Xamarin SDK Setup
Instructions for adding the OneSignal Xamarin SDK to your Xamarin app for iOS, Android, and derivatives like Amazon
Step 1. Requirements
- OneSignal Account
- OneSignal App ID, available in Settings > Keys & IDs
iOS Requirements
- iOS 11+ or iPadOS 11+ device (iPhone, iPad, iPod Touch) to test on. Xcode 14+ simulator works running iOS 16+
- mac with Xcode 12+
- p8 Authentication Token or p12 Push Notification Certificate
Warning
Building for iOS using Xamarin.iOS 15.4 or below may cause release build issues. Either upgrade to Xamarin.iOS 15.4+ or change the iOS project's Linker Behavior to 'Don't Link'
Android Requirements
- Android 5.0+ device or emulator with "Google Play Store (Services)" installed
- Set up your Google/Firebase keys in OneSignal
- Project using AndroidX
- Visual Studio 2019 or newer
Amazon & Huawei Requirements
Follow these instructions if your app is distributed on the Amazon AppStore and/or the Huawei AppGallery.
The Xamarin OneSignal SDK works for both Xamarin Forms and Xamarin Single View projects.
Step 2. Add NuGet Package
2.1 In your Solution
window, under your Android/iOS/Shared projects, drop down the app project.
2.2 Right-click the Dependencies
folder and select Manage NuGet packages
2.3 In the NuGet package window, ensure that the Package Source
at the bottom bar of the window is set to nuget.org
2.4 Search for OneSignalSDK.DotNet
under the Browse
tab and select the OneSignalSDK.DotNet
package. Here you can select the version to be added. For pre-releases, ensure you have the Include prereleases
option checked at the bottom tab.
2.5 Click Add Package
to add the OneSignal package to the app
Step 3. Add Code
Xamarin Forms Project
3.1A Add the OneSignal initialization code in the App.xml.cs
file of your Xamarin Forms project as demonstrated below
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.Core;
using OneSignalSDK.DotNet.Core.Debug;
public App() {
InitializeComponent();
// Enable verbose OneSignal logging to debug issues if needed.
OneSignal.Debug.LogLevel = LogLevel.VERBOSE;
// OneSignal Initialization
OneSignal.Initialize("YOUR_ONESIGNAL_APP_ID");
// RequestPermissionAsync will show the notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission
OneSignal.Notifications.RequestPermissionAsync(true);
MainPage = new MainPage();
}
Xamarin Single View App
Android
3.1B For your Android-specific apps, ensure the OneSignalSDK.DotNet
package has been added to the Android app project
3.2B Add the OneSignal initialization code in MainActivity.cs
in the OnCreate method of your Android app project
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.Core;
using OneSignalSDK.DotNet.Core.Debug;
protected override void OnCreate(Bundle savedInstanceState) {
base.OnCreate(savedInstanceState);
//... Leave exisiting code here
// Enable verbose OneSignal logging to debug issues if needed.
OneSignal.Debug.LogLevel = LogLevel.VERBOSE;
// OneSignal Initialization
OneSignal.Initialize("YOUR_ONESIGNAL_APP_ID");
// RequestPermissionAsync will show the notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission
OneSignal.Notifications.RequestPermissionAsync(true);
}
iOS
3.1B For your iOS specific apps, ensure the OneSignalSDK.DotNet
package has been added to the iOS app project
3.2B Add the OneSignal initialization code in AppDelegate.cs
in your FinishedLaunching
method of your iOS app project
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.Core;
using OneSignalSDK.DotNet.Core.Debug;
public override bool FinishedLaunching(UIApplication app, NSDictionary options) {
//... Leave existing code here
// Enable verbose OneSignal logging to debug issues if needed.
OneSignal.Debug.LogLevel = LogLevel.VERBOSE;
// OneSignal Initialization
OneSignal.Initialize("YOUR_ONESIGNAL_APP_ID");
// RequestPermissionAsync will show the notification permission prompt.
// We recommend removing the following code and instead using an In-App Message to prompt for notification permission
OneSignal.Notifications.RequestPermissionAsync(true);
return base.FinishedLaunching(app, options);
}
Step 4. Android - Update the Target Android version
Open your Android project’s options, then under Build > Android Application set your “Target Android version” to at least version “Android 13.0 (API level 33)”.
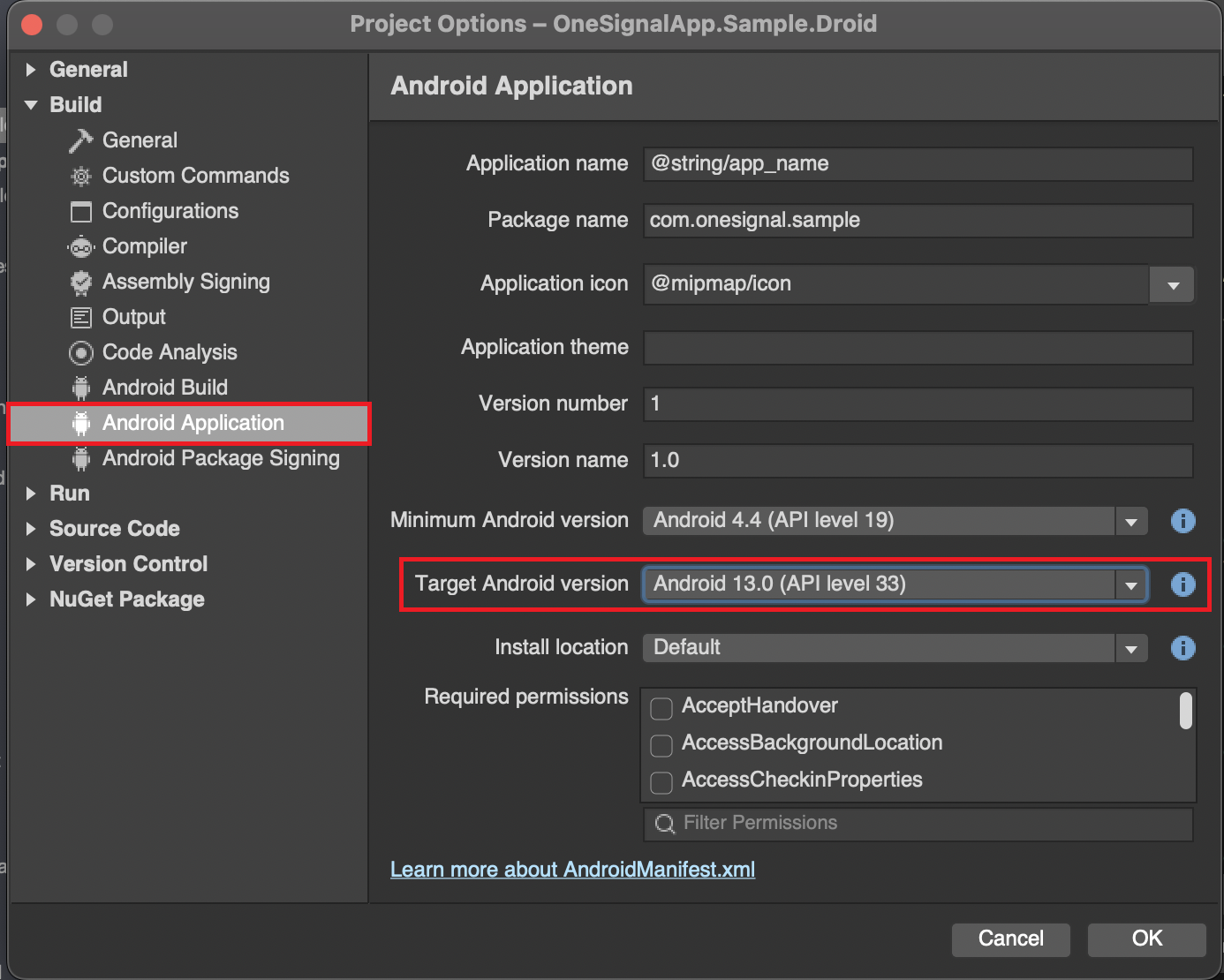
Set your “Target Android version” to at least “Android 13.0 (API level 33)”.
Step 5. iOS - Add the Notification Service Extension
5.1 To display Rich Media such as images and buttons on notifications, use Confirmed Deliveries and increment/decrement Badges through push notifications, you'll first need to set up a Notification Service Extension and App Groups.
5.1 Right-click your project solution and click Add>Add New Project
5.2 Click iOS>Extension>Notification Service Extension and select Next
5.3 Enter the name OneSignalNotificationServiceExtension
and click Next
Important
The Bundle ID for this extension should be the same as the Bundle ID for your main application with
.OneSignalNotificationServiceExtension
added to the end.For example, if your primary application's Bundle ID is
com.example.test
, the Bundle ID for your extension should becom.example.test.OneSignalNotificationServiceExtension
.
5.4 Click Create
to add the Notification Service Extension to your project
5.5 Under the OneSignalNotificationServiceExtension
project, right-click on Manage NuGet Packages
5.6 Search for OneSignalSDK.DotNet
under the Browse
tab and select the OneSignalSDK.DotNet
package. Here you can select the version to be added. For pre-releases, ensure you have the Include prereleases
option checked at the bottom tab.
5.7 Select the NotificationService.cs
source file under your OneSignalNotificationServiceExtension
and replace it with the following
using System;
using Foundation;
using UserNotifications;
using OneSignalSDK.DotNet;
using OneSignalSDK.DotNet.iOS;
namespace OneSignalNotificationServiceExtension
{
[Register("NotificationService")]
public class NotificationService : UNNotificationServiceExtension
{
Action<UNNotificationContent> ContentHandler { get; set; }
UNMutableNotificationContent BestAttemptContent { get; set; }
UNNotificationRequest ReceivedRequest { get; set; }
protected NotificationService(IntPtr handle) : base(handle)
{
// Note: this .ctor should not contain any initialization logic.
}
public override void DidReceiveNotificationRequest(UNNotificationRequest request, Action<UNNotificationContent> contentHandler)
{
ReceivedRequest = request;
ContentHandler = contentHandler;
BestAttemptContent = (UNMutableNotificationContent)request.Content.MutableCopy();
NotificationServiceExtension.DidReceiveNotificationExtensionRequest(request, BestAttemptContent, contentHandler);
}
public override void TimeWillExpire()
{
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
NotificationServiceExtension.ServiceExtensionTimeWillExpireRequest(ReceivedRequest, BestAttemptContent);
ContentHandler(BestAttemptContent);
}
}
}
5.8 Open the OneSignalNotificationServiceExtension
project’s Info.plist
file and make sure the deployment target is at least iOS 10 or higher
5.9 Open the iOS app project’s Entitlements.plist
file and enable App Groups. Please add an app group using your Bundle Identifier - this will be something similar to com.yourcompany.yourapp
. The full name of the app group needs to be in the following format: group.{your_bundle_id}.onesignal
:
5.10 Using the exact same app group name you specified above, repeat the same procedure for your OneSignalNotificationServiceExtension
project, which will have its own Entitlements.plist
file
That's it! Now, your application will be able to display push notification images, action buttons, and more.
Step 6. Run Your App and Send Yourself a Notification
Run your app on a physical device to make sure it builds correctly. Note that the iOS Simulator does not support receiving remote push notifications.
- Android and iOS devices should be prompted to subscribe to push notifications if you used the example setup code provided.
Check your OneSignal Dashboard Audience > All Subscription to see your Device Record.
Then head over to Messages > New Push to Send your first Push Notification from OneSignal.
Please note that Identity Verification is coming soon to v5 of the Xamarin SDK.
Troubleshooting
If you run into any issues please see our Xamarin troubleshooting guide.
Try the example project on our Github repository.
If stuck, contact support directly or email [email protected] for help.
For faster assistance, please provide:
- Your OneSignal App Id
- Details, logs, and/or screenshots of the issue.
- Steps to reproduce
Step 7. Implement a Soft-Prompt In-App Message
Optional
It is recommended that apps create an alert, modal view, or other interface that describes the types of information they want to send and gives people a clear way to opt in or out.
OneSignal provides an easy option for a "soft-prompt" using In-App Messages to meet this recommendation and have a better user experience. This also permits you to ask for permission again in the future, since the native permission prompt can no longer be shown in your app if the user clicks deny.
See our How to Prompt for Push Permissions with an In-App Message Guide for details on implementing this.
Setup Complete!
Visit Mobile Push Tutorials for next steps.
Updated over 1 year ago