Ionic & Capacitor SDK Setup
Instructions for adding the OneSignal SDK to your Ionic or Capacitor app for iOS, Android, Amazon Fire apps
Looking for a strictly Cordova setup?
See our Cordova SDK Setup guide instead.
Step 1. Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs
- Ionic 1 or newer
- Cordova 9.1.0 or newer
- Capacitor 2.0.0 or newer
iOS Requirements
- iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- mac with Xcode 12+
- p8 Authentication Token or p12 Push Notification Certificate
- Cordova [email protected] or newer
- CocoaPods version 1.12.1+ Install with the following from the Terminal
sudo gem install cocoapods
pod repo update
Android Requirements
- Android 4.1+ device or emulator with "Google Play Store (Services)" installed
- Set up your Google/Firebase keys in OneSignal
- Project using AndroidX
- Cordova [email protected] or newer
Amazon & Huawei Requirements
Follow these instructions if your app is distributed on the Amazon AppStore and/or the Huawei AppGallery.
Step 2. Import OneSignal Plugin
You should remove any other push SDKs that you are not using – otherwise, you may see duplicate notifications being sent to your users.
Run the following from your project directory.
npm install onesignal-cordova-plugin
npx cap sync
ionic cordova plugin add onesignal-cordova-plugin --save
ionic plugin add onesignal-cordova-plugin --save
Step 3. Add Required Code
When creating a new Ionic + Capacitor/Cordova project inside of the Ionic Dashboard or using the CLI, you get the option to choose what kind of project you want to create.
Option A: Ionic + Capacitor (Angular)
Add the following to your src/app/app.component.ts
.
OneSignalInit();
// Add the following to your existing ready fuction.
constructor(platform: Platform, statusBar: StatusBar, splashScreen: SplashScreen) {
platform.ready().then(() => {
statusBar.styleDefault();
splashScreen.hide();
OneSignalInit();
}
}
// Add the following to your existing ready fuction.
.run(function($ionicPlatform) {
$ionicPlatform.ready(function() {
//... keep any existing code
OneSignalInit();
}
}
Option B: Ionic + Capacitor (React)
Add the following to your src/App.tsx
or src/index.tsx
.
OneSignalInit();
Option C: Ionic + Capacitor (Vue)
Add the following to your src/main.tsx
.
document.addEventListener("deviceready", OneSignalInit, false);
Option D: Ionic + Cordova (Angular)
Add the following to your src/app/app.component.ts
.
constructor(platform: Platform) {
platform.ready().then(() => {
OneSignalInit();
});
}
3.2 Add the OneSignalInit
Function
Add the OneSignalInit()
code block to the same file as noted above.
import OneSignal from 'onesignal-cordova-plugin';
// Call this function when your app starts
function OneSignalInit(): void {
// Uncomment to set OneSignal device logging to VERBOSE
// OneSignal.setLogLevel(6, 0);
// NOTE: Update the setAppId value below with your OneSignal AppId.
OneSignal.setAppId("YOUR_ONESIGNAL_APP_ID");
OneSignal.setNotificationOpenedHandler(function(jsonData) {
console.log('notificationOpenedCallback: ' + JSON.stringify(jsonData));
});
// Prompts the user for notification permissions.
// * Since this shows a generic native prompt, we recommend instead using an In-App Message to prompt for notification permission (See step 7) to better communicate to your users what notifications they will get.
OneSignal.promptForPushNotificationsWithUserResponse(function(accepted) {
console.log("User accepted notifications: " + accepted);
});
}
// Call this function when your app start
function OneSignalInit() {
// Uncomment to set OneSignal device logging to VERBOSE
// window.plugins.OneSignal.setLogLevel(6, 0);
// NOTE: Update the setAppId value below with your OneSignal AppId.
window["plugins"].OneSignal.setAppId("YOUR_ONESIGNAL_APP_ID");
window["plugins"].OneSignal.setNotificationOpenedHandler(function(jsonData) {
console.log('notificationOpenedCallback: ' + JSON.stringify(jsonData));
});
// iOS - Prompts the user for notification permissions.
// * Since this shows a generic native prompt, we recommend instead using an In-App Message to prompt for notification permission (See step 6) to better communicate to your users what notifications they will get.
window["plugins"].OneSignal.promptForPushNotificationsWithUserResponse(function(accepted) {
console.log("User accepted notifications: " + accepted);
});
}
3.3 Replace YOUR_ONESIGNAL_APP_ID
with your OneSignal AppId, available in Settings > Keys & IDs.
Step 4. Update Project Settings to Target Android 13 (Android Only)
Validate your target SDK version is at least version 33.
Requirements:
- Ionic Capacitor v2 or higher
android {
compileSdkVersion 33
...
defaultConfig {
...
targetSdkVersion 33
}
}
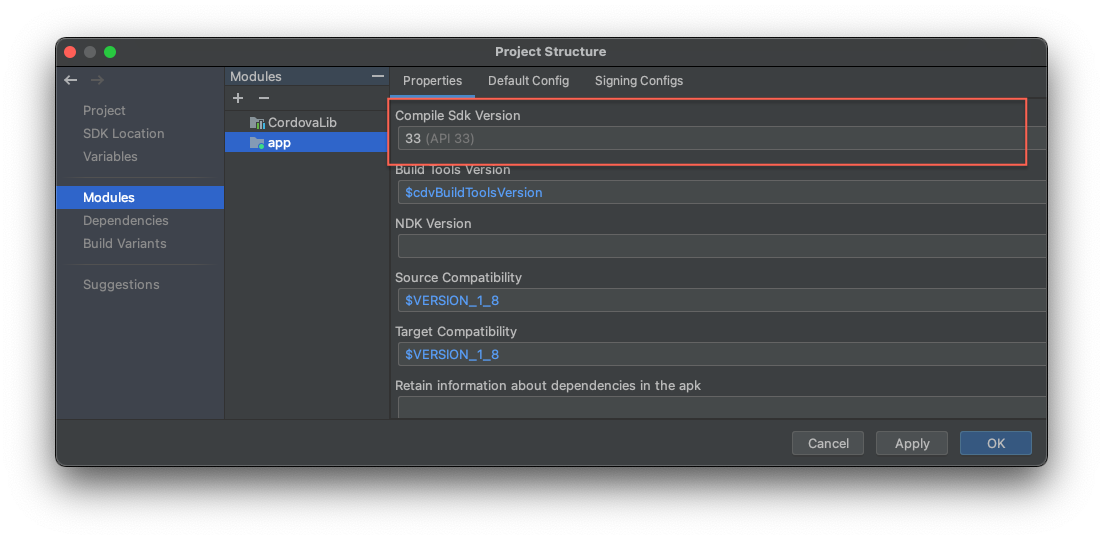
Set the Compile Sdk Version to 33.
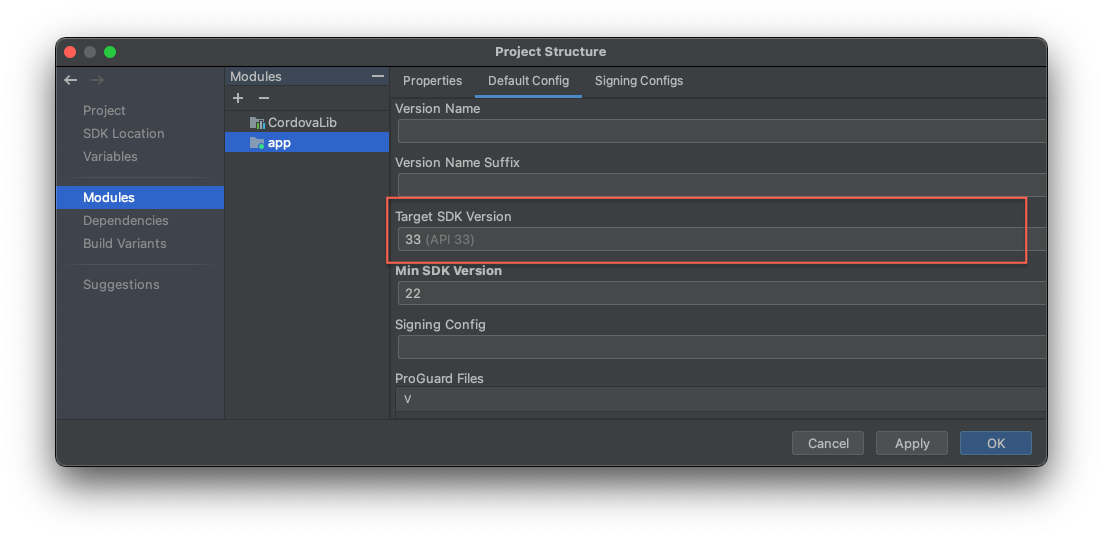
In Default Config, set the Target SDK Version to 33.
Step 5. Configure your Xcode project (iOS Only)
5.1 In /platforms/ios
open the .wcworkspace
project in Xcode.
- Run
xed platforms/ios
from your project directory to do this.
5.2 Select the root project, and under Signing & Capabilities
, enable Push Notifications
.
5.3 Next, enable Background Modes
and check Remote Notifications
.
iOS Service Extensions
Highly recommended: This step is optional but highly recommended. The OneSignalNotificationServiceExtension
allows your application (in iOS) to receive rich notifications with images and/or buttons, along with Badges and advanced analytics like Outcomes.
5.4 Use Objective-C in this step, then come back to this page and continue following the steps below. Please follow the Notification Service Extension guide (only follow Steps 2 in Objective-C).
5.5 Select the new OneSignalNotificationServiceExtension
Target, select Build Settings
, then search for Code Signing Entitlements
.
5.6 Delete both Debug
and Release
entries so they are blank.
5.7 In the Project Navigator, select the top-level project directory and select the OneSignalNotificationServiceExtension
target.
Ensure the Deployment Target
is set to the same value as your Main Application Target. Unless you have a specific reason not to, you should set the Deployment Target
to be iOS 10 which is the version of iOS that Apple released Rich Media for push. iOS versions under 10 will not be able to get Rich Media.
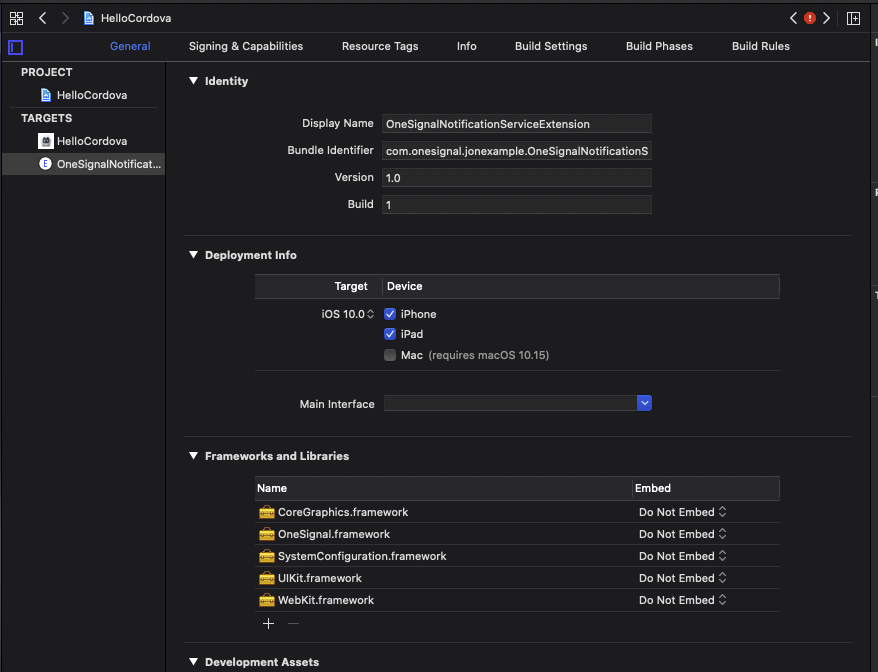
This should be the same value as your Main Application Target.
5.8 Close Xcode and open platform/ios/Podfile
5.9 Add the following to the bottom of the file.
target 'OneSignalNotificationServiceExtension' do
use_frameworks!
pod 'OneSignalXCFramework', '>= 3.0.0', '< 4.0'
end
5.10 Open you terminal to platform/ios
and run pod install
iOS - Add App Groups (Recommended)
In order for your application to use Confirmed Deliveries and increment/decrement Badges through push notifications, you need to setup an App Group for your application.
Please follow the iOS SDK App Groups setup guide to add the OneSignal App Group in your app.
iOS Automatic Builds (Optional)
If you are using an automated build system, you may run into issues where the Push Notification capability is not enabled for your project. In order to resolve this problem, you can follow these steps:
5.11 In your Xcode project, make sure the Push Notifications capability (as well as any other capabilities your app needs).
5.12 Close the Xcode project. In the /platforms/ios
folder you will see a {yourProjectName}.entitlements
file. Copy this file to the root of your Cordova project.
5.13 Edit your config.xml
file to include the following. Make sure to replace [yourProjectName]
with the name of your project.
<platform name="ios">
<resource-file src="[yourProjectName].entitlements" />
</platform>
Step 6. Run Your App and Send Yourself a Notification
Run your app on a physical device to make sure it builds correctly. Note that the iOS Simulator does not support receiving remote push notifications.
- Android and iOS devices should be prompted to subscribe to push notifications if you used the example setup code provided.
Check your OneSignal Dashboard Audience > All Users to see your Device Record.
Then head over to Messages > New Push to Send your first Push Notification from OneSignal.
Troubleshooting
If you run into any issues please see our Cordova Variants troubleshooting guide.
Try the example project on our Github repository.
If stuck, contact support directly or email [email protected] for help.
For faster assistance, please provide:
- Your OneSignal App Id
- Details, logs, and/or screenshots of the issue.
- Steps to reproduce
Step 7. Set Custom User Properties
Recommended
After initialization, OneSignal will automatically collect common user data by default. Use the following methods to set your own custom userIds, emails, phone numbers, and other user-level properties.
Set External User Id
Required if using integrations.
Recommended for messaging across multiple channels (push, email, sms).
OneSignal creates channel-level device records under a unique Id called the player_id
. A single user can have multiple player_id
records based on how many devices, email addresses, and phone numbers they use to interact with your app.
If your app has its own login system to track users, call setExternalUserId
at any time to link all channels to a single user. For more details, see External User Ids.
let externalUserId = '123456789'; // You will supply the external user id to the OneSignal SDK
window.plugins.OneSignal.setExternalUserId(externalUserId);
Set Email and Phone Number
Recommended if using Email and SMS messaging.
Use the provided SDK methods to capture email and phone number when provided. Follow the channel quickstart guides for setup:
// Ionic 5 Capacitor may need to use (window as any).plugins.OneSignal
window.plugins.OneSignal.setEmail("[email protected]");
// Ionic 5 Capacitor may need to use (window as any).plugins.OneSignal
window.plugins.OneSignal.setSMSNumber("+11234567890");
// Pass in email provided by customer
[OneSignal setEmail:@"[email protected]"];
// Pass in phone number provided by customer
[OneSignal setSMSNumber:@"+11234567890"];
Data Tags
Optional
All other event and user properties can be set using Data Tags. Setting this data is required for more complex segmentation and message personalization.
window.plugins.OneSignal.sendTags({key: "value", key2: "value2"});
[OneSignal sendTag:@"key" value:@"value"];
Step 8. Implement a Soft-Prompt In-App Message
Optional
It is recommended that apps create an alert, modal view, or other interface that describes the types of information they want to send and gives people a clear way to opt in or out.
OneSignal provides an easy option for a "soft-prompt" using In-App Messages to meet this recommendation and have a better user experience. This also permits you to ask for permission again in the future, since the native permission prompt can no longer be shown in your app if the user clicks deny.
See our How to Prompt for Push Permissions with an In-App Message Guide for details on implementing this.
Step 9. Add Android Icons (Android Only)
Follow the Android Notification Icons instructions to create small notification icons.
Step 10. Add Amazon ADM Support (Kindle Fire Apps Only)
Place your api_key.txt
file into your <project-dir>/platforms/android/assets/
folder.
To create an api_key.txt
for your app, follow our Generate an Amazon API Key.
Setup Complete!
Visit Mobile Push Tutorials for next steps.
Updated 4 months ago