Expo SDK setup
Instructions for adding the OneSignal React Native & Expo SDK to your app for iOS, Android, and derivatives like Amazon.
Expo Managed Workflow
This documentation shows how to use our SDK with your Expo Managed Workflow application.
If you are using the Bare Workflow, please review the React Native + Expo SDK Setup instead.
Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs
iOS requirements
- iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- mac with Xcode 12+
- p8 Authentication Token or p12 Push Notification Certificate
- Your
app.json
oreas.json
orapp.config.js
file needs the iOSbundleIdentifier
property set. See Expo > Configure development and production variants.
Android requirements
- Android 5.0+ device or emulator with "Google Play Store (Services)" installed
- Set up your Android Firebase Credentials
Amazon & Huawei requirements
Follow these instructions if your app is distributed on the Amazon AppStore and/or the Huawei AppGallery.
1. Add the OneSignal package to your app
OneSignal Expo sample app
Sample repo containing the sample app for running OneSignal with Expo.
Make sure you have the Expo CLI installed on your computer.
1.1 Install the OneSignal Expo plugin using the Expo CLI
npx expo install onesignal-expo-plugin
1.2 Install the SDK using Yarn or NPM
- Yarn:
yarn add react-native-onesignal
- NPM
npm install --save react-native-onesignal
1.3 Configure your app.json/app.config.js
Add the plugin to the plugin array and ensure that your bundle identifier is set for iOS development:
app.json
{
"ios": {
"supportsTablet": true,
"bundleIdentifier": "com.onesignal.example"
},
"plugins": [
[
"onesignal-expo-plugin",
{
"mode": "development",
}
]
]
}
or
app.config.js
export default {
...
plugins: [
ios: {
bundleIdentifier: "com.onesignal.example", // Define the bundle ID here
supportsTablet: true,
},
[
"onesignal-expo-plugin",
{
mode: "development",
}
]
]
};
Plugin Prop
You can pass props to the plugin config object to configure:
Plugin Prop | ||
---|---|---|
mode | required | Used to configure APNs environment entitlement. "development" or "production" |
devTeam | optional | Used to configure Apple Team ID. You can find your Apple Team ID by running expo credentials:manager e.g: "91SW8A37CR" |
iPhoneDeploymentTarget | optional | Target IPHONEOS_DEPLOYMENT_TARGET value to be used when adding the iOS NSE. A deployment target is nothing more than the minimum version of the operating system the application can run on. This value should match the value in your Podfile e.g: "14.5" . |
smallIcons | optional | An array of local paths to small notification icons for Android. Image should be white, transparent, and 96x96 in size. Input images will be automatically scaled down and placed in the appropriate resource folders. e.g: ["./assets/ic_stat_onesignal_default.png"] . See https://documentation.onesignal.com/docs/customize-notification-icons#small-notification-icons. |
largeIcons | optional | An array of local paths to large notification icons for Android. Image should be white, transparent, and 256x256 in size. e.g: ["./assets/ic_onesignal_large_icon_default.png"] . See https://documentation.onesignal.com/docs/customize-notification-icons#large-notification-icons. |
iosNSEFilePath | optional | The local path to a custom Notification Service Extension (NSE), written in Objective-C. The NSE will typically start as a copy of the default NSE, then altered to support any custom logic required. e.g: "./assets/NotificationService.m" . |
1.4 Adding the OneSignal App ID
Add your OneSignal App ID to your Expo constants via the extra param:
{
"extra": {
"oneSignalAppId": "<YOUR APP ID HERE>"
}
}
You can then access the value to pass to the initialize function:
import { LogLevel, OneSignal } from 'react-native-onesignal';
import Constants from "expo-constants";
OneSignal.Debug.setLogLevel(LogLevel.Verbose);
OneSignal.initialize(Constants.expoConfig.extra.oneSignalAppId);
// Also need enable notifications to complete OneSignal setup
OneSignal.Notifications.requestPermission(true);
Alternatively, pass the OneSignal app ID directly to the function:
OneSignal.initialize("YOUR-ONESIGNAL-APP-ID");
1.5 Run your Expo app
You need to create a development build for your app.
$ npx expo prebuild
# Build your native iOS project
$ npx expo run:ios
# Build your native Android project
$ npx expo run:android
2. Testing
Run your app on a physical device to make sure it builds correctly.
If you used the provided code, then the requestPermission
method, should prompt you to subscribe to push notifications. You can always change this later but for now, click "Allow" to subscribe to push notifications.
Check your OneSignal Dashboard Audience > Subscriptions to see your Subscription and click the options button on the left to set yourself as a Test Subscription.
Troubleshooting
If running into issues, see our Mobile Troubleshooting Guide.
Try our example projects on our Github repository.
If stuck, contact support directly or email support@onesignal.com for help.
For faster assistance, please provide:
- Your OneSignal App ID
- Details, logs, and/or screenshots of the issue.
- Steps to reproduce
PBXGroup Error
You may see this error when building with Xcode 16.
RuntimeError -
PBXGroup
attempted to initialize an object with unknown ISAPBXFileSystemSynchronizedRootGroup
from attributes:{"isa"=>"...", "exceptions"=>["//", "..."], "explicitFileTypes"=>{}, "explicitFolders"=>[], "path"=>"OneSignalNotificationServiceExtension", "sourceTree"=>"<group>"}
Check the error for the folder name under "path"
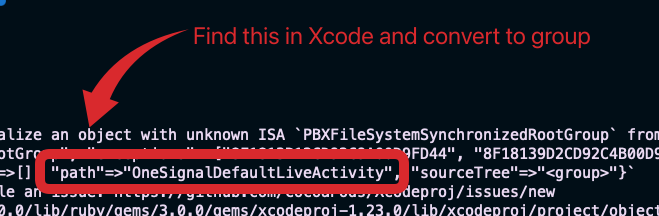
Then in your project, open your XCWorkspace folder in Xcode and open the project directory in the left sidebar.
From here, you should see the folders corresponding with the the targets you have available on this project. You should find folder that matches the "path" in the error you've received. Update the folder to "Convert to Group".
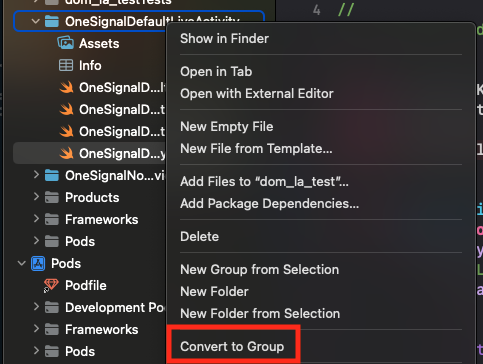
Cycle Inside Error
You may see this error when building with Xcode 15 or higher, which is caused by a Xcode 15+ default configuration change affecting cross platform systems.
Error: Cycle inside SampleProject; building could produce unreliable results.
In your project, open your XCWorkspace folder in Xcode and open the project directory in the left sidebar.
Select your app target and navigate to the "Build Phases" tab. You should have a phase called "Embed App Extensions" or "Embed Foundation Extensions". Drag and move this build phase to above "Run Script". Your error should now be resolved.
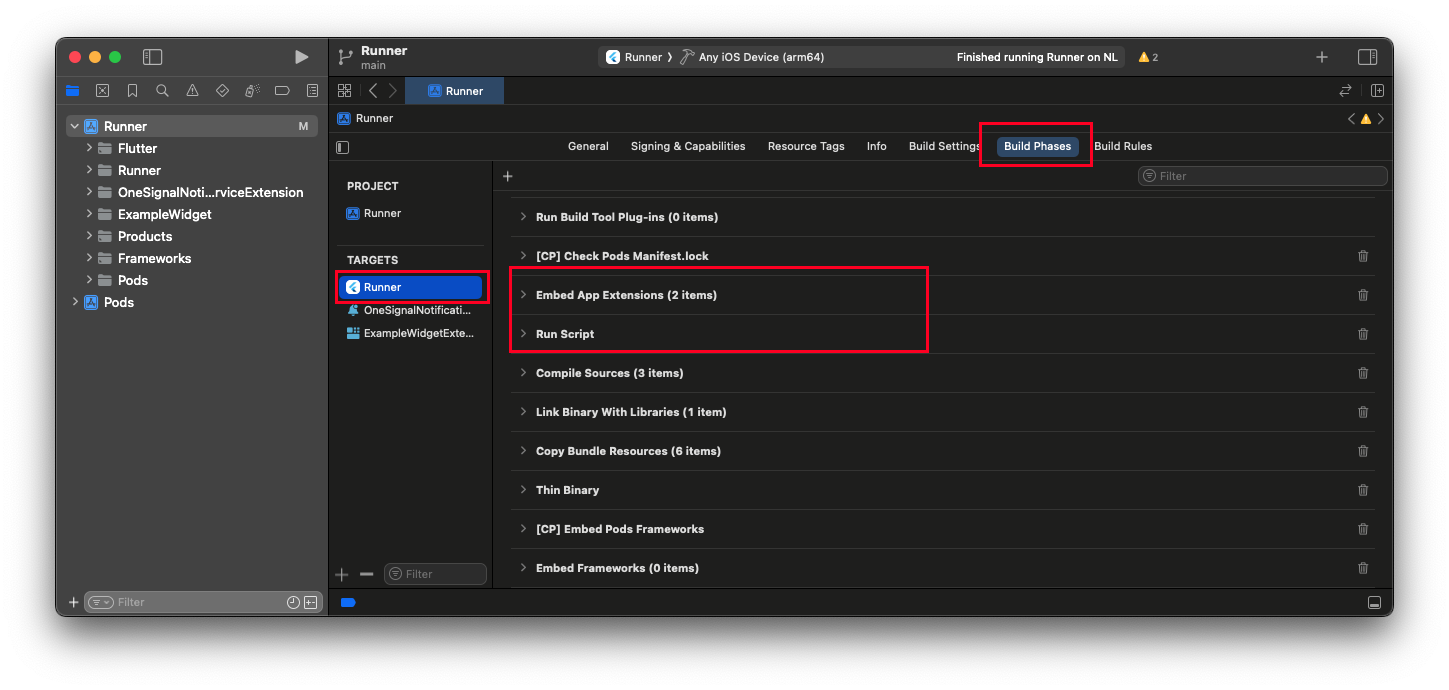
If that does not fix the error, you may need to un-check "Copy only when installing". This option can be found when you expand the build phase that you just moved.
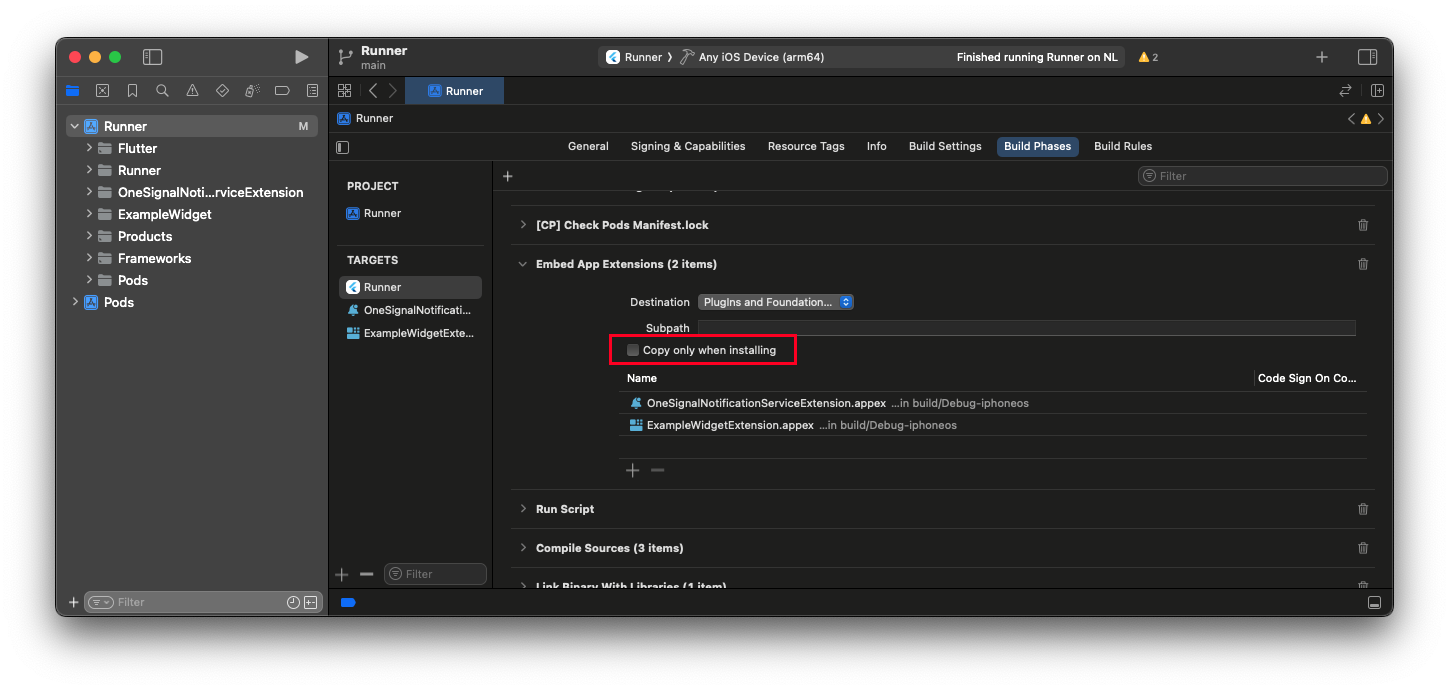
Users & subscriptions
Required if using integrations.
Recommended for messaging across multiple channels (push, email, sms).
If you need user consent before tracking their data. OneSignal provides user consent methods to help delay initialization of our SDK until consent is provided. See Handling Personal Data for details.
External ID & aliases
When a user downloads and opens your mobile app or uninstalls and re-installs your app on the same device, a Subscription and a User is created within the OneSignal app.
You can identify that user across multiple subscriptions by setting the External ID property. The External ID should be distinct user ID representing a single user. We recommend using the same user ID as in your Integrations or main analytics tool.
When you authenticate users in your app, call our login
method at any time to link this subscription to a user.
let externalId = "123456789" // You will supply the external id to the OneSignal SDK
OneSignal.login(externalId);
Our mobile SDKs have methods for detecting User state changes that might be helpful for internal tracking.
External ID & custom aliases
If your users have multiple user IDs, we do support additional custom Aliases. However, you should still always set the External ID as this is the main alias we use to identify users. See Users for details.
Add email and phone number subscriptions
Recommended if using email and SMS messaging.
Like push subscriptions, email addresses and phone numbers each are a new subscription within OneSignal. Your email and sms subscriptions will be tied to the same user if created using our SDK or if you setting the External ID. You can Import your current user data and use our APIs and/or SDK methods to capture new email addresses and phone numbers when provided to you by your users.
// Pass in email provided by customer
OneSignal.User.addEmail("example@domain.com");
// Pass in phone number provided by customer
OneSignal.User.addSms("+11234567890");
Users & subscriptions
See Users and Subscriptions for more details.
Property & event tags
Tags are custom key : value
pairs of String data used for tracking any custom user events and properties. Setting tags is required for more complex Segments and Message Personalization.
For event triggered messages, use tags with Time Operators to note the date and time the event is/occurred and setup automations for sending to those users. See Abandoned Cart Example for details.
OneSignal.User.addTag("key", "value");
Data tags
If you want to store custom data within OneSignal for segmentation, message personalization, and event triggered automation, see Tags for details.
Importing users and subscriptions
If you have a list of user data from a previous source, you can import it into OneSignal following our Import guides.
Message configuration
Common setup items to get the most of your integration.
Deep linking
See our Deep Linking guide to set those up for push and other messaging channels.
Basic SDK setup complete!
For details on the above methods and other methods available, see our Mobile SDK reference.
Updated 5 days ago