignal:OneSignal:[5.0.0, 5.99.99]'
releaseImplementation 'com.onesignal:OneSignal:[5.0.0, 5.99.99]'
// **** Only include hms:push for your Huawei AppGallery builds.
huaweiImplementation 'com.huawei.hms:push:6.3.0.304'
// Omit Google / Firebase libraries for Huawei builds.
huaweiImplementation('com.onesignal:OneSignal:[5.0.0, 5.99.99]') {
exclude group: 'com.google.android.gms'
exclude group: 'com.google.firebase'
}
}
8. Prefer HMS over FCM (Optional)
If you did step " 7. Omit Google Libraries for Huawei AppGallery builds (Optional)" above this step doesn't apply.
By default OneSignal prefers using FCM over HMS if both are included in your app. If you like to change this to prefer HMS instead you can add the following to your AndroidManifest.xml
:
<application>
...
<meta-data android:name="com.onesignal.preferHMS" android:value="true"/>
...
</application>
Common Troubleshooting issues.
While testing, make sure to keep the OneSignal setLogLevel
method set to VERBOSE.
Check the logs to see any errors being thrown and Huawei Common Error Codes.
Getting notification_types: -25
"notification_types":-25
means OneSignal timed out waiting for a response from Huawei's HMS to get a push token. This is most likely due to another 3rd-party HMS push SDK or your own HmsMessageService getting this event instead of OneSignal.
Please refer to the above step on how to check this and forward the event if this is the case.
Getting notification_types: -28
This means there is a class HMS is missing from the app that is needed for push. Just having com.huawei.hms:push
in the build.gradle
will cause this specific error not to happen any more. However, if you have some aggressive Proguard or R8 settings, this might cause issues. We recommend turn off minifyEnabled
temporary if you have it to see if that is the root of the issue.
Also, you shouldn't mix and match major release versions of other HMS libraries. Start with either 4 or 5. Make sure not to have a mixture from 3 to 5 which is going to create other errors
Error getting Huawei Push Token
E/OneSignal: HMS ApiException getting Huawei push token!
com.huawei.hms.common.ApiException: -5: Core error
Check your Proguard or R8 rules to make sure they are setup properly. Possibly disable it temporarily to see if it is related. If it fixes the issue after disabling Proguard or R8 then you can follow this guide and turn it back on:
- Configuring Obfuscation Scripts:
https://developer.huawei.com/consumer/en/doc/development/HMS-Guides/Preparations#h1-1575707474823
Updated 3 months ago