React Native SDK setup
Instructions for adding the OneSignal React Native to your app for iOS, Android, and derivatives like Amazon
Requirements
- A bare React Native app. If using a managed Expo app, see Expo SDK Setup.
- iOS: iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- iOS: mac with Xcode 12+
- Android: Android 5.0+ device or emulator with "Google Play Store (Services)" installed
- Configured OneSignal App and Platform
Configure your OneSignal app and platform
If your team already created an account with OneSignal, ask to be invited as an admin role so you can setup the app. Otherwise, sign up for a free account at onesignal.com to get started!
Details on configuring your OneSignal app (click to expand)
You can setup multiple platforms (iOS, Android, Web, Email, SMS) within the same OneSignal App under Settings > Platforms. If you want to create a new app select New App/Website. If this is your first OneSignal app, you will see the next page.
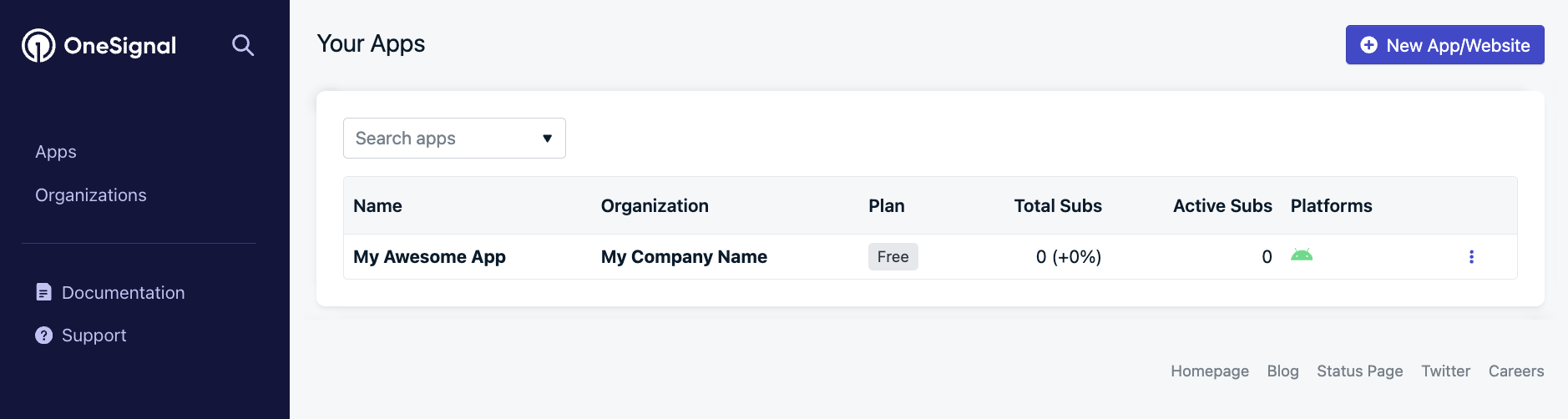
Name your app and organization something recognizable, then select the platform to setup. You can always set up more platforms in this OneSignal App later within Settings > Platforms.
Click Next: Configure Your Platform.
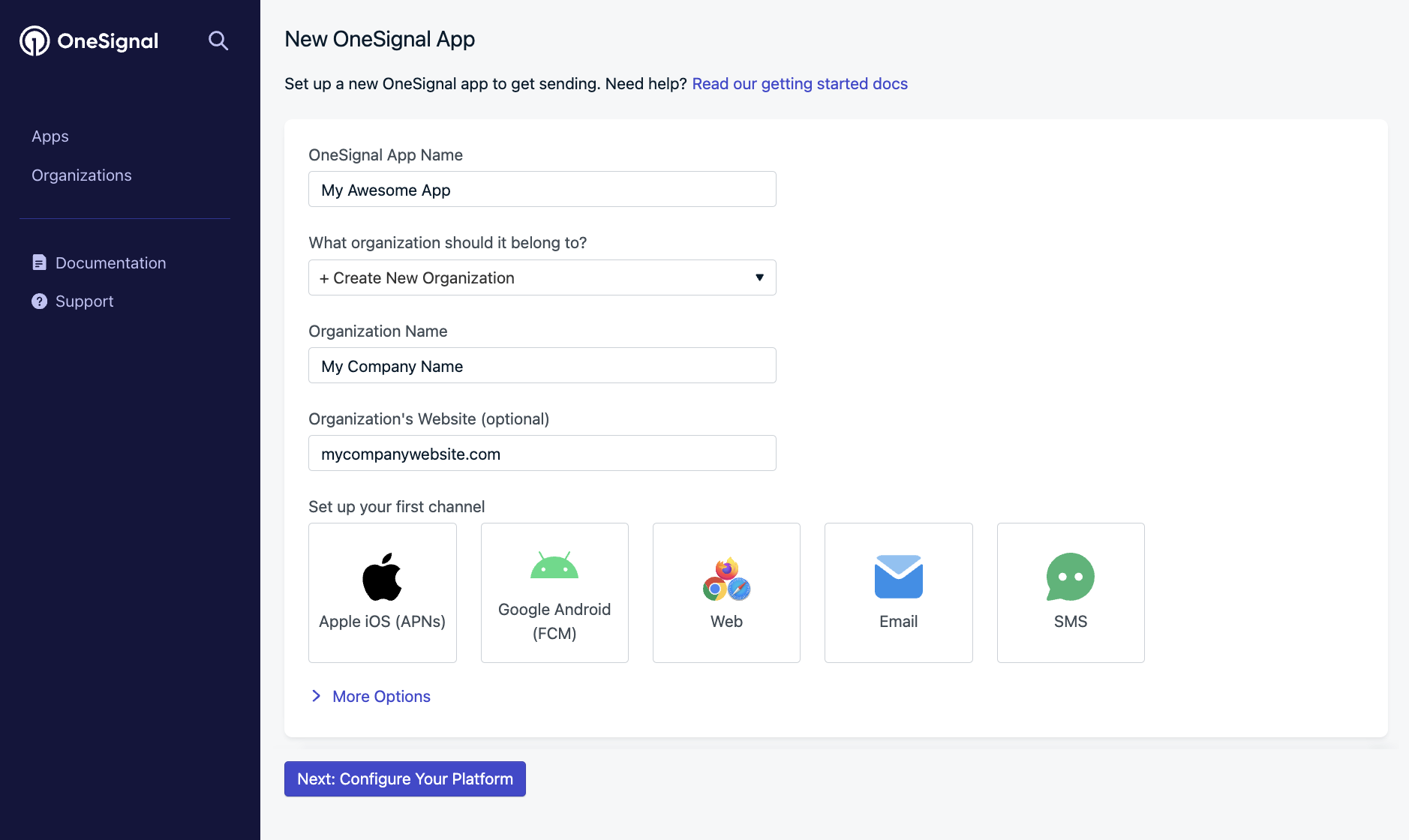
To configure your app, follow the prompts based on the platforms you support.
- Android: Set up your Android Firebase Credentials
- iOS: p8 Authentication Token or p12 Push Notification Certificate
- Amazon: Generate an Amazon API Key
- Huawei: Authorize OneSignal to send Huawei Push
After you setup your credentials, click Save & Continue.
Choose your Apps Target SDK, the click Save & Continue.
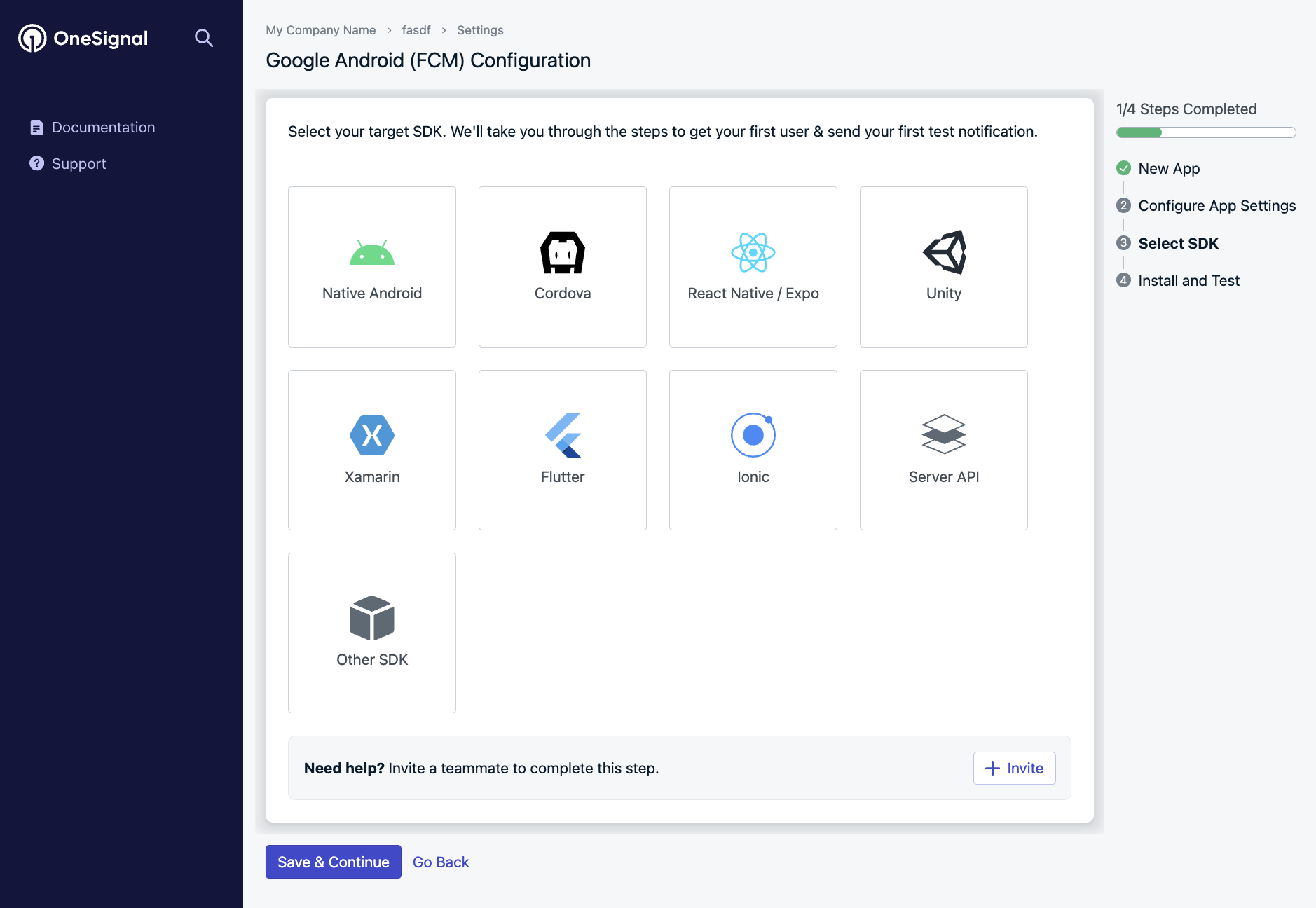
Finally, you will be directed to install the SDK and provided your OneSignal App ID. Make sure to save your App ID as you will need it later.
If you need a teammate or your developer to assist, you can click Invite them to the app and select Done when finished.
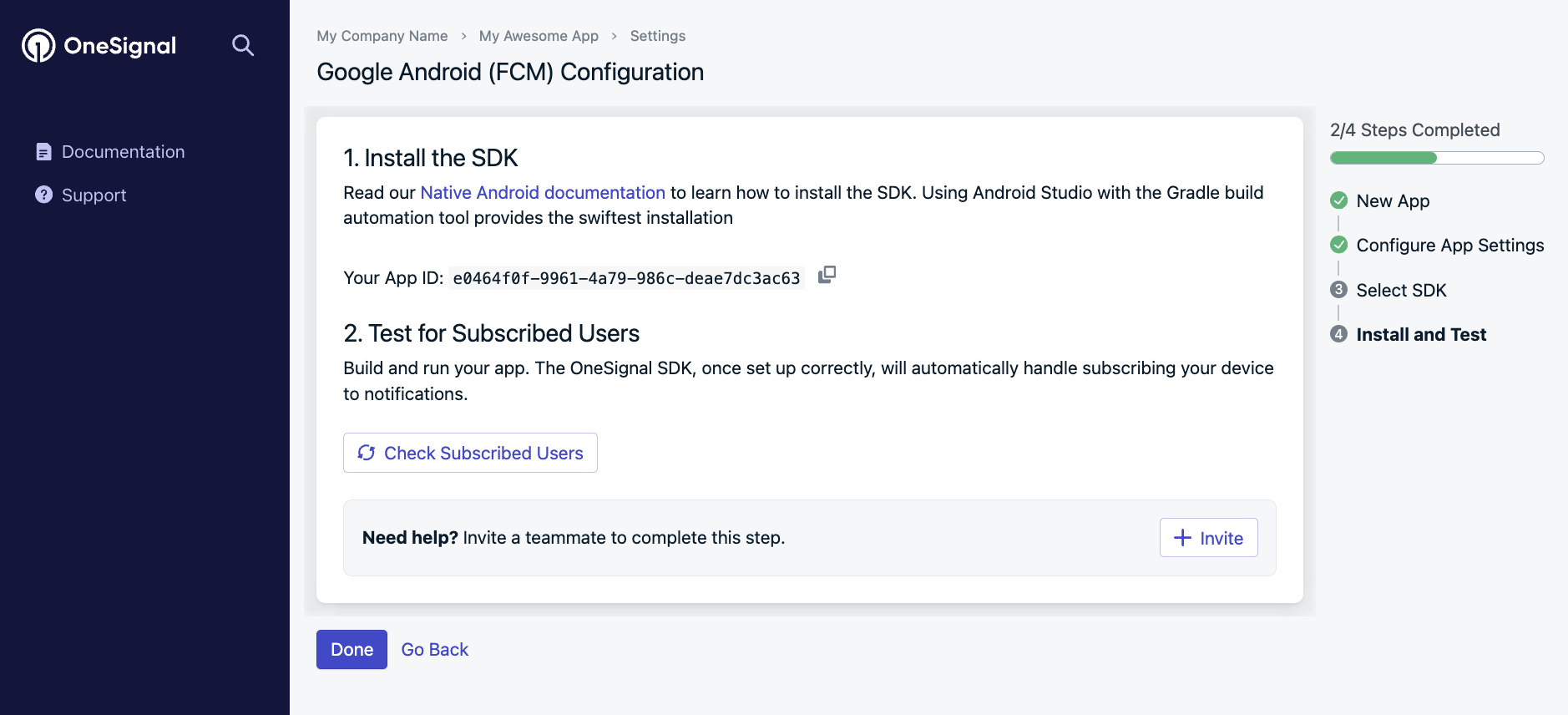
Continue through the documentation to finish adding OneSignal to your app.
Setup
1. Add SDK
Add the react-native-onesignal
package to your project.
- Yarn:
yarn add react-native-onesignal
- npm
npm install --save react-native-onesignal
If using React Native version higher than0.60
skip to step 2 because Autolinking is now done automatically.
If using React Native 0.60
or lower, run: react-native link react-native-onesignal
2. iOS setup
Open the .xcworkspace
file in Xcode located your project's ios folder.
Select the root project > your main app target > Signing & Capabilities.
If you do not see Push Notifications enabled, click + Capability and add Push Notifications.
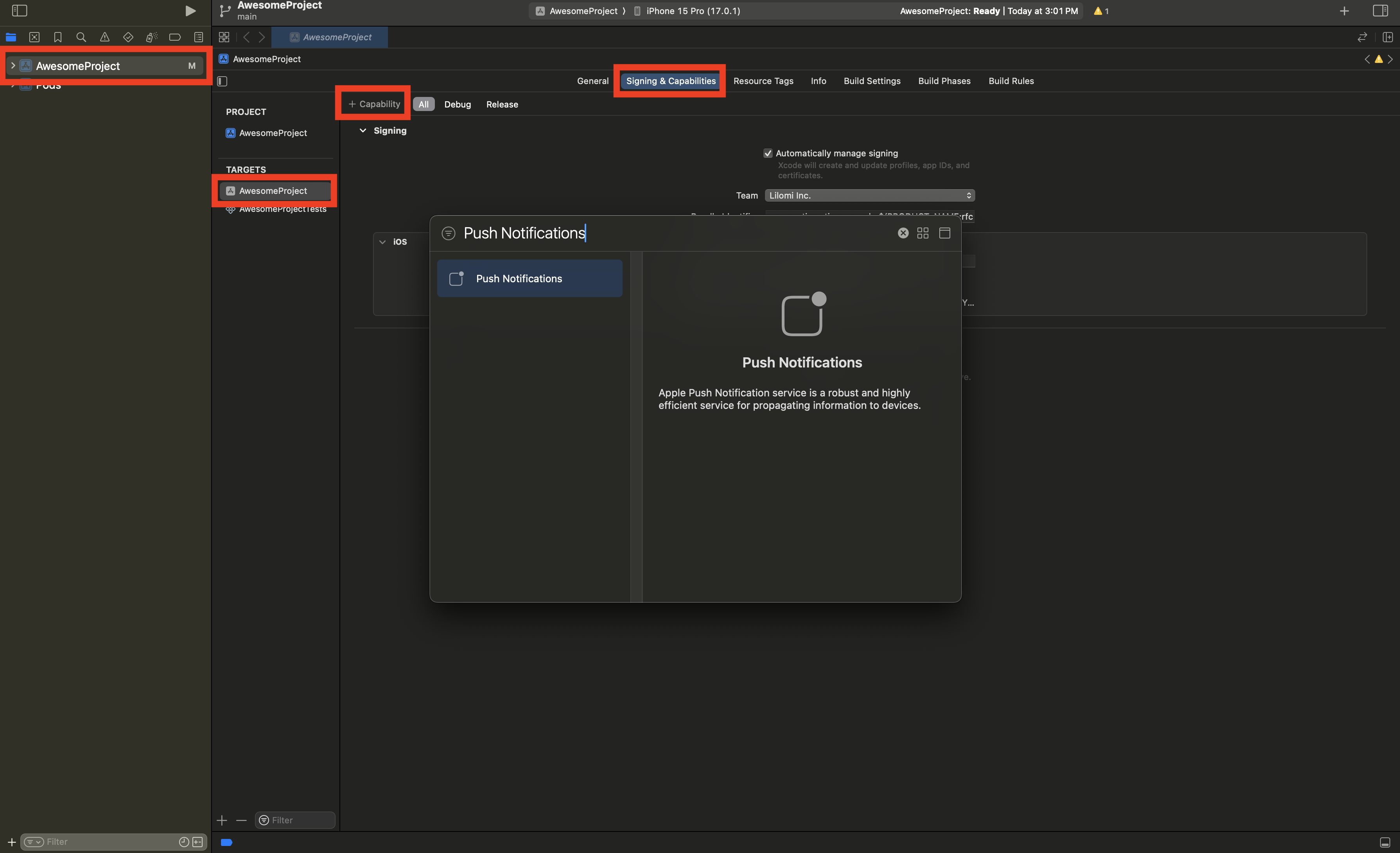
Click + Capability again and add Background Modes. Then check Remote notifications.
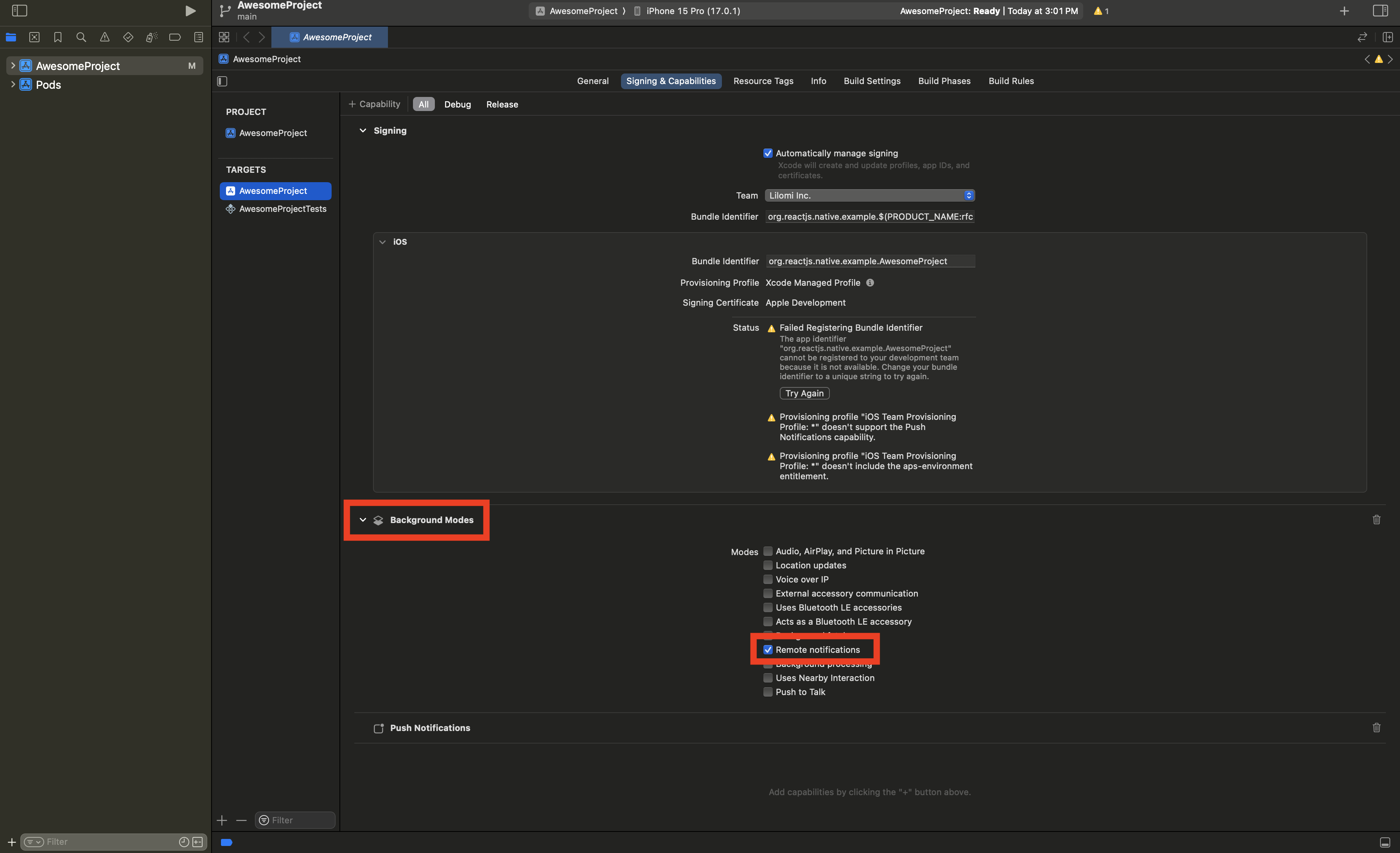
Add Notification Service Extension
Expo setup
If you are using the OneSignal Expo Plugin, the Notification Service Extension should be added automatically. Please follow the set up guide for Expo here.
The OneSignalNotificationServiceExtension allows your iOS application to receive rich notifications with images, buttons, and badges. It's also required for OneSignal's Confirmed Delivery analytics features.
In Xcode Select File > New > Target...
Select Notification Service Extension then Next.
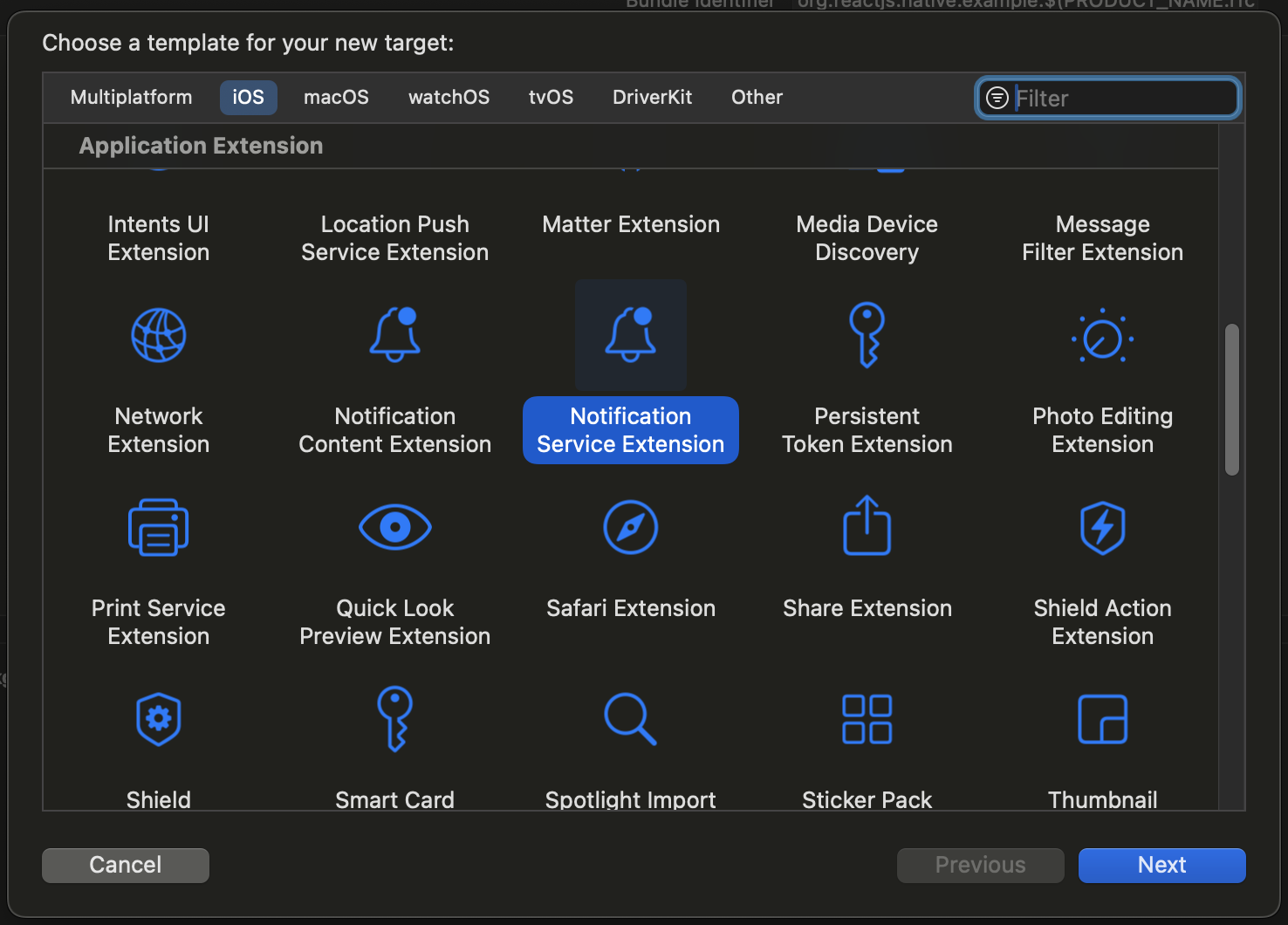
Enter the product name as OneSignalNotificationServiceExtension
and press Finish.
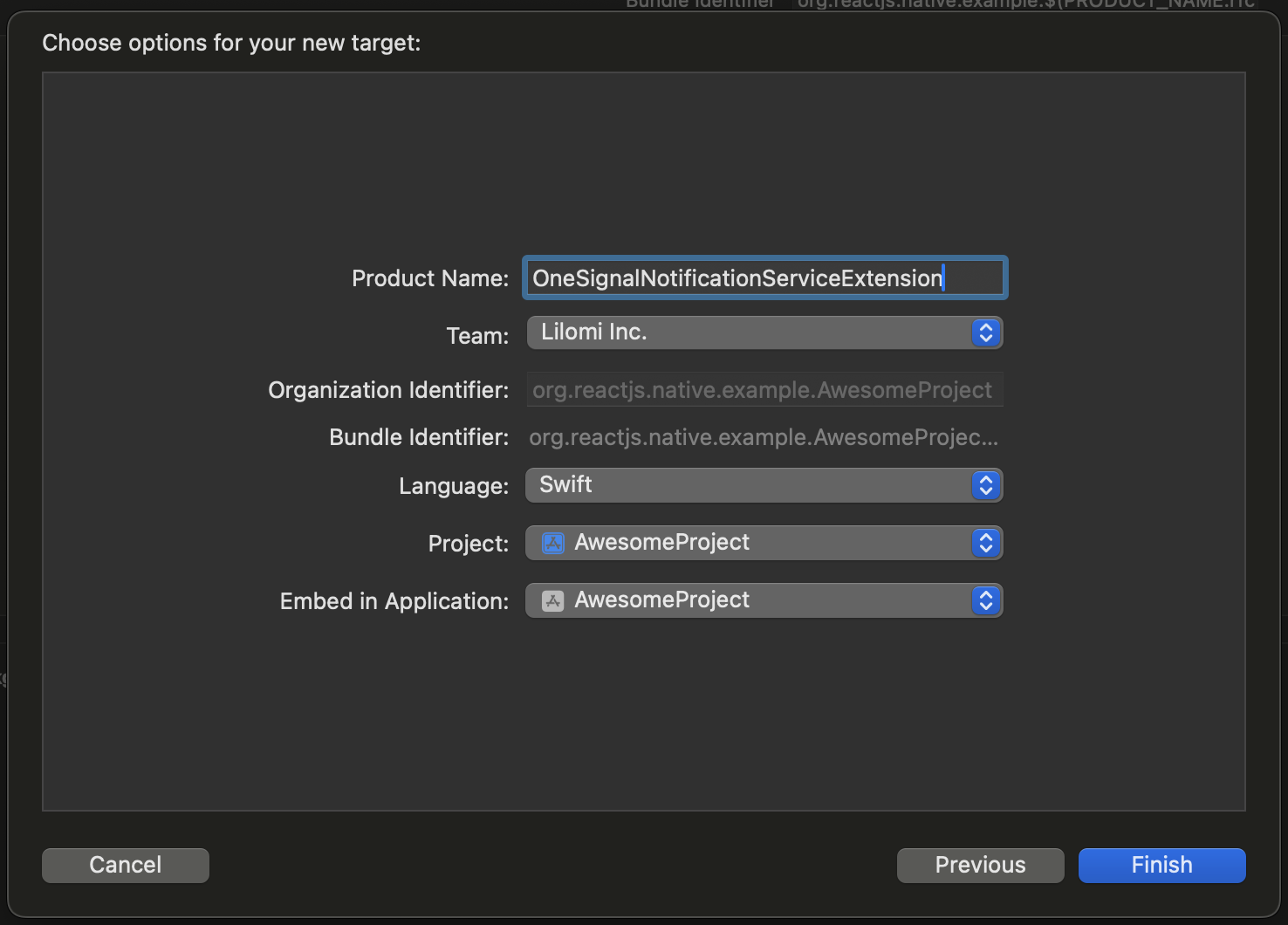
Do not activate the scheme on the dialog that is shown after selecting "Finish".
Press Cancel on the "Activate" scheme prompt.
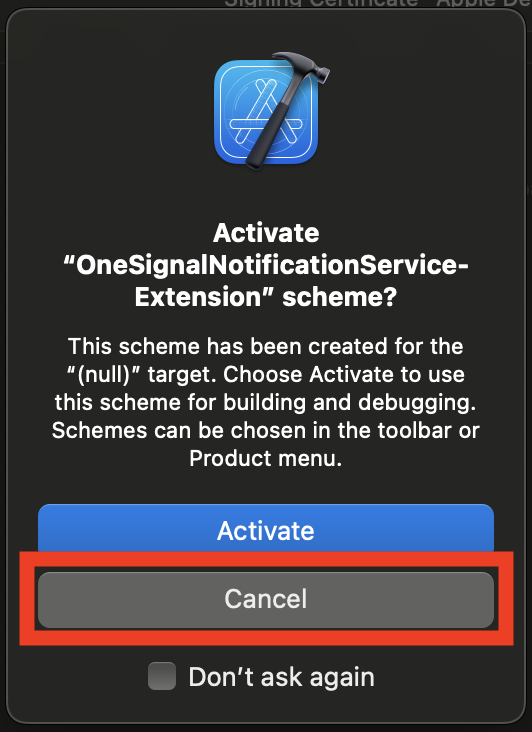
By canceling, you keep Xcode debugging your app instead of the extension you just created. If you activated by accident, you can switch back to debug your app target (middle-top next to the device selector).
Select the OneSignalNotificationServiceExtension target and General settings.
Set Minimum Deployments to be the same value as your Main Application Target. This should be iOS 14.5 or higher.
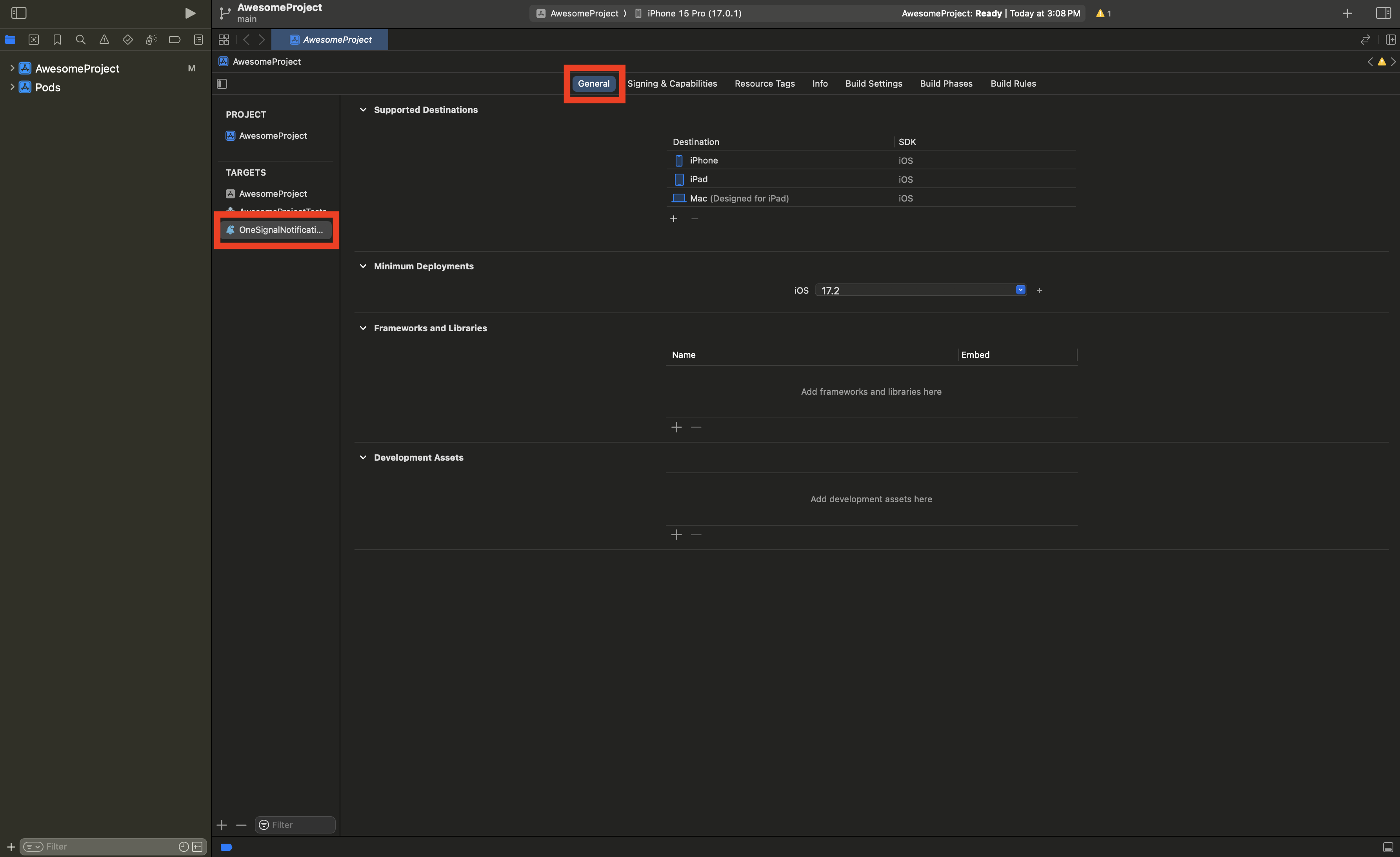
Set the OneSignalNotificationServiceExtension Target Minimum Deployments value to be the same as your Main Application Target.
Add App Groups
App Groups allow your app and the OneSignalNotificationServiceExtension to communicate when a notification is received, even if your app is not active. This is required for badges and Confirmed Deliveries.
Select your Main App Target > Signing & Capabilities > + Capability > App Groups.
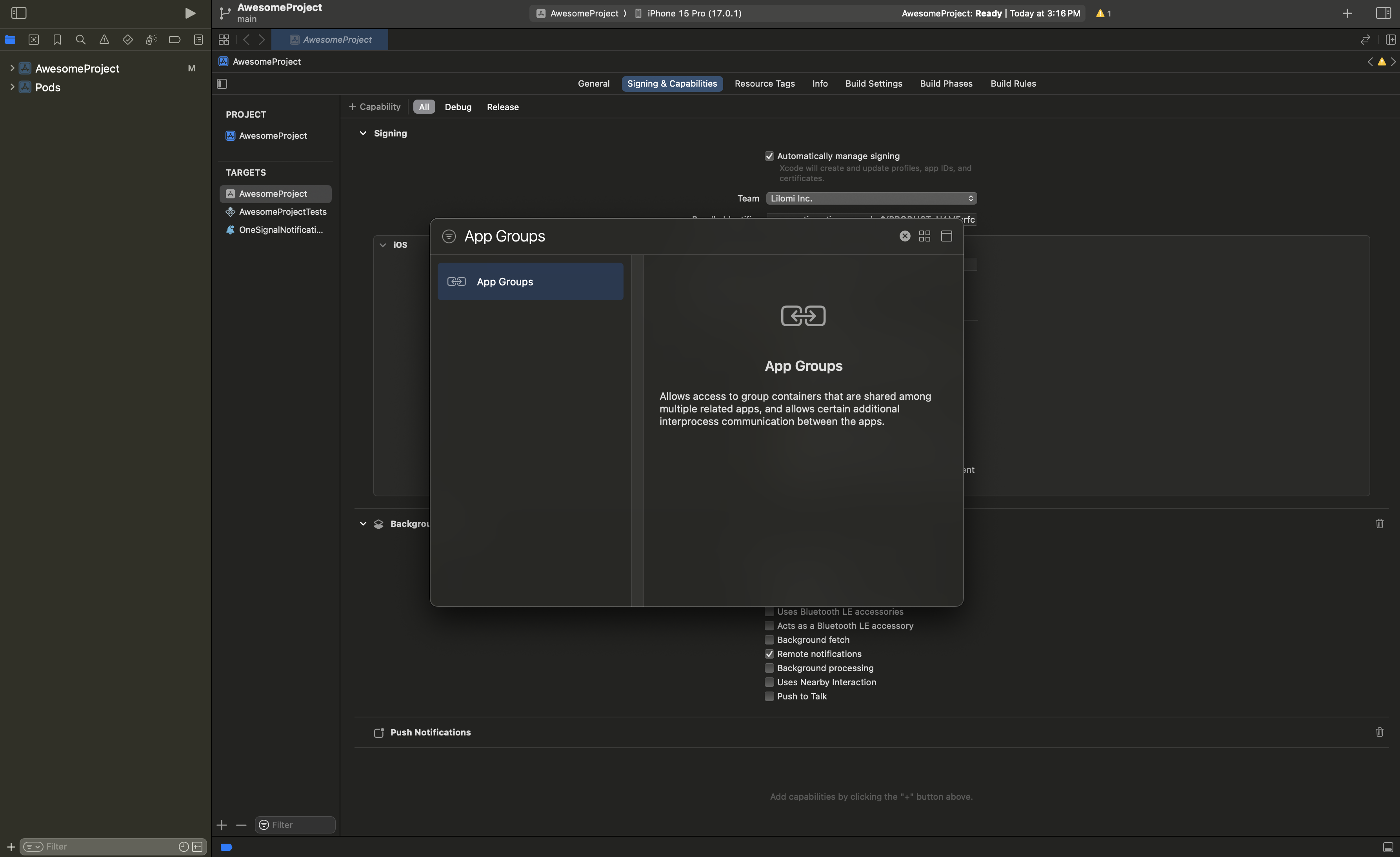
Within App Groups, click the + button.
Set the App Groups container to be group.YOUR_BUNDLE_IDENTIFIER.onesignal
where YOUR_BUNDLE_IDENTIFIER
is the same as your Main Application "Bundle Identifier".
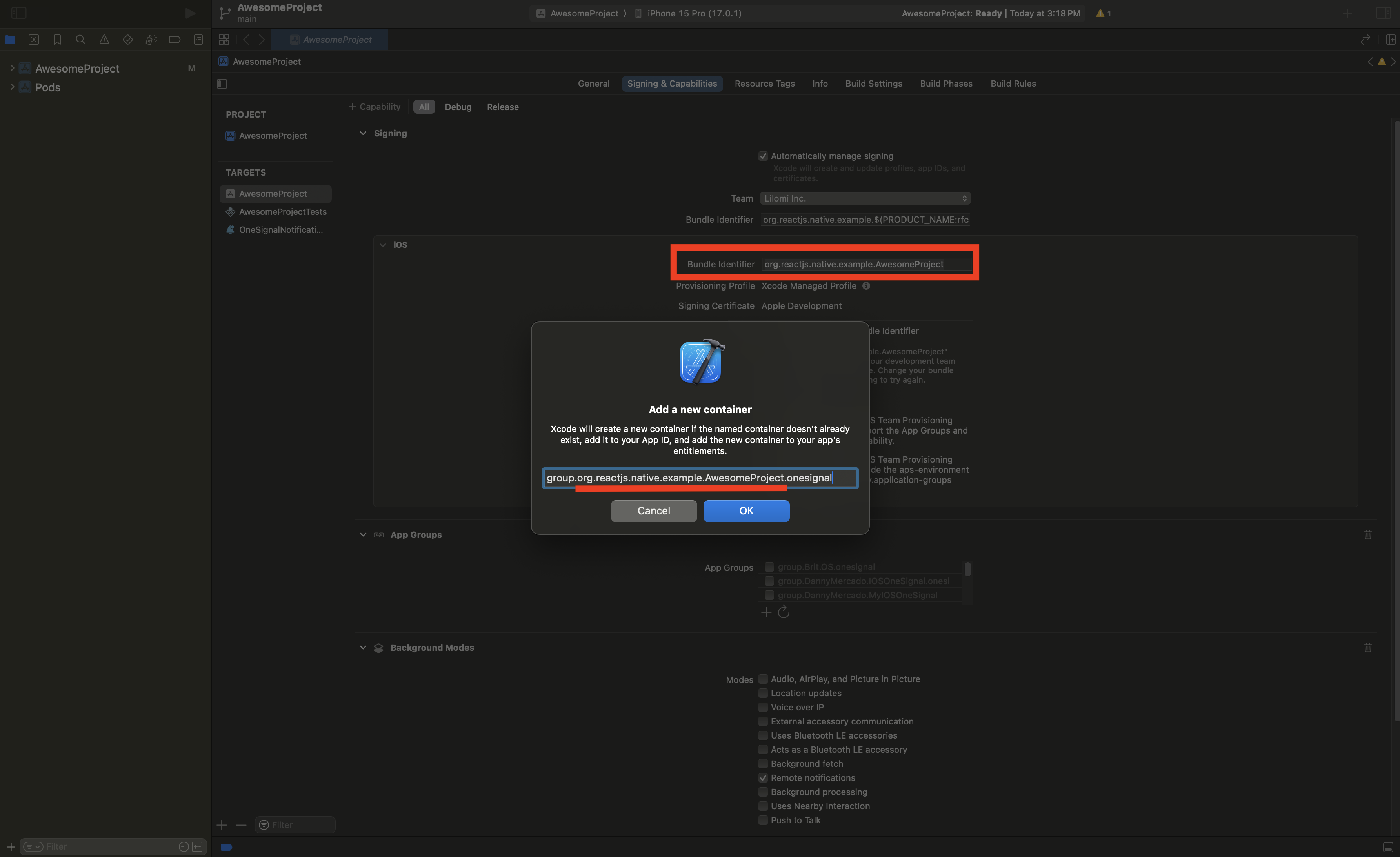
Press OK and repeat for the OneSignalNotificationServiceExtension Target.
Select the OneSignalNotificationServiceExtension Target > Signing & Capabilities > + Capability > App Groups.
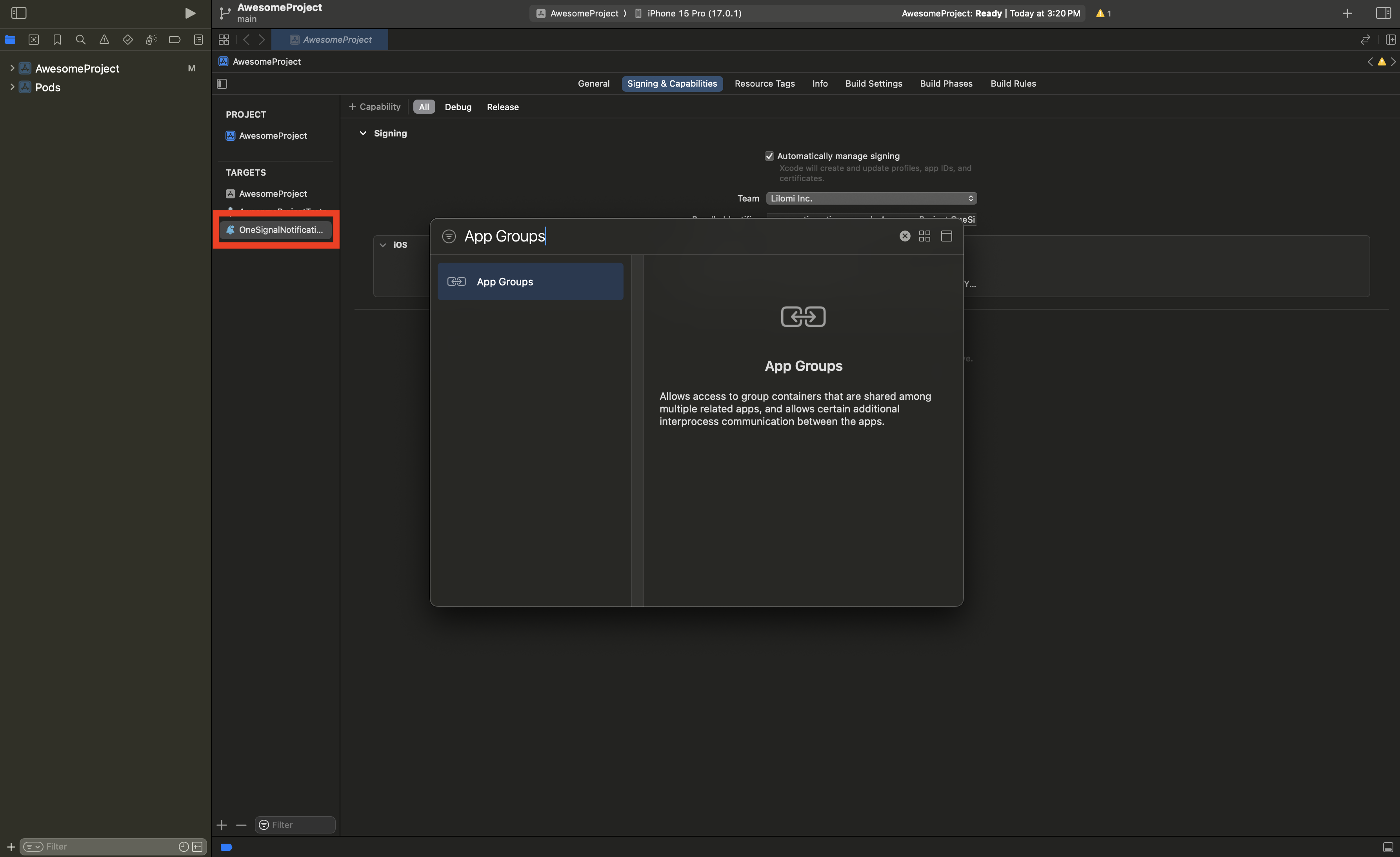
Within App Groups, click the + button.
Set the App Groups container to be group.YOUR_BUNDLE_IDENTIFIER.onesignal
where YOUR_BUNDLE_IDENTIFIER
is the same as your Main Application "Bundle Identifier".
DO NOT INCLUDE OneSignalNotificationServiceExtension
.
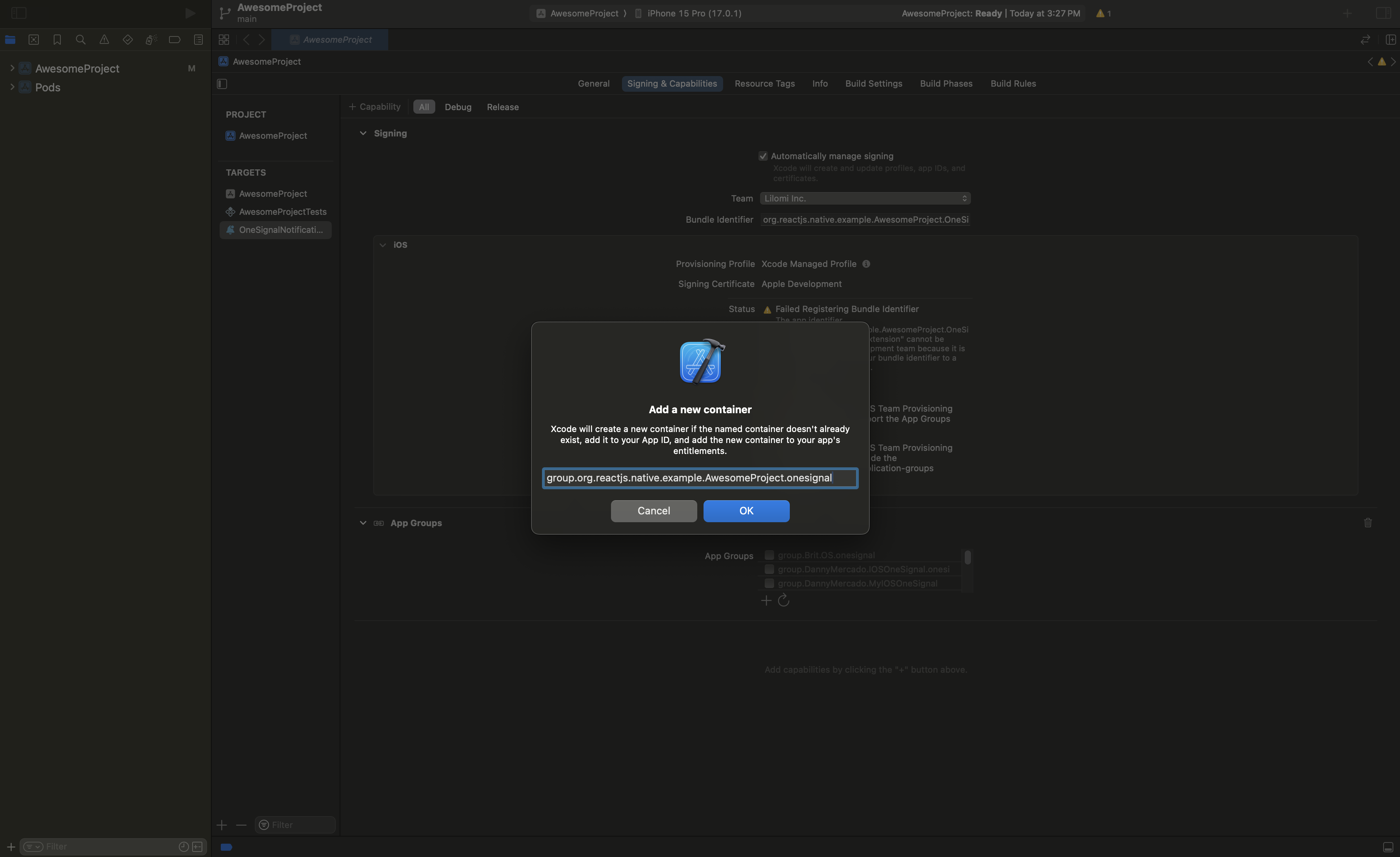
Do not include OneSignalNotificationServiceExtension
Optional instructions to setup custom App Group Name (click to expand)
This step is only required if you do not want to use the default app group name (which is group.{your_bundle_id}.onesignal
).
Open your Info.plist
file and add a new OneSignal_app_groups_key
as a String
type.
Enter the group name you checked in the last step as it's value.
Make sure to do the same for the Info.plist
under the OneSignalNotificationServiceExtension
folder.
Add OneSignal SDK to the OneSignalNotificationServiceExtension
In the /ios
directory of your project, open the Podfile
and add the following outside of the main target (should be at the same level as your main target):
target 'OneSignalNotificationServiceExtension' do
pod 'OneSignalXCFramework', '>= 5.0.0', '< 6.0'
end
# Resolve react_native_pods.rb with node to allow for hoisting
require Pod::Executable.execute_command('node', ['-p',
'require.resolve(
"react-native/scripts/react_native_pods.rb",
{paths: [process.argv[1]]},
)', __dir__]).strip
platform :ios, min_ios_version_supported
prepare_react_native_project!
# If you are using a `react-native-flipper` your iOS build will fail when `NO_FLIPPER=1` is set.
# because `react-native-flipper` depends on (FlipperKit,...) that will be excluded
#
# To fix this you can also exclude `react-native-flipper` using a `react-native.config.js`
# ```js
# module.exports = {
# dependencies: {
# ...(process.env.NO_FLIPPER ? { 'react-native-flipper': { platforms: { ios: null } } } : {}),
# ```
flipper_config = ENV['NO_FLIPPER'] == "1" ? FlipperConfiguration.disabled : FlipperConfiguration.enabled
linkage = ENV['USE_FRAMEWORKS']
if linkage != nil
Pod::UI.puts "Configuring Pod with #{linkage}ally linked Frameworks".green
use_frameworks! :linkage => linkage.to_sym
end
target 'AwesomeProject' do
config = use_native_modules!
use_react_native!(
:path => config[:reactNativePath],
# Enables Flipper.
#
# Note that if you have use_frameworks! enabled, Flipper will not work and
# you should disable the next line.
:flipper_configuration => flipper_config,
# An absolute path to your application root.
:app_path => "#{Pod::Config.instance.installation_root}/.."
)
target 'AwesomeProjectTests' do
inherit! :complete
# Pods for testing
end
post_install do |installer|
# https://github.com/facebook/react-native/blob/main/packages/react-native/scripts/react_native_pods.rb#L197-L202
react_native_post_install(
installer,
config[:reactNativePath],
:mac_catalyst_enabled => false
)
end
end
target 'OneSignalNotificationServiceExtension' do
pod 'OneSignalXCFramework', '>= 5.0.0', '< 6.0'
end
From your terminal, navigate to this ios
folder and run pod install --repo-update
In the Xcode project navigator, select the OneSignalNotificationServiceExtension folder and open the NotificationService.m
or NotificationService.swift
file.
Replace the whole file's contents with the following code.
import UserNotifications
import OneSignalExtension
class NotificationService: UNNotificationServiceExtension {
var contentHandler: ((UNNotificationContent) -> Void)?
var receivedRequest: UNNotificationRequest!
var bestAttemptContent: UNMutableNotificationContent?
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.receivedRequest = request
self.contentHandler = contentHandler
self.bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
if let bestAttemptContent = bestAttemptContent {
/* DEBUGGING: Uncomment the 2 lines below to check this extension is executing
Note, this extension only runs when mutable-content is set
Setting an attachment or action buttons automatically adds this */
// print("Running NotificationServiceExtension")
// bestAttemptContent.body = "[Modified] " + bestAttemptContent.body
OneSignalExtension.didReceiveNotificationExtensionRequest(self.receivedRequest, with: bestAttemptContent, withContentHandler: self.contentHandler)
}
}
override func serviceExtensionTimeWillExpire() {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
if let contentHandler = contentHandler, let bestAttemptContent = bestAttemptContent {
OneSignalExtension.serviceExtensionTimeWillExpireRequest(self.receivedRequest, with: self.bestAttemptContent)
contentHandler(bestAttemptContent)
}
}
}
#import <OneSignalExtension/OneSignalExtension.h>
#import "NotificationService.h"
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNNotificationRequest *receivedRequest;
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@end
@implementation NotificationService
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
self.receivedRequest = request;
self.contentHandler = contentHandler;
self.bestAttemptContent = [request.content mutableCopy];
/* DEBUGGING: Uncomment the 2 lines below and comment out the one above to ensure this extension is executing
Note, this extension only runs when mutable-content is set
Setting an attachment or action buttons automatically adds this */
// NSLog(@"Running NotificationServiceExtension");
// self.bestAttemptContent.body = [@"[Modified] " stringByAppendingString:self.bestAttemptContent.body];
[OneSignalExtension didReceiveNotificationExtensionRequest:self.receivedRequest
withMutableNotificationContent:self.bestAttemptContent
withContentHandler:self.contentHandler];
}
- (void)serviceExtensionTimeWillExpire {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
[OneSignalExtension serviceExtensionTimeWillExpireRequest:self.receivedRequest withMutableNotificationContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
}
@end
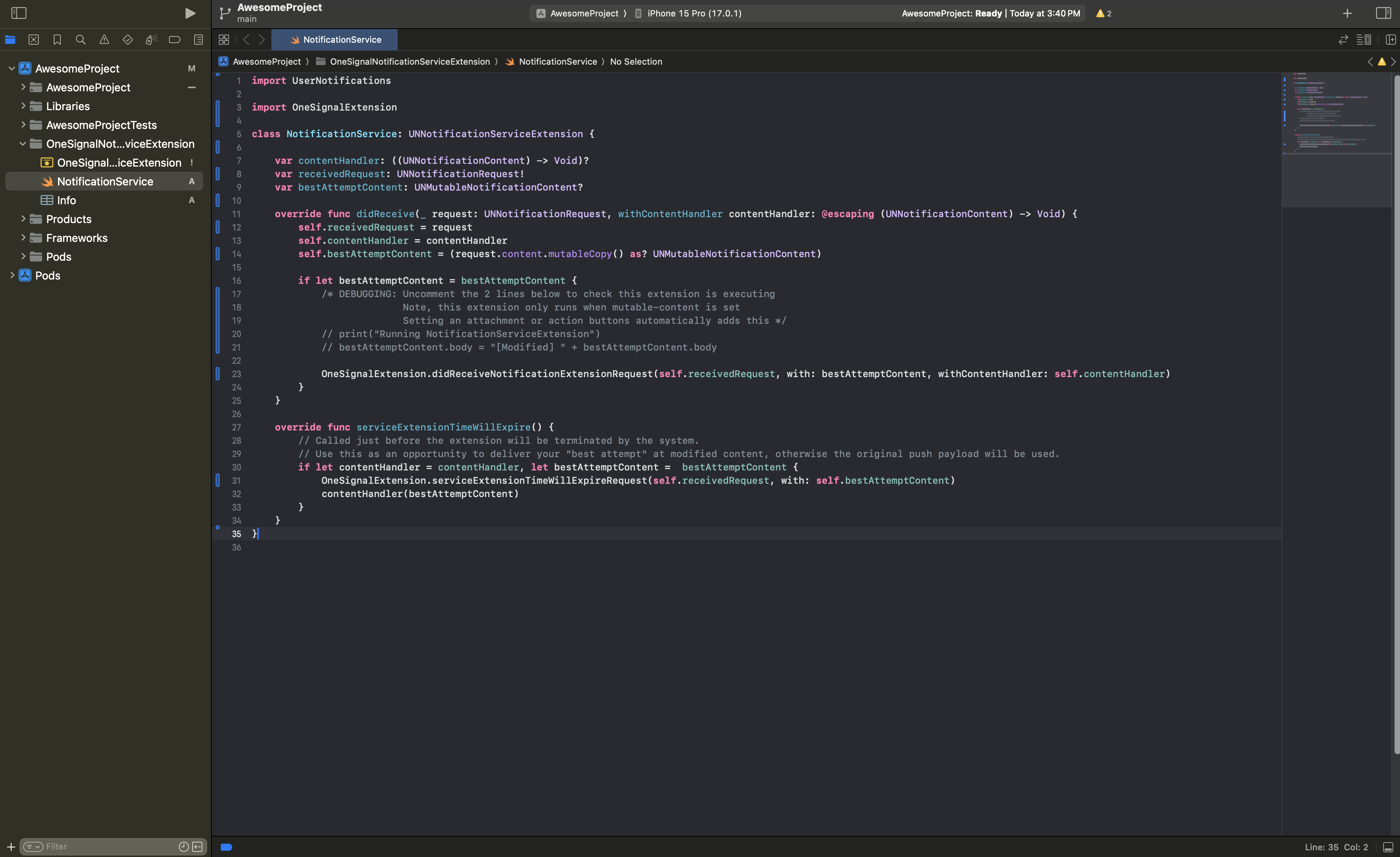
Example of the NotificationService.swift file.
3. Android setup
Ensure that you've followed this guide to set up your [Android Firebase Credentials](https://documentation.onesignal.com/docs/android-firebase-credentials).
By default, notifications will be shown with a small bell icon in the notification shade. Follow the Customize Notification Icons guide to create your own small and large notification icons for your app.
4. Initialization
In your App.js
, App.tsx
or index.js
initialize OneSignal and try the example methods below:
import { LogLevel, OneSignal } from 'react-native-onesignal';
// Add OneSignal within your App's root component
const App = () => {
// Remove this method to stop OneSignal Debugging
OneSignal.Debug.setLogLevel(LogLevel.Verbose);
// OneSignal Initialization
OneSignal.initialize("ONESIGNAL_APP_ID");
// requestPermission will show the native iOS or Android notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission
OneSignal.Notifications.requestPermission(true);
// Method for listening for notification clicks
OneSignal.Notifications.addEventListener('click', (event) => {
console.log('OneSignal: notification clicked:', event);
});
}
Event Listeners & Components
We suggest using a base/root component to add as an event listener. If you choose a sub-component that is only shown in some situations (such as using a homepage as an event listener), the component may unmount later on as the user navigates elsewhere in your app.
If you encounter problems with one or more of the events listeners, please see our troubleshooting documentation here.
PBXGroup Error
You may see this error when building with Xcode 16.
RuntimeError -
PBXGroup
attempted to initialize an object with unknown ISAPBXFileSystemSynchronizedRootGroup
from attributes:{"isa"=>"...", "exceptions"=>["//", "..."], "explicitFileTypes"=>{}, "explicitFolders"=>[], "path"=>"OneSignalNotificationServiceExtension", "sourceTree"=>"<group>"}
Check the error for the folder name under "path"
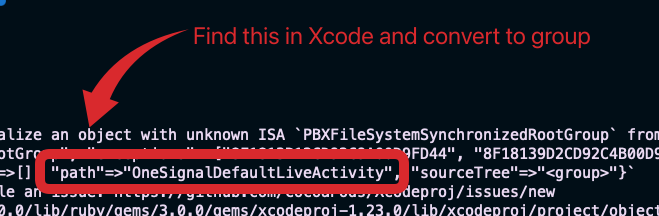
Then in your project, open your XCWorkspace folder in Xcode and open the project directory in the left sidebar.
From here, you should see the folders corresponding with the the targets you have available on this project. You should find folder that matches the "path" in the error you've received. Update the folder to "Convert to Group".
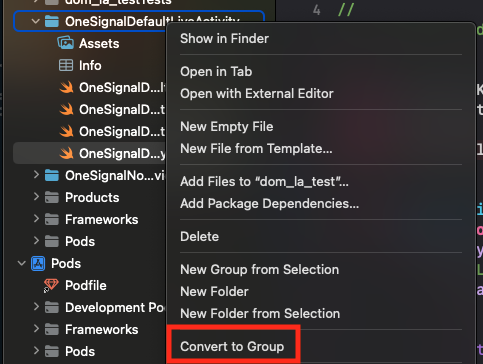
Cycle Inside Error
You may see this error when building with Xcode 15 or higher, which is caused by a Xcode 15+ default configuration change affecting cross platform systems.
Error: Cycle inside SampleProject; building could produce unreliable results.
In your project, open your XCWorkspace folder in Xcode and open the project directory in the left sidebar.
Select your app target and navigate to the "Build Phases" tab. You should have a phase called "Embed App Extensions" or "Embed Foundation Extensions". Drag and move this build phase to above "Run Script". Your error should now be resolved.
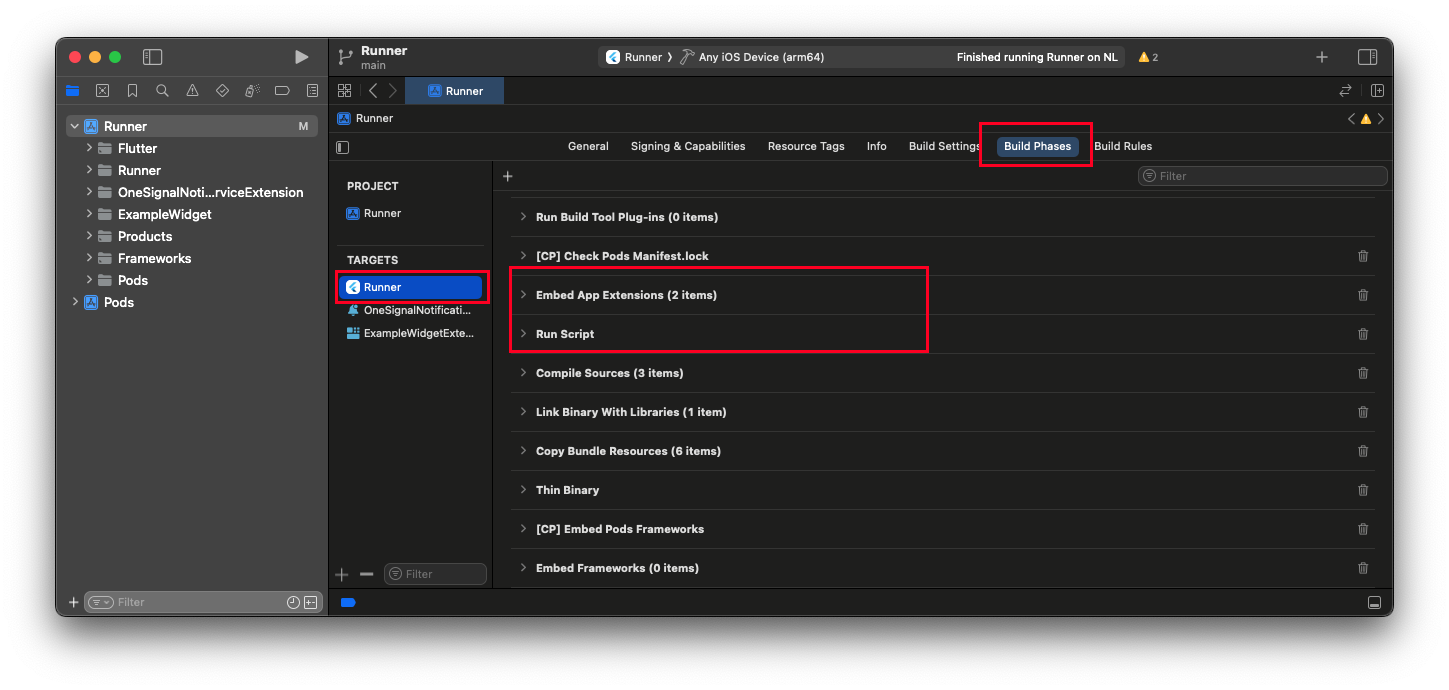
If that does not fix the error, you may need to un-check "Copy only when installing". This option can be found when you expand the build phase that you just moved.
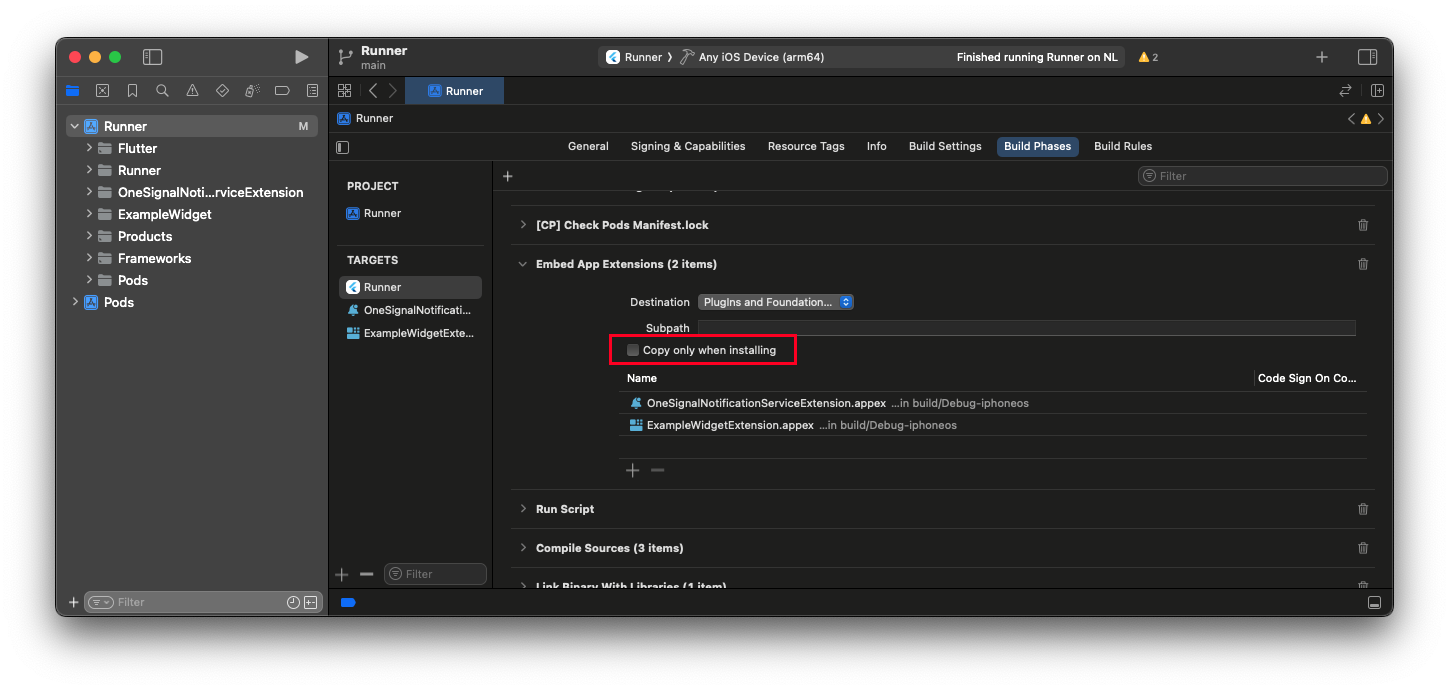
5. Testing
Run your app on a physical device to make sure it builds correctly.
If you used the provided code, then the requestPermission
method, should prompt you to subscribe to push notifications. You can always change this later but for now, click "Allow" to subscribe to push notifications.
Check your OneSignal Dashboard Audience > Subscriptions to see your Subscription and click the options button on the left to set yourself as a Test Subscription.
Troubleshooting
If running into issues, see our Mobile Troubleshooting Guide.
Try our example projects on our Github repository.
If stuck, contact support directly or email support@onesignal.com for help.
For faster assistance, please provide:
- Your OneSignal App ID
- Details, logs, and/or screenshots of the issue
- Steps to reproduce
Users & subscriptions
Required if using integrations.
Recommended for messaging across multiple channels (push, email, sms).
If you need user consent before tracking their data. OneSignal provides user consent methods to help delay initialization of our SDK until consent is provided. See Handling Personal Data for details.
External ID & aliases
When a user downloads and opens your mobile app or uninstalls and re-installs your app on the same device, a Subscription and a User is created within the OneSignal app.
You can identify that user across multiple subscriptions by setting the External ID property. The External ID should be distinct user ID representing a single user. We recommend using the same user ID as in your Integrations or main analytics tool.
When you authenticate users in your app, call our login
method at any time to link this subscription to a user.
let externalId = "123456789" // You will supply the external id to the OneSignal SDK
OneSignal.login(externalId);
Our mobile SDKs have methods for detecting User state changes that might be helpful for internal tracking.
External ID & custom aliases
If your users have multiple user IDs, we do support additional custom Aliases. However, you should still always set the External ID as this is the main alias we use to identify users. See Users for details.
Add email and phone number subscriptions
Recommended if using email and SMS messaging.
Like push subscriptions, email addresses and phone numbers each are a new subscription within OneSignal. Your email and sms subscriptions will be tied to the same user if created using our SDK or if you setting the External ID. You can Import your current user data and use our APIs and/or SDK methods to capture new email addresses and phone numbers when provided to you by your users.
// Pass in email provided by customer
OneSignal.User.addEmail("example@domain.com");
// Pass in phone number provided by customer
OneSignal.User.addSms("+11234567890");
Users & subscriptions
See Users and Subscriptions for more details.
Property & event tags
Tags are custom key : value
pairs of String data used for tracking any custom user events and properties. Setting tags is required for more complex Segments and Message Personalization.
For event triggered messages, use tags with Time Operators to note the date and time the event is/occurred and setup automations for sending to those users. See Abandoned Cart Example for details.
OneSignal.User.addTag("key", "value");
Data tags
If you want to store custom data within OneSignal for segmentation, message personalization, and event triggered automation, see Tags for details.
Importing users and subscriptions
If you have a list of user data from a previous source, you can import it into OneSignal following our Import guides.
Message configuration
Common setup items to get the most of your integration.
Deep linking
See our Deep Linking guide to set those up for push and other messaging channels.
Basic SDK setup complete!
For details on the above methods and other methods available, see our Mobile SDK reference.
Updated 3 days ago