.NET MAUI SDK setup
Instructions for adding OneSignal .NET SDK to your cross-platform MAUI app for iOS, Android, and Desktop.
Requirements
- .NET 7.0+
- Visual Studio 2019 or newer
- iOS: iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- iOS: mac with Xcode 12+
- Android: Android 5.0+ device or emulator with "Google Play Store (Services)" installed
- Configured OneSignal App and Platform
Configure your OneSignal app and platform
If your team already created an account with OneSignal, ask to be invited as an admin role so you can setup the app. Otherwise, sign up for a free account at onesignal.com to get started!
Details on configuring your OneSignal app (click to expand)
You can setup multiple platforms (iOS, Android, Web, Email, SMS) within the same OneSignal App under Settings > Platforms. If you want to create a new app select New App/Website. If this is your first OneSignal app, you will see the next page.
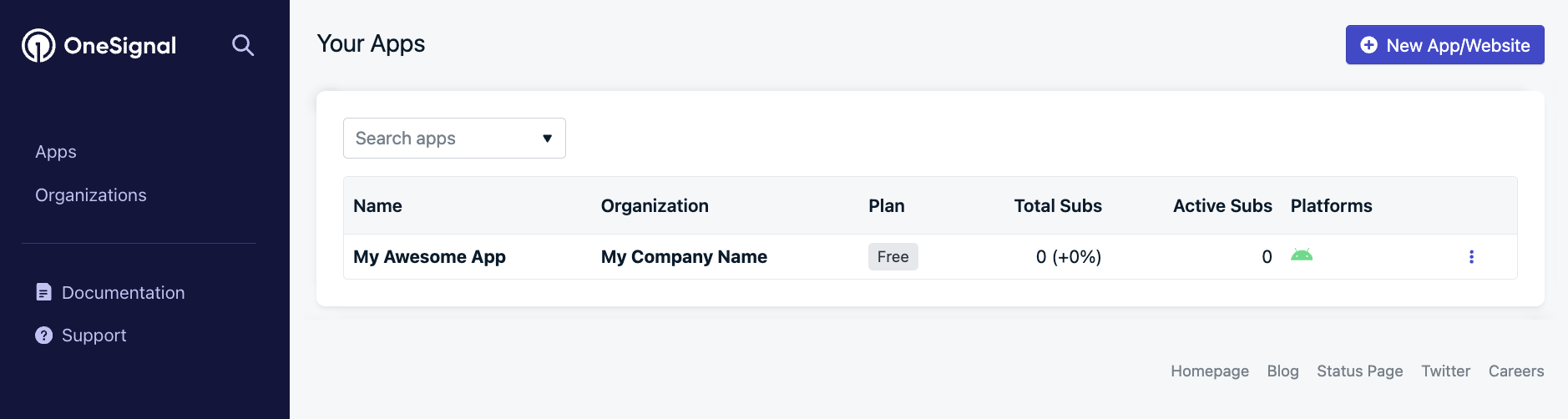
Name your app and organization something recognizable, then select the platform to setup. You can always set up more platforms in this OneSignal App later within Settings > Platforms.
Click Next: Configure Your Platform.
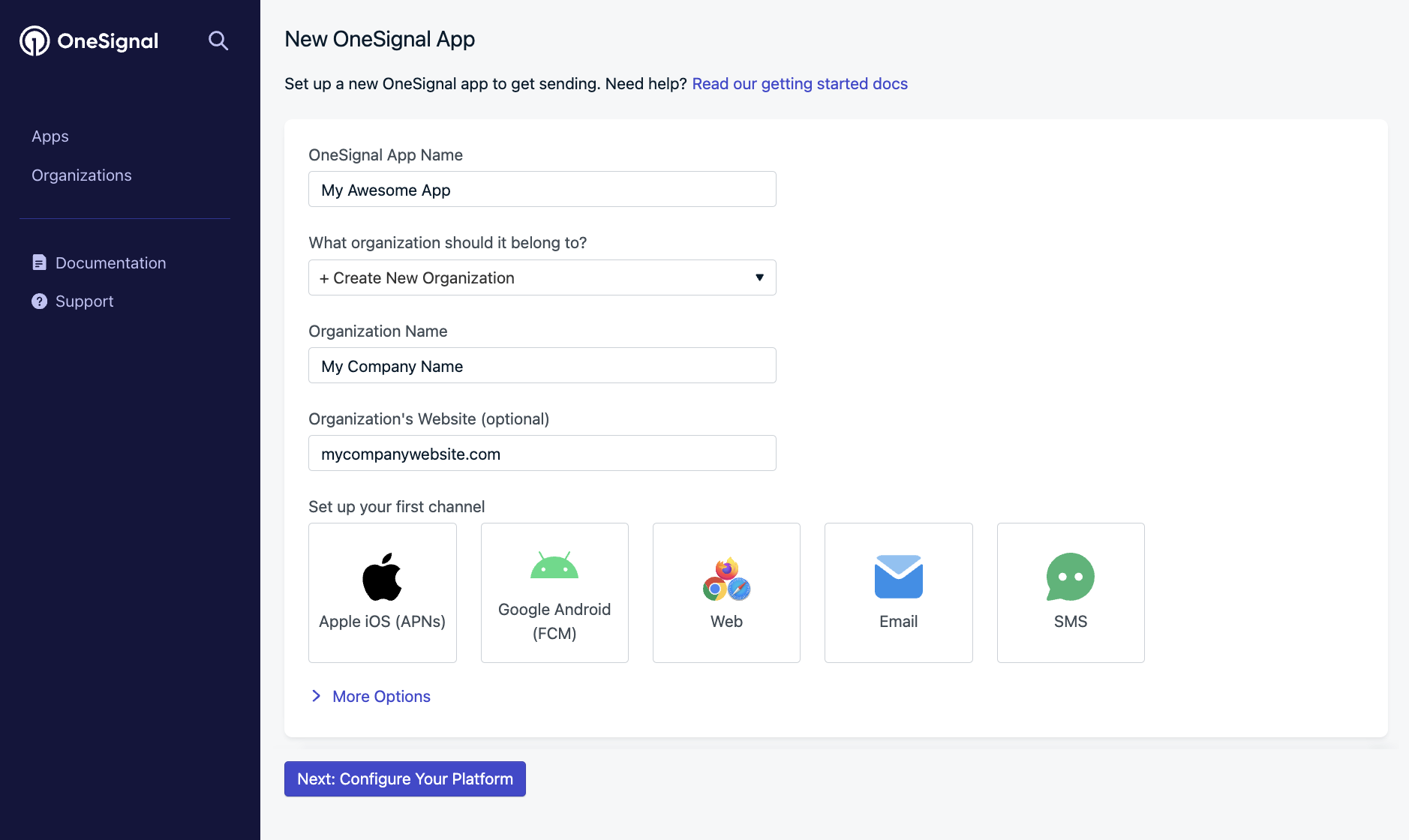
To configure your app, follow the prompts based on the platforms you support.
- Android: Set up your Android Firebase Credentials
- iOS: p8 Authentication Token or p12 Push Notification Certificate
- Amazon: Generate an Amazon API Key
- Huawei: Authorize OneSignal to send Huawei Push
After you setup your credentials, click Save & Continue.
Choose your Apps Target SDK, the click Save & Continue.
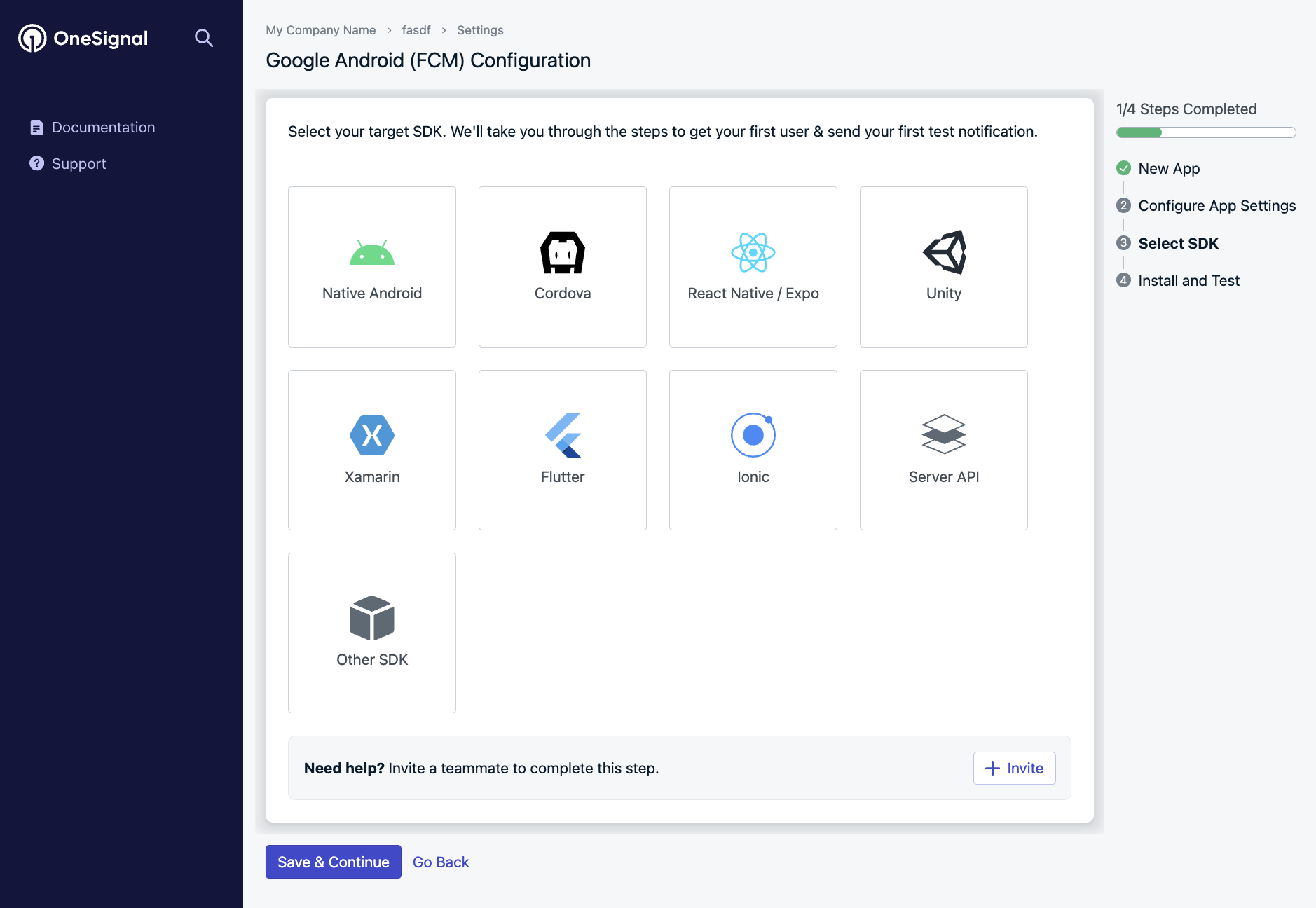
Finally, you will be directed to install the SDK and provided your OneSignal App ID. Make sure to save your App ID as you will need it later.
If you need a teammate or your developer to assist, you can click Invite them to the app and select Done when finished.
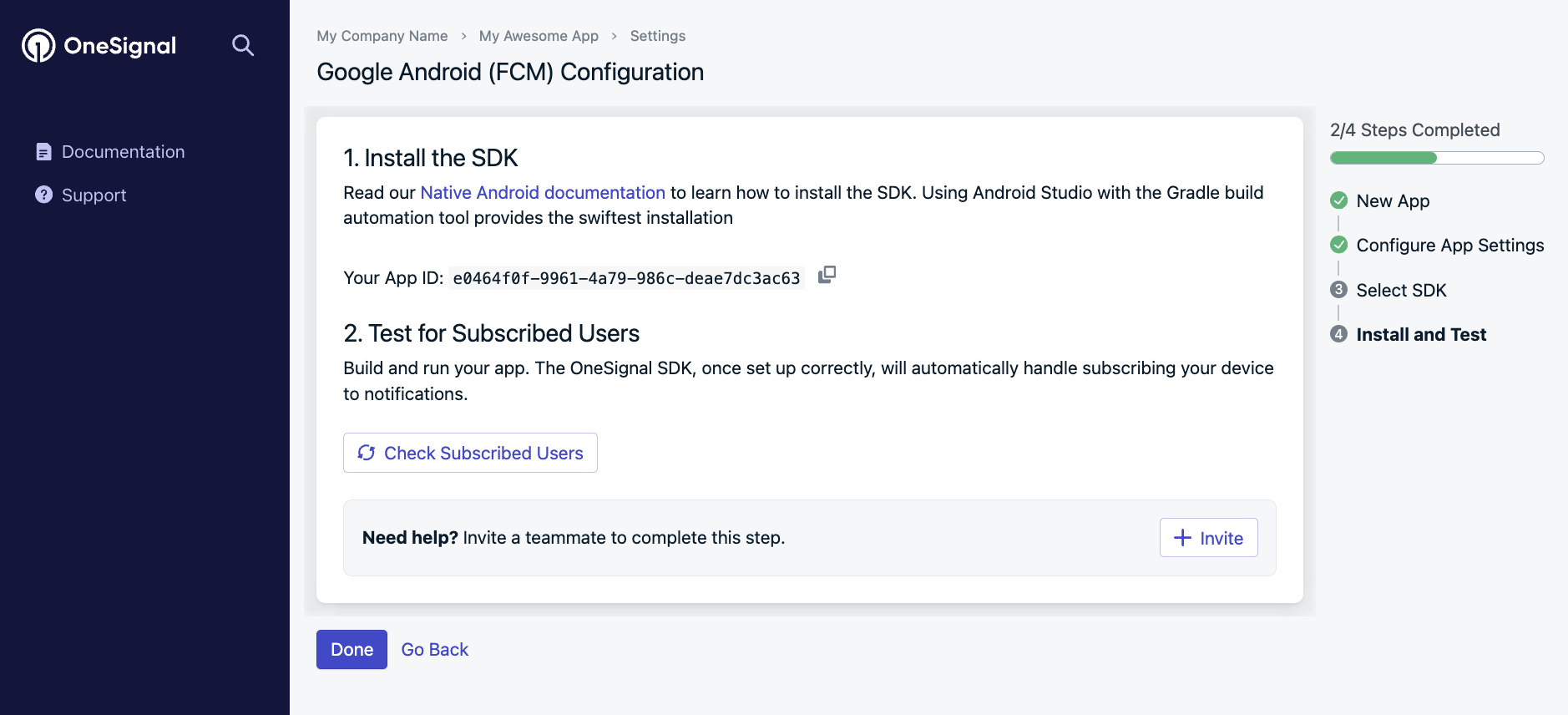
Continue through the documentation to finish adding OneSignal to your app.
Choosing your IDE
With the deprecation of Visual Studio for Mac, the official suggestion from Microsoft is to use VSCode along with the newly released .NET maui extension (please keep in mind this extension is currently in "preview"). Another option available to Mac users is Rider.
Setup
1. Add our SDK
Using the .NET CLI :
dotnet add package OneSignalSDK.DotNet
2. Initialization
Note on Xamarin Forms
Xamarin Forms is no longer supported beyond version 5.0.2 of our .NET SDK. Microsoft has guides on migrating to Maui here, if needed.
.NET MAUI
Initialize OneSignal in App.xaml.cs in your MAUI project as follows:
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.Core;
using OneSignalSDK.DotNet.Core.Debug;
public App() {
InitializeComponent();
// Enable verbose OneSignal logging to debug issues if needed.
OneSignal.Debug.LogLevel = LogLevel.VERBOSE;
// OneSignal Initialization
OneSignal.Initialize("YOUR_ONESIGNAL_APP_ID");
// RequestPermissionAsync will show the notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission (See step 5)
OneSignal.Notifications.RequestPermissionAsync(true);
MainPage = new AppShell();
}
3. Adding a Service Extension
Microsoft has a guide on generating a Notification Service Extension here, using Visual Studio and Visual Studio for Mac, but does not have any guidance on how you would create this for VSCode at this time.
If you generate a service extension following the guide above, you can follow this the code in our sample project found on Github
using System;
using Foundation;
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.iOS;
using UIKit;
using UserNotifications;
namespace OneSignalNotificationServiceExtension
{
[Register("NotificationService")]
public class NotificationService : UNNotificationServiceExtension
{
Action<UNNotificationContent> ContentHandler { get; set; }
UNMutableNotificationContent BestAttemptContent { get; set; }
UNNotificationRequest ReceivedRequest { get; set; }
protected NotificationService(IntPtr handle) : base(handle)
{
// Note: this .ctor should not contain any initialization logic.
}
public override void DidReceiveNotificationRequest(UNNotificationRequest request, Action<UNNotificationContent> contentHandler)
{
ReceivedRequest = request;
ContentHandler = contentHandler;
BestAttemptContent = (UNMutableNotificationContent)request.Content.MutableCopy();
NotificationServiceExtension.DidReceiveNotificationExtensionRequest(request, BestAttemptContent, contentHandler);
}
public override void TimeWillExpire()
{
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
NotificationServiceExtension.ServiceExtensionTimeWillExpireRequest(ReceivedRequest, BestAttemptContent);
ContentHandler(BestAttemptContent);
}
}
}
4. Testing
Run your app on a physical device to make sure it builds correctly.
If you used the provided code, then the requestPermission
method, should prompt you to subscribe to push notifications. You can always change this later but for now, click "Allow" to subscribe to push notifications.
Check your OneSignal Dashboard Audience > Subscriptions to see your Subscription and click the options button on the left to set yourself as a Test Subscription.
Troubleshooting
If running into issues, see our Mobile Troubleshooting Guide.
Try our example projects on our Github repository.
If stuck, contact support directly or email [email protected] for help.
For faster assistance, please provide:
- Your OneSignal App ID
- Details, logs, and/or screenshots of the issue.
- Steps to reproduce
Users & subscriptions
Required if using integrations.
Recommended for messaging across multiple channels (push, email, sms).
If you need user consent before tracking their data. OneSignal provides user consent methods to help delay initialization of our SDK until consent is provided. See Handling Personal Data for details.
External ID & aliases
When a user downloads and opens your mobile app or uninstalls and re-installs your app on the same device, a Subscription and a User is created within the OneSignal app.
You can identify that user across multiple subscriptions by setting the External ID property. The External ID should be distinct user ID representing a single user. We recommend using the same user ID as in your Integrations or main analytics tool.
When you authenticate users in your app, call our login
method at any time to link this subscription to a user.
var externalId = "123456789" // You will supply the external id to the OneSignal SDK
OneSignal.login(externalId);
Our mobile SDKs have methods for detecting User state changes that might be helpful for internal tracking.
External ID & custom aliases
If your users have multiple user IDs, we do support additional custom Aliases. However, you should still always set the External ID as this is the main alias we use to identify users. See Users for details.
Add email and phone number subscriptions
Recommended if using email and SMS messaging.
Like push subscriptions, email addresses and phone numbers each are a new subscription within OneSignal. Your email and sms subscriptions will be tied to the same user if created using our SDK or if you setting the External ID. You can Import your current user data and use our APIs and/or SDK methods to capture new email addresses and phone numbers when provided to you by your users.
// Pass in email provided by customer
OneSignal.User.addEmail("[email protected]");
// Pass in phone number provided by customer
OneSignal.User.addSms("+11234567890");
Users & subscriptions
See Users and Subscriptions for more details.
Property & event tags
Tags are custom key : value
pairs of String data used for tracking any custom user events and properties. Setting tags is required for more complex Segments and Message Personalization.
For event triggered messages, use tags with Time Operators to note the date and time the event is/occurred and setup automations for sending to those users. See Abandoned Cart Example for details.
OneSignal.User.addTagWithKey("test1", "val1");
Data tags
If you want to store custom data within OneSignal for segmentation, message personalization, and event triggered automation, see Tags for details.
Importing users and subscriptions
If you have a list of user data from a previous source, you can import it into OneSignal following our Import guides.
Message configuration
Common setup items to get the most of your integration.
Deep linking
See our Deep Linking guide to set those up for push and other messaging channels.
Basic SDK setup complete!
For details on the above methods and other methods available, see our Mobile SDK reference.
Updated 4 months ago